Introduction to Programming and Computer Instructions
Programming is the process of creating instructions for computers to follow and accomplish tasks. It involves turning human language instructions into detailed binary machine language. Before learning programming, individuals may have different levels of experience, ranging from no experience to proficient programming skills. Programming languages are tools that humans use to create these instructions, translating high-level code into machine-readable binary code. Understanding the basics of writing instructions for both computers and non-computer tasks is essential in the world of programming.
Uploaded on Sep 08, 2024 | 0 Views
Download Presentation
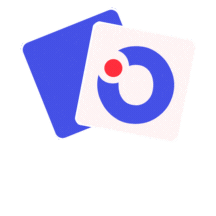
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
CS101 Programming
Programming Programming is creating the instructions the computer will follow to accomplish a task. Augusta Ada King, Countess of Lovelace (10 December 1815 27 November 1852)
iClicker Question Before CS101, what was your experience with computer programming? A) I know how to program. B) I have done some programming but do not know it well. C) I have no programming experience
Congratulations, you are a programmer!
How do we write instructions for non computer tasks? We usually use normal human language in a step by step manner. For example: 1 Turn to your left 2 Take 5 steps 3 Go to whiteboard 4 Pick up pen 5 Write President s name on board Etc. .
What type of instructions do computers need? Very detailed and in binary (also called Machine Language) 0000000001000001100110100001100101100111000111000000111010101 0111010101010101010101000010000101100111110110001011011100100 0000101010100101000100010101011100101000010101111101000000111 1101010101010101011000011010000110010110011100011100000011101 0101011101010101010101010100001000010110011111011000101101110 0100000010101010010100010001010101110010100001010111110100000 0111110101010101010101100001101000011001011001110001110000001 1101010101110101010101010101010000100001011001111101100010110 1110010000001010101001010001000101010111001010000101011111010 0000011111010101010101010110000110100001100101100111000111000 0001110101010111010101010101010101000010000101100111110110001 0110111001000000101010100101000100010101011100101000010101111 1010000001111101010101010101011000011010000110010110011100011 1000000111010101011101010101010101010100001000010110011111011 0001011011100100000010101010010100010001010101110010100001010 11111010000001111101010101010
Program Languages are for humans! 1. We create instructions using a programming language. For example the below Python code for altering the color of a picture : 2. Another program (compiler or interpreter) turns the Python code into the binary code the computer can run: > then> 1010110000110100001100101100111000111 0000001110101010111010101010101010101 0000100001011001111101100010110111001 0000001010101001010001000101010111001 0100001010111110100000011111010101010 1010101100001101000011001011001110001 1100000011101010101110101010101010101 0100001000010110011111011000101101110 0100000010101010010100010001010101110 0101000010101111101000000111110101010 1010101011000011010000110010110011100 0111000000111010101011101010101010101 0101000010000101100111110110001011011 1001000000101010100101000100010101011 1001010000101011111010000001111101010 10101010100100 def makeSunset2(picture): reduceGreen30Percent(picture) reduceBlue30Percent(picture) def reduceGreen30Percent(picture): for p in getPixels(picture): value = getGreen(p) setGreen(p,value*0.7) def reduceBlue30Percent(picture): for p in getPixels(picture): value = getBlue(p) setBlue(p,value*0.7)
To hard to write binary instructions so instead we use programming languages John Backus created the first programming language, FORTRAN, at IBM in 1957 Programmers write instructions for computers using a programming language. After we write the instructions in a program language another program then turns the instructions into the machine language the computers needs. Admiral Grace Hopper
Many Types of Program Languages Low Level Languages Machine Languages (1GL) Assembly Languages (2GL) High Level Languages Procedural Languages (3GL) Task Oriented Languages (4GL) Problem Constraint Languages (5GL) (Someday) Natural Languages Star Trek
Hello World Program Written In A 1st Generation Machine Language 1010110000110100001100101100111000111000000111010101011101 0101010101010101000010000101100111110110001011011100100000 0101010100101000100010101011100101000010101111101000000111 1101010101010101011000011010000110010110011100011100000011 1010101011101010101010101010100001000010110011111011000101 1011100100000010101010010100010001010101110010100001010111 1101000000111110101010101010101100001101000011001011001110 0011100000011101010101110101010101010101010000100001011001 1111011000101101110010000001010101001010001000101010111001 0100001010111110100000011111010101010101010110000110100001 1001011001110001110000001110101010111010101010101010101000 0100001011001111101100010110111001000000101010100101000100 0101010111001010000101011111010000001111101010101010101011 0000110100001100101100111000111000000111010101011101010101
Hello World Program Written In A 2nd Generation Assembly Language .HW: stringz "Hello World" .text .align 16 .global main# .proc main# main: .prologue 14, 32 .save ar.pfs, r33 alloc r33 = ar.pfs, 0, 4, 1, 0 .vframe r34 mov r34 = r12 adds r12 = -16, r12 mov r35 = r1 .save rp, r32 mov r32 = b0 .body addl r14 = @ltoffx(.HW), r1 ;; ld8.mov r14 = [r14], .HW ;; st8 [r34] = r14 ld8 r36 = [r34] br.call.sptk.many b0 = puts# mov r1 = r35 ;;
Hello World Program Written In The 3rd Generation Language C++ // Hello World in C++ (pre-ISO) #include <iostream.h> main() { cout << "Hello World!" << endl; return 0; }
Hello World Program Written In The 5th Generation Language Python print("Hello World")
Someday Star Trek https://www.youtube.com/watch?v=5LgwAD-IioY
Hello World In Other Program Languages http://helloworldcollection.de
How to create a program 1 Program Specification Define the problem 2 Program Design Create an algorithm Can use: Top-Down Design OOP (Object Oriented Design) Logic Structures Flow Charts Etc. Create Pseudocode 3 Program Code 4 Program Test 5 Program Documentation 6 Program Maintenance