Python Coding Examples for Beginners
Explore various Python coding examples including calculating Fibonacci numbers, manipulating arrays, implementing Euclid's algorithm, finding prime numbers, and solving list difference problems. The examples cover a range of fundamental Python concepts and are accompanied by explanations to help beginners understand and practice coding in Python effectively.
Download Presentation
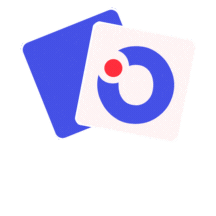
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
BAZI RNEK PYTHON KODLARI
split from index k and add first part of arr to the end # Calculates the first n Fibonacci numbers n = 100 # Keep track of the two most recent Fibonacci numbers a, b = 1, 1 print(a, b, end='') for i in range(2, n+1): # The next number (b) is a+b, and a becomes the previous b a, b = b, a+b print(' ', b, end='') def splitArr(arr, n, k): for i in range(n-1, k-1, -1): x = arr[n-1] for j in range(n-1, 0, -1): arr[j] = arr[j - 1] arr[0] = x # main arr = [12, 10, 5, 6, 52, 36] n = len(arr) position = 2 splitArr(arr, n, position) for i in range(0, n): print(arr[i], end = ' ')
Euclid's algorithm for finding the gcd of a number >>> a, b = 1071, 462 >>> while b: ... a, b = b, a % b >>> print(a) def SieveOfEratosthenes(n): # Create a boolean array "prime[0..n]" and initialize all entries it as true. A value in prime[i] will finally be false if i is Not a prime, else true. prime = [True for i in range(n+1)] p = 2 while (p * p <= n): # If prime[p] is not changed, then it is a prime if (prime[p] == True): # Update all multiples of p for i in range(p * 2, n+1, p): prime[i] = False p += 1 # Print all prime numbers for p in range(2, n): if prime[p]: print(p) print(SieveOfEratosthenes(50))
SAMPLE EXAM QUESTONS A a daki python fonksiyonu iki listeyi arg man olarak al p aralar ndaki farkl elemanlar n listesini geri d nd recektir. Eksik kalan sat r yaz n z. def Diff(li1, li2): li_dif=[] for i in li1+li2: A a daki python kodu ekrana ne basar? def func(li, n): li2=[] for i in range(len(li)): if(li[i]==n): li2.append(i) return li2 test_list = [1, 3, 4, 3, 6, 7, 9, 3, 2, 5] print ("Result is : " + str(func(test_list, 3))) if i not in li1 or i not in li2: li_dif.append(i) return li_dif Result is : [1, 3, 7] basar.
def spam() : eggs = 'spam' print(eggs) flip_flop = True increment = 0 number = 6 while number > 1 : if flip_flop == True : increment = 2 flip_flop = False else : increment = -3 flip_flop = True number = number + increment print(number) def bacon() : eggs = 'bacon' print(eggs) spam() print(eggs) eggs = 'global' bacon() print(eggs)
def spam(number) : if (number[0] % 2) == 0 : number[0] = number[0] // 4 print(number[0]) def spam(number) : if (number % 2) == 0 : number = number // 4 print(number) def bacon(number) : if (number[0] % 2) != 0 : number[0] = 5 * number[0] + 1 print(number[0]) def bacon(number) : if (number % 2) != 0 : number = 5 * number + 1 print(number) number = [30] while number[0] > 1 : bacon(number) spam(number) number = 30 while number > 1 : bacon(number) spam(number)
def isPalindrome(s): return s == s[::-1] # Driver code s = "malayalam" ans = isPalindrome(s) if ans: print("Yes") else: print("No")
>>> scores = [87, 75, 75, 50, 32, 32] >>> ranks = [] >>> for score in scores: ... ranks.append(scores.index(score) + 1) ... >>> ranks [1, 2, 2, 4, 5, 5]
def removeDuplicate(str): s=set(str) s="".join(s) print("Without Order:",s) t="" for i in str: if(i in t): pass else: t=t+i print("With Order:",t) str="geeksforgeeks" removeDuplicate(str)
def uncommon(A, B): un_comm = [i for i in B.split() if i not in A.split()] return un_comm #Driver code A = "Geeks for Geeks" B = "Learning from Geeks for Geeks" print(uncommon(A, B))
# initializing string test_str = 'Geeksforgeeks is best for geeks and CS' # printing original string print("The original string is : " + str(test_str)) # initializing word list word_list = ["best", 'CS', 'for'] # initializing replace word repl_wrd = 'gfg' # Replace multiple words with K # Using join() + split() + list comprehension res = ' '.join([repl_wrd if idx in word_list else idx for idx in test_str.split()]) # printing result print("String after multiple replace : " + str(res))
def checkEmpty(input, pattern): # If both are empty if len(input)== 0 and len(pattern)== 0: return 'true' # If only pattern is empty if len(pattern)== 0: return 'true' while (len(input) != 0): # find sub-string in main string index = input.find(pattern) # check if sub-string founded or not if (index ==(-1)): return 'false' # slice input string in two parts and concatenate input = input[0:index] + input[index + len(pattern):] return 'true' input ='GEEGEEKSKS' pattern ='GEEKS' print (checkEmpty(input, pattern))
# To find minimum sum of product of number def findMinSum(num): sum = 0 # Find factors of number and add to the sum i = 2 while(i * i <= num): while(num % i == 0): sum += i num /= i i += 1 sum += num # Return sum of numbers having minimum product return sum # Driver Code num = 12 print findMinSum(num)
def hailstone(n): count = 1 """Print the terms of the 'hailstone sequence' from n to 1.""" assert n > 0 print(n) if n > 1: if n % 2 == 0: count += hailstone(n / 2) else: count += hailstone((n * 3) + 1) return count result = hailstone(10) print(result)
#Function to get gcd of a and b def gcd(a, b): if b == 0: return a; else: return gcd(b, a%b) # Driver program to test above functions arr = [1, 2, 3, 4, 5, 6] leftRotate(arr, 2, 6) print(arr, end=" ") #Function to left rotate arr[] of size n by d def leftRotate(arr, d, n): for i in range(gcd(d,n)): # move i-th values of blocks temp = arr[i] j = i while 1: k = j + d if k >= n: k = k - n if k == i: break arr[j] = arr[k] j = k arr[j] = temp
# Function to do insertion sort def insertionSort(arr): # Traverse through 1 to len(arr) for i in range(1, len(arr)): key = arr[i] # Move elements of arr[0..i-1], that are greater than key, to one position ahead of their current position j = i-1 while j >= 0 and key < arr[j] : arr[j + 1] = arr[j] j -= 1 arr[j + 1] = key # Driver code to test above arr = [12, 11, 13, 5, 6] insertionSort(arr) for i in range(len(arr)): print ("% d" % arr[i])
# Program to get list of specified number of consecutive elements def grouping(nums): list=[] count=1 for i in range(1, len(nums)): if nums[i] != nums[i-1]: list.append((nums[i-1], count)) count=1 else: count+=1 return list def get_list(nums, n): result = [a[0] for a in grouping(nums) if a[1] == n] return result nums1 = [1, 1, 3, 4, 4, 5, 6, 5, 6, 7] n = 2 print(get_list(nums1, n))
#function to find the difference between consecutive numbers in a given list def diff_consecutive_nums(nums): result = [b-a for a, b in zip(nums[:-1], nums[1:])] return result #function to reverse each list in a given list of lists def reverse_list_lists(nums): nums=[i[::-1] for i in nums] return nums nums = [[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12], [13, 14, 15, 16]] print(reverse_list_lists(nums)) #program to create a list whose ithelement is the maximum of the first i elements from a input list def test(nums): return [max(nums[:i]) for i in range(1, len(nums) + 1)] nums = [0, -1, 3, 8, 5, 9, 8, 14, 2, 4, 3, -10, 10, 17, 41, 22, -4, -4, -15, 0] print(test(nums))