Selection Statements in Programming
Selection statements in programming use Boolean expressions to control program flow. Learn about if statements, if-else statements, nested if statements, and logical operators. Explore how to generate random numbers, compute BMI, and work with the boolean data type.
Download Presentation
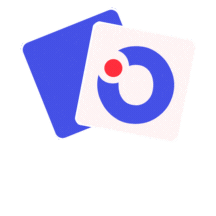
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Selections The statements condition. program can execute decide based which on to a Selection statements use conditions that are Boolean expressions. A Boolean expression is an expression that evaluates to a Boolean value:true or false. Object Oriented Programming 1
Boolean data type The boolean data type declares a variable with the value either true or false (they are literals like number). Ex. boolean lightsOn = true; Object Oriented Programming 2
If (one-way) statement An if statement is a construct that enables a program to specify alternative paths of execution. if (boolean-expression) { statement(s); } Object Oriented Programming 3
Cont. Object Oriented Programming 4
Two-way if else An execution path based on whether the condition is true or false. if (boolean-expression) { statement(s)-for-the-true-case; } else { statement(s)-for-the-false-case; } if-else statement decides the Object Oriented Programming 5
Nested or Multi-way if An if statement can be inside another if statement to form a nested if statement. The if (j > k) statement is nested inside the if (i > k) statement in the following. Object Oriented Programming 6
Generating Random Numbers You can use Math.random() to obtain a random double value between 0.0 and 1.0, excluding 1.0. SubtractionQuiz.java Object Oriented Programming 7
Computing Body Mass Index (BMI) BMI = weigh in KG/ (height in meters)2 ComputeAndInterpretBMI.java Object Oriented Programming 8
Logical Operators The logical operators !, &&, ||, and ^ can be used to create a compound Boolean expression. Object Oriented Programming 9
Cont. Write a program that checks whether a number is divisible by 2 and 3, by 2 or 3, and by 2 or 3 but not both. TestBooleanOperators.java LeapYear.java (A leap year is divisible by 4 but not by 100 or divisible by 400.) Lottery.java Object Oriented Programming 11
Switch statement A switch statement executes statements based on the value of a variable or an expression. switch (switch-expression) { case value1: statement(s)1; break; case value2: statement(s)2; break; case valueN: statement(s)N; break; default: statement(s)-for-default; } The keyword break is optional. The break statement immediately ends the switch statement. The switch statement observes the following rules: The switch-expression must yield a value of char, byte, short, int, or String type and must always be enclosed in parentheses. (The char and String types will be introduced in the next chapter.) The value1, . . ., and valueN must have the same data type as the value of the switchexpression. Note that value1, . . ., and valueN are constant expressions, meaning that they cannot contain variables, such as 1 + x. When the value in a case statement matches the value of the switch-expression, the statements starting from this case are executed until either a break statement or the end of the switch statement is reached. The default case, which is optional, can be used to perform actions when none of the specified cases matches the switch-expression. Object Oriented Programming 12
Conditional Expression A conditional expression evaluates an expression based on a condition. Object Oriented Programming 13
Operator Precedence and Associativity Operator precedence and associativity determine the order in which operators are evaluated Object Oriented Programming 14
Decreasing order of precedence All binary operators except assignment operators are left associative. Object Oriented Programming 15
Mathematical functions They can be categorized as trigonometric methods, exponent methods, and service methods. They all are used like Math.? Object Oriented Programming 16
Cont. Object Oriented Programming 17
Character data type A character data type represents a single character. Computers use binary numbers internally. A character is stored in a computer as a sequence of 0s and 1s. Mapping a character to its binary representation is called encoding. Object Oriented Programming 18
Cont. Java supports Unicode, an encoding scheme established by the Unicode Consortium to support the interchange, processing, and display of written texts. Unicode was originally designed as a 16- bit character encoding. The Unicode standard therefore has been extended to allow up to 1,112,064 characters. Object Oriented Programming 19
Cont. Those characters that go beyond the original 16-bit limit are characters. Java supports the supplementary characters. A 16-bit Unicode takes two bytes, preceded by \u, expressed in four hexadecimal digits that run from \u0000 to \uFFFF. Unicode includes ASCII code, with \u0000 to \u007F corresponding to the 128 ASCII characters. called supplementary Object Oriented Programming 20
Escape Sequence for special characters Java uses a special notation to represent special characters. Object Oriented Programming 21
Casting between character and numeric type A char can be cast into any numeric type, and vice versa. Object Oriented Programming 22
Comparing and Testing Character Two characters can be compared using the relational operators comparing two numbers. This is done by comparing the Unicodes of the two characters just like Object Oriented Programming 23
Cont. Object Oriented Programming 24
String A string is a sequence of characters. To represent a string of characters, use the data type called String. For example; String message = "Welcome to Java"; String is a predefined class in the Java library, just like the classes System and Scanner. The String type is not a primitive type. It is known as a reference type. Object Oriented Programming 25
Cont. Strings are objects in Java. Instance method and static method Object Oriented Programming 26
Reading a string from the console Enter three words separated by spaces: Welcome to Java Object Oriented Programming 27
Reading single character char c = (char) System.in.read(); Object Oriented Programming 28
String Comparison OrderTwoCities.java Object Oriented Programming 29
Obtain substring You can obtain a single character from a string using the charAt method. You can also obtain a substring from a string using the substring method in the String class Object Oriented Programming 30
Finding a Character or a Substring in a String The String class provides several versions of indexOf and lastIndexOf methods to find a character or a substring in a string. Object Oriented Programming 31
Conversion between strings and numbers You can convert a numeric string into a number. To convert a string into an int value, use the Integer.parseInt method, as follows. int intValue = Integer.parseInt(intString); ( 123 ) Double doubleValue Double.parseDouble(doubleString); ( 65.35 ) If the string is not a numeric string, the conversion would cause a runtime error. = Object Oriented Programming 32
Cont. You can convert a number into a string, simply use the string concatenating operator as follows: String s = number + ""; HexDigit2Dec.java Object Oriented Programming 33
Formatting console output You can use the System.out.printf method to display formatted output on the console. Object Oriented Programming 34
Cont. System.out.printf(format, item1, item2, ..., itemk) Object Oriented Programming 35
Cont. Object Oriented Programming 36
Cont. By default, the output is right justified. You can put the minus sign (-) in the format specifier to specify that the item is left justified in the output within the specified field. Object Oriented Programming 37
Loops A loop can be used to tell a program to execute statements repeatedly. While Do while For Object Oriented Programming 38
While SubtractionQuizLoop.java Controlling loop with sentinel value SentinelValue.java Input/output redirection java SentinelValue < input.txt java ClassName > output.txt Object Oriented Programming 39
Dowhile A do-while loop is the same as a while loop except that it executes the loop body first and then checks the loop continuation condition. Object Oriented Programming 40
For loop A for loop has a concise syntax for writing loops. Object Oriented Programming 41
Which loop to use? You can use a for loop, a while loop, or a do-while loop, whichever is convenient. Pre test loop (while and for) Post test loop (do while) Object Oriented Programming 42
Break and Continue The break and continue keywords provide additional controls in a loop. Object Oriented Programming 43
Methods Methods can be used to define reusable code and organize and simplify coding. Object Oriented Programming 44
Defining method A method definition consists of its method name, parameters, return value type, and body. Object Oriented Programming 45
Modularizing Code Modularizing makes the code easy to maintain and debug and enables the code to be reused. By encapsulating the code for obtaining the gcd in a method, this program has several advantages: 1. It isolates the problem for computing the gcd from the rest of the code in the main method. Thus, the logic becomes clear and the program is easier to read. 2. The errors on computing the gcd are confined in the gcd method, which narrows the scope of debugging. 3. The gcd method now can be reused by other programs. Object Oriented Programming 46
Overloading methods Overloading methods enables you to define the methods with the same name as long as their signatures are different. Overloaded methods must have different parameter lists. You cannot overload methods based on different modifiers or return types. TestMethodOverloading.java Object Oriented Programming 47
Cont. Can you invoke the max method with an int value and a double value, such as max(2,2.5)? If so, which of the max methods is invoked? The answer to the first question is yes. The answer to the second question is that the max method for finding the maximum of two double values is invoked. The argument value 2 is automatically converted into a double value and passed to this method. The Java compiler finds the method that best matches a method invocation. Since the method max(int, int) is a better matches for max(3, 4) than max(double, double), max(int, int) is used to invoke max(3, 4). Object Oriented Programming 48
Scope of Variables The scope of a variable is the part of the program where the variable can be referenced. The scope of a method parameter covers the entire method. A variable declared in the initial-action part of a for-loop header has its scope in the entire loop. However, a variable declared inside a for- loop body has its scope limited in the loop body from its declaration to the end o the block that contains the variable Object Oriented Programming 49
You can declare a local variable with the same name in different blocks in a method, but you cannot declare a local variable twice in the same block or in nested blocks Object Oriented Programming 50