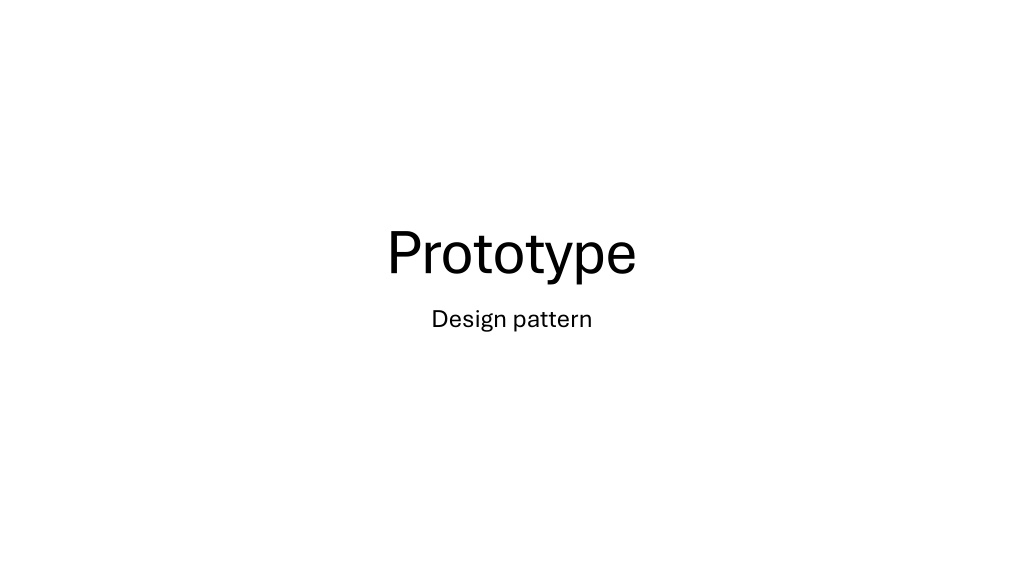
Implementing Prototype Design Pattern in Java
Learn how to implement the Prototype design pattern in Java to create precise object copies, allowing clients to instantiate objects without directly accessing private properties. Understand the concept of decoupling and the benefits it offers in object creation and manipulation.
Download Presentation
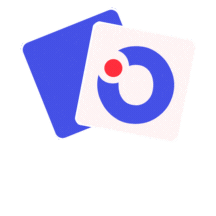
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Prototype Design pattern
vod -Pklad z relnho svta Prototypy produktu D len bun k
Pro Vytvo en p esn kopie objektu Jak ud lat? Vytvo it objekt od stejn t dy Nakop rovat stejn hodnoty do vlastnost Car A = new Car(); A.maxSpeedKmH = 300; A.Brand= Buggati ; A.Color= Color.Red; Car B = new Car(); //kopie B.maxSpeedKmH = A.MaxSpeedKmH; B.Brand= A.Brand; B.Color= A.Color;
Problm Nemus me zn t t du Jeho vlastnosti mohou b t private
een Tvo iv (creational) n vrhov vzor Interface prototype Schopnost prototypu vytv et p esnou kopii - funkce clone ()
Co jsme tm zskali? P stup k priv tn m vlastnostem Mo nost klienta vytv et objekty Decoupling
Interface public class Car implements Prototype { private string brand; private Color color; private int maxSpeedKmH; Public interface Prototype { Car clone(); } Public Car(Car car){ this.maxSpeedKmH = car.maxSpeedKmH; this.Brand = car.brand; this.Color = car.color; } Public Car clone() { return new Car(this); } }
Abstract class public class Car : Vehicle { private int maxSpeedKmH; public abstract class Vehicle { private String brand; private Color color; public Car(Car car):base(){ this.maxSpeedKmH = car.maxSpeedKmH; } public abstract Vehicle clone(); public Car clone(){ return new Car(this); } private Vehicle(Vehicle vehicle){ this.brand= vehicle.brand; this.color= vehicle.color; } } } public class Bus : Vehicle { private int doors; public Bus clone() { return new Bus(this); }
Rozdl public void listClone(List<Vehicle> vehicles){ List<Vehicle> copyList= new List<>(); for(Vehicle vehicle: vehicles){ if(vehicle instanceofCar) { //P idat kopii auta } else if (vehicle instanceof Bus){ //P idat kopii autobusu } } public void listClone(List<Vehicle> vehicles) { List<Vehicle> copyList = new List<>(); for(Vehicle vehicle: vehicles){ copyList.Add(vehicle.clone()); } }
Shallow vs Deep copy Mezi kop rovan mi vlastnostmi je reference na n jak objekt
Shallow copy Stejn reference na objekt v pam ti Jak koli zm na u obou public class Car : Vehicle { private int maxSpeedKmH; private GpsSystem gpsSystem; public Car(Car car):base(){ this.maxSpeedKmH = car.maxSpeedKmH; this.gpsSystem = car.gpsSystem; } public Car clone(){ return new Car(this); } }
Deep copy Zm na neovlivn druh a naopak Vytvo me nov objekt public class Car : Vehicle { private int maxSpeedKmH; private GpsSystem gpsSystem; public Car(Car car):base(){ this.maxSpeedKmH = car.maxSpeedKmH; this.gpsSystem = new GPsSytem(); // or gpsSystem.clone() } public Car clone(){ return new Car(this); } }
public class VehicleChache { Prototype registry private Map<String, Vehicle> cache = new HashMap<>(); public VehicleCache() { Car car = new Car(); car.brand = "Bugatti"; car.model = "Chiron"; car.topSpeed = 261; Bus bus = new Bus(); car.brand = "Mercedes"; car.model = "Setra"; car.topSpeed = 48; Katalog prototyp Hash mapa Vytvo en prototyp P stupn klientovi cache.put("Bugatti Chiron", car); cache.put("Mercedes Setra", bus); public Vehicle get(String key){ return cache.get(key).clone(); } } }
Factory vs prototype Factory Nen mo n kopie Prototype Overhead
Kdy pout Mnoho r zn ch t d Obt n konstrukce Dynamick vytv en
Nevhody Obt n implementovat clone() 1. Nekop rovateln objekty 2. Cyklick reference Moc r zn instance