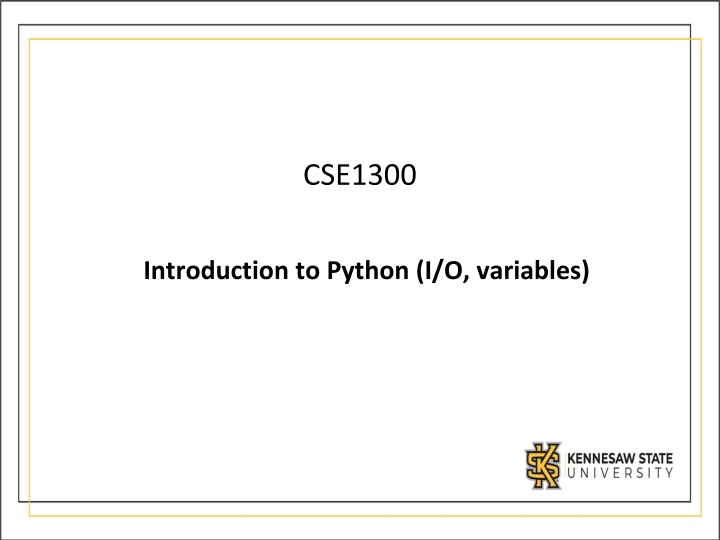
Python Programming: Introduction, Basics, and Setup
"Learn about Python programming language, its features, why you should learn it, setting it up, your first program with 'Hello, World!', running programs, and common print statement variations."
Download Presentation
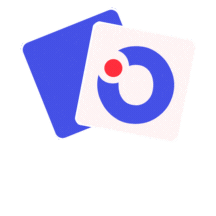
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
CSE1300 Introduction to Python (I/O, variables)
What is Python? High-level Language: Python is a high-level, interpreted programming language created by Guido van Rossum and first released in 1991. It emphasizes code readability, making it a favorite for both beginners and experienced developers. Inspired by the idea that code should be simple, clear, and almost as easy to read as plain English. Python s syntax is designed to reduce complexity fewer symbols and a heavy reliance on indentation. General-Purpose: Widely used in web development, data science, scripting, automation, and more. Community & Ecosystem: Strong community support with numerous libraries and frameworks.
Why Learn Python? Beginner-Friendly: Straightforward syntax helps you focus on logic rather than complex language rules. Job Market Demand: Popular in fields like data science, web dev, and AI, opening many career opportunities. Versatility: Suitable for small scripts, large-scale enterprise solutions, and everything in between.
Setting Up Python You can simply follow the steps from the below video from the FYE website. https://mediaspace.kennesaw.edu/playlist/dedicated/1_zvmtmstx/1 _u2pfx0ja
Your First Python Program The Hello, World! Tradition Printing Hello, World! is a time-honored tradition for learning a new programming language. It demonstrates the basic syntax for outputting text to the screen and confirms that everything is installed and working. print("Hello, world!") Explanation: print() is a built-in function in Python that sends text or other data to the console (standard output). The text "Hello, world!" is a string literal, enclosed in quotes.
Running the Program Interactive Mode (REPL): Type python or python3 in your terminal, then type print("Hello, world!") and press Enter. Script Mode: 1. Open a text editor or IDE. 2. Write the code in a file named hello.py. 3. Run it by typing python hello.py in your terminal.
Common Variations of PRINT statements Using different strings: print("Welcome to Python!") Printing numbers: print(42) OR print("The answer is:", 42) Formatted strings (f-strings): name = "Alice" print(f"Hello, {name}!") Mastering print() is fundamental. Output is often the fastest way to verify logic during development.
Basic Syntax & Indentation Python s syntax is designed to be concise and readable. Unlike many languages that rely on curly braces {} to denote blocks of code, Python uses indentation to determine block structure.
Indentation How It Works: A group of statements that are indented to the same level constitutes a block. if x > 0: print("x is positive") x = x - 1 print("Done!") Typically, 4 spaces per indentation level (instead of tabs) is the Python community standard (PEP 8). Why It Matters: Indentation is not just for style Python will throw an IndentationError if used incorrectly.
Line Endings Python statements usually end when the line ends. No need for semicolons (;) as in some other languages, although you can use them if desired (not recommended for code clarity).
Comments # This is a comment explaining the next line print("Hello, Python!") # inline comment Docstrings: Triple-quoted strings often used at the beginning of modules or functions to describe functionality. """ This module provides a greeting function. """ def greet(name): """Return a greeting for a specific name.""" return f"Hello, {name}" Single-Line Comments: Start a line with #.
Purpose of comments Documenting code improves readability and maintainability. Helps other developers (and your future self) understand the purpose of specific code sections. Other Syntax Conventions Case Sensitivity: Python is case-sensitive (Name vs. name are different variables). Underscores: Often used in variable names (e.g., my_variable) to increase readability. Line Continuation: If a statement is too long, you can use a backslash \ or wrap the expression in parentheses, brackets, or braces to continue on the next line.
Common Errors IndentationError: Occurs when your code s indentation is inconsistent or incorrect. SyntaxError: Typos, missing punctuation, or incorrect use of characters can trigger this. Carefully check your whitespace alignment to ensure logical grouping of statements. Takeaway Proper indentation is essential to structuring programs in Python. Comments help clarify intent, making code more readable and maintainable.
Introduction to I/O Input: Data coming into a program from external sources (keyboard, files, sensors, etc.). Output: Data or information sent from a program to be displayed or stored somewhere (console, file, screen). Role in Programming Enables interaction between the user and the program (e.g., entering names, numbers, commands). Essential for debugging and verifying that your code works as intended.
Console vs. File I/O Console I/O: Reading user input from the command line and printing output to the terminal. File I/O: Storing data in files for later use, or reading data from files instead of direct user input. Importance in Real-World Applications Web Apps: Handling form submissions (input) and displaying web pages (output). Data Processing: Reading data from CSV or text files, generating output reports. Python s Approach Straightforward functions like input() (for input) and print() (for output). Rich standard library with modules (e.g., open() function) for file I/O, networking, etc. Key Takeaway Understanding Python s I/O methods is fundamental to building interactive and practical applications.
Reading Input with input() user_input = input("Enter something: ") print(f"You entered: {user_input}") Pauses the program until the user presses Enter. Returns user input as a string. Prompting the User You can provide text inside input() to guide the user on what to type. Example: input("What is your name? "). Why input() Returns a String Simplifies immediate capturing of user input. You can convert it to other types (int, float) as needed.
Converting Input to Numbers Why Conversion is Needed input() always returns strings. Mathematical operations require numeric types (int or float). Integer Conversion: age_str = input("Enter your age: ") age = int(age_str) print(age * 2) int() tries to convert a string to an integer.
height_str = input("Enter your height in meters: ") height = float(height_str) print(height * 2) Float Conversion: float() attempts to parse a string into a decimal value.
try: Handling Invalid Input: num = int(input("Enter an integer: ")) except ValueError: print("That wasn't an integer!") Use try and except blocks to catch errors gracefully.
Variables An Overview A named placeholder in memory to store a value that can change over time (hence variable ). Purpose Allows retrieval and manipulation of data through a name rather than having to deal with raw memory addresses. Examples score = 100 player_name = "Alice score and player_name hold integer and string data, respectively.
Scope Variables declared in a function are local by default. Global scope variables can be accessed throughout a module (useful but can be dangerous if misused). Case Sensitivity Python treats Score and score as different variables. Maintain consistency in naming to avoid confusion. Why They Matter Fundamental concept in all programming. Keep track of state (e.g., counters, user data, configurations).
Creating and Assigning Variables Single Assignment: age = 20 message = "Hello Creates variables age (int) and message (str). Multiple Assignment: x, y, z = 1, 2, 3 Assign different values to different variables in one line.
Same Value to Multiple Variables: a = b = c = 0 All reference the same value initially. Changing one does not affect the others if the value is immutable, but watch out for mutable objects like lists. Reassignment: count = 5 count = count + 1 # Now count is 6 Variables can change throughout the program s execution.
Naming Conventions snake_case: user_name, total_price. Avoid starting with digits or using special characters (except _). Why This Matters Variables improve readability and maintainability of your code. Proper naming clarifies the role of each piece of data.
Data Types in Python Overview of Common Data Types int: Whole numbers (e.g., 10, -5). float: Decimal or floating-point numbers (e.g., 3.14). str: Strings (e.g., "Hello"). bool: Booleans (True or False). NoneType: Represents the absence of a value (None).
Collections (brief mention) list: Ordered, mutable sequences. tuple: Ordered, immutable sequences. dict: Key-value pairs for fast lookups. set: Unordered collections with unique elements.
Working with Integers and Floats Integer Arithmetic + (add), - (subtract), * (multiply), // (floor division), ** (exponent). Example: 7 // 3 yields 2, ignoring the remainder. Float Arithmetic +, -, *, / (true division). Example: 7 / 3 yields 2.3333...
Precision Issues Floating-point numbers can introduce rounding errors (e.g., 0.1 + 0.2 != 0.3 exactly). Decimal module or round() can help manage precision. Mixing int and float Expressions with both types result in floats. Example: 1 + 2.0 3.0 Type Conversions float(5) 5.0 int(5.9) 5 (truncation, not rounding) Practical Applications Counting items, indexing lists (int). Dealing with measurements, currency calculations (float, but watch out for precision).
String Variables in Detail String Creation Single quotes 'hello' or double quotes "hello". Triple quotes """long string""" for multi-line strings or docstrings. Concatenation & Repetition "Hello " + "World" "Hello World" "Hi" * 3 "HiHiHi" Indexing s = "Python" print(s[0]) # 'P' print(s[-1]) # n Zero-based indexing: first character at index 0, last at -1.
s = "Python" print(s[1:4]) # 'yth' print(s[:2]) # 'Py' print(s[2:]) # 'thon Allows extracting substrings. Slicing
Boolean Variables and Comparisons Definition Boolean variables hold either True or False. Comparison Operators == (equal to), != (not equal to), >, <, >=, <=. Example: 5 > 3 True. Boolean Expressions Combine comparisons with logical operators: and, or, not. Example: (x > 0) and (x < 10) True if x is between 1 and 9.
Practical Use Cases Checking conditions (e.g., user login success or failure). Loop control, branching logic in programs. Short-Circuiting and and or might not evaluate the second operand if the first one decides the outcome. Example: if x != 0 and (10 / x) > 2: if x is 0, (10 / x) isn t even evaluated, avoiding error. Truthy & Falsy Values Many Python objects can be used in a Boolean context. 0, "" (empty string), [] (empty list) are considered False. Everything else is True.
Dynamic Typing in Python What is Dynamic Typing? Variables are not locked to a single data type. They can change over time. Example x = 10 # x is an int x = "Ten" # now x is a str Advantages Faster to develop prototypes. Flexible with changing requirements. Disadvantages Potential for type-related errors that the interpreter won t catch until runtime. Harder to maintain large projects without careful type checking or documentation.
Putting It All Together (Sample Code) # Simple calculator to add two numbers num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) total = num1 + num2 print(f"The sum of {num1} and {num2} is {total}")
Explanation Line 1: A comment describing the purpose. Lines 2-3: Reading input from the user, converting strings to floats. Line 4: Adding the two numbers. Line 5: Displaying a formatted string with the result. What We ve Used input() for user input. float() to convert from string to float. Variables to store data. print() to show the result.
Potential Enhancements Add error handling with try/except. Allow the user to choose different operations (+, , , ). Practical Value Demonstrates the core basics of Python: variables, I/O, and arithmetic. Exercise Extend this code to handle subtraction, multiplication, and division. Validate user input to catch invalid entries.
Common Errors and Troubleshooting SyntaxError Occurs when Python can t parse code. Example: Missing colon in a function definition. IndentationError Happens when indentation is inconsistent. Example: Mixing tabs and spaces in one code block. NameError Using a variable or function that hasn t been defined. Example: print(scroe) instead of score.
TypeError Invalid operation on a data type (e.g., subtracting a string from an integer). Example: "Hello" - 2. ValueError Problem with converting data from one type to another. Example: int("abc"). How to Fix Them Check Code Carefully: Look for typos and indentation mistakes. Print Debugging: Print variable values to isolate problems. Google or Documentation: Many solutions are documented online.
Simple Debugging Tips Print Statements Print variables at key points to verify values. Keep track of progress in loops or conditionals. Comment Out Sections Temporarily remove code you suspect might be causing issues. Helps isolate the problem area. Use an IDE/Debugger PyCharm, VS Code, or other IDEs have built-in debugging tools. Step through your code line by line, inspect variable values.
Rubber Duck Debugging Explain your code to a rubber duck (or a colleague), often helps you find logic errors. Limit Scope Write small, modular functions. Test each part individually before combining them. Read Error Messages Carefully Python s error messages typically point you to the exact line and type of error.