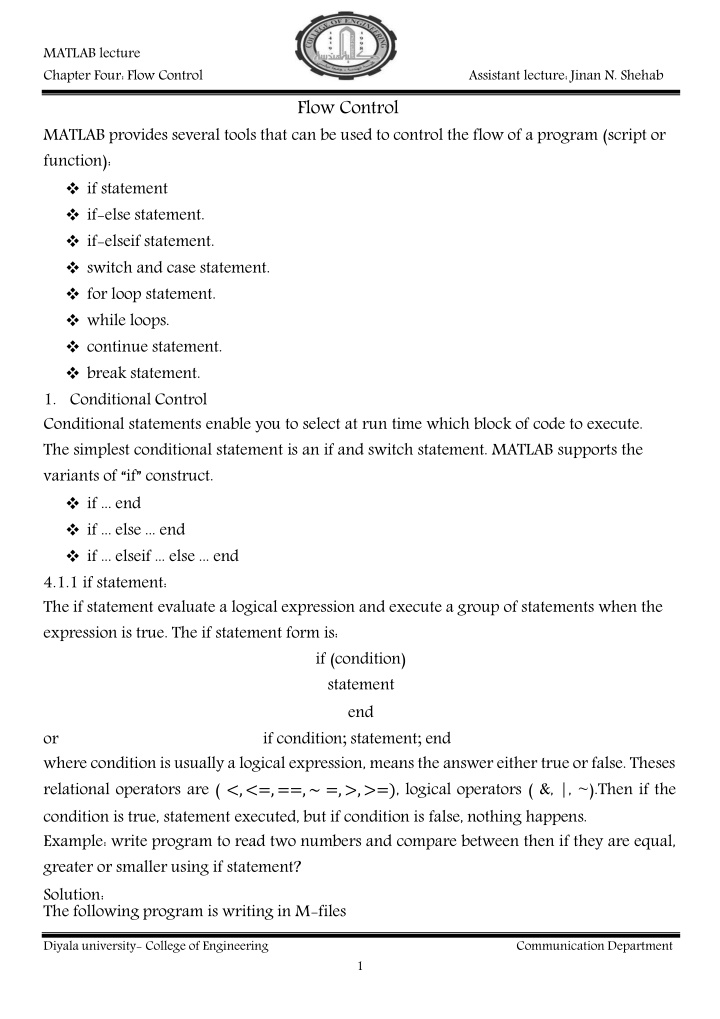
Flow Control in MATLAB Programming
MATLAB provides tools for controlling the flow of a program through conditional statements like if, if-else, if-elseif, switch-case, loops, and controlling statements like continue and break. Learn about how to use these constructs effectively in your MATLAB programs.
Download Presentation
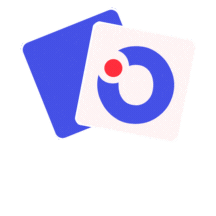
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
MATLAB lecture Chapter Four: Flow Control Assistant lecture: Jinan N. Shehab Flow Control MATLAB provides several tools that can be used to control the flow of a program (script or function): if statement if-else statement. if-elseif statement. switch and case statement. for loop statement. while loops. continue statement. break statement. 1. Conditional Control Conditional statements enable you to select at run time which block of code to execute. The simplest conditional statement is an if and switch statement. MATLAB supports the variants of if construct. if ... end if ... else ... end if ... elseif ... else ... end 4.1.1 if statement: The if statement evaluate a logical expression and execute a group of statements when the expression is true. The if statement form is: if (condition) statement end or where condition is usually a logical expression, means the answer either true or false. Theses relational operators are ( <,<=,==,~ =,>,>=), logical operators ( &, |, ~).Then if the condition is true, statement executed, but if condition is false, nothing happens. Example: write program to read two numbers and compare between then if they are equal, greater or smaller using if statement? Solution: The following program is writing in M-files if condition; statement; end Diyala university- College of Engineering Communication Department 1
MATLAB lecture Chapter Four: Flow Control close all clear all num1=input('enter number one'); num2=input('enter number two'); if num1>num2, disp('number one is greater'); end if num2> num1; disp('number two is greater');end if num1==num2 disp('number one is equal to number two') end Assistant lecture: Jinan N. Shehab Example:- the general quadratic equation x1, x2= the term (b2-4ac) is equal to zero and a is not equal to zero? Solution:- close all clear all a= input(' enter the value of a'); b= input(' enter the value of b'); c= input(' enter the value of c'); if (b^2-4*a*c==0)&(a~=0) disp('this quadratic equation has equal roots') x=-b/(2*a) end 4.1.2 if-else statement: Theif-elsestatementisusing tochoose exactly oneoutoftwostatements (possiblycompound statements) to be executed; specifies the conditions under which the first statement is to be executed and provides an alternative statement to execute if these conditions are not met. The basic form of if-else statement for use in a program file is: if (condition) statement 1; else statement 2; end has an equal roots (x1= x2) if or Diyala university- College of Engineering if condition statement 1 else statement 2 end Communication Department 2
MATLAB lecture Chapter Four: Flow Control Example:- repeat above example Solution:- close all clear all a= input(' enter the value of a'); b= input(' enter the value of b'); c= input(' enter the value of c'); if (b^2-4*a*c==0)&(a~=0) disp('this quadratic equation has equal roots') x=-b/(2*a) else disp('this quadratic equation did not have equal roots') end 4.1.3 if- elseif statements: In most programming languages, choosing from multiple options means using nested if-else statements. However, MATLAB has another method of accomplishing this, using the elseif clause. The Efficient Method to choose from among more than two actions, the elseif clause is used. For example, if there are n choices (where n > 3 in this example), the following general form would be used: If condition 1 statement 1 elseif condition 2 statement 2 elseif condition n statement n end Example:- write program to implementing the following continuous mathematical function y = f(x): y = 1 for x < 1 y = x2 for 1 x 2 y = 4 for x > 2 Solution: Assistant lecture: Jinan N. Shehab Diyala university- College of Engineering Communication Department 3
MATLAB lecture Chapter Four: Flow Control close all clear all x= input(' enter the value of x'); if x < -1 y = 1 elseif x >= -2 && x <= 2 y = x^2 elseif x>2 y = 4 end 4.1.4 switch and case statements: A switch statement can often be used in place of a nested if-else or an if statement with many elseif clauses. Switch statements are used when an expression is tested to see whether it is equal to one of several possible values. The general form of a switch statement is: switch variable case case-number1 statement 1 case case-number 2 statement 2 case case-number N statement N otherwise statemen t end so the statement following the case statement is executed when the case number match the variable value entry with the switch statement. Example:- write M-file to define a (3 3) matrix A with elements from 1 to 9 then find the requirement below depending on your entry from 1 to 4 : 1- The transpose of matrix A. 2- The determine of matrix A. 3- The inverse of matrix A. 4- The sum of the main diagonal of matrix A. Assistant lecture: Jinan N. Shehab Diyala university- College of Engineering Communication Department 4
MATLAB lecture Chapter Four: Flow Control Solution:- close all clear all A=[1:3;4:6;7:9] n= input(' Enter your choice from 1 to 4: ') switch n case 1 disp(' The transpose of matrix A is : ') A' case 2 disp(' The determinate of matrix A is : ') det(A) case 3 disp(' The inverse of matrix A is : ') inv(A) case 4 disp(' The sum of main diagonal of matrix A is : ') trace(A) otherwise disp(' Wrong number, plz enter another number ') end 4.2 for- loop The for statement, or the for loop, is used when it is necessary to repeat statement(s) in a script or function, and when it is known ahead of time how many times the statements will be repeated. The general form of for statement is: for variable= x: s: y statement, ., statement end x= represent the beginning , y= the end time, s= the step of the loop, if not want s then the step size =1. Example:- find the factorial of the any input number (n!)? Solution: Assistant lecture: Jinan N. Shehab Diyala university- College of Engineering Communication Department 5
MATLAB lecture Chapter Four: Flow Control close all clear all n=input(' Enter any number: ') f=1; for i=1:1:n f=f*i; end disp(' The factorial of this number is: ') f 4.2.1 nested for Loops The action of a loop can be any valid statement(s). When the action of a loop is another loop, this is called a nested loop. Example:- write program to print a triangle of * s instead of a box? For example, * * * * * * Solution:- close all clear all row = 3; for i=1:row for j=1:i fprintf('*') end fprintf('\n') end 4.3 while loop: The while loop executes a statement or group of statements repeatedly as long as the controlling expression (logical condition) is true. The general form of a while statement is: statement Assistant lecture: Jinan N. Shehab while expression end Diyala university- College of Engineering Communication Department 6
MATLAB lecture Chapter Four: Flow Control So the statements are executed while the real part of the expression has all non-zero elements. The expression is usually the result of a logical expressions( ==, <,>, <=, >= or ~=) Example: - find the factorial of the any input number (n!) by using while statement? Solution:- close all clear all n=input('enter any number: '); f=1; i=1; while(i<=n) f=f*i; i=i+1; end disp(' The factorial of this number is: ') f 4.4 continue statement The continue statement passes control to the next iteration of the for or while loop in which it appears, skipping any remaining statements in the body of the loop. In nested loops, continue passes control to the next iteration of the for or while loop enclosing it. Example:- write a script file to print the even elements in matrix A. where A = 23 11 12 34 [ 42 56 2 9] 77 82 52 21 12 10 33 2 Solution:- close all clear all A=[23 11 12 34;42 56 2 9;77 82 52 21;12 10 33 2]; for i=1:4 for j=1:4 if rem(A(i,j),2)~=0 continue end disp(A(i,j)) Assistant lecture: Jinan N. Shehab Diyala university- College of Engineering Communication Department 7
MATLAB lecture Chapter Four: Flow Control end end 4.5 break loop The break statement terminates the execution of a for loop or while loop. When a break statement is encountered, execution continues with the next statement outside of the loop. In nested loops, exits from the innermost statement is encountered, execution continues with the next statement outside of the loop. In nested loops, break exits from the innermost loop only. Example:-writeM-fileto findasolutionforthe exponentialseriesbelow,??= + ?+?+ Make the output precision be 0.0001 (the program willstopped when the value of ! ?? Reached to 0.0001). Solution:- close all clear all x=input('Enter the value of x: ') n=input(' Enter the value of n: ') s=0; for i=1:n f=1; for j=1:i f=f*j; end f1=f; e=x^i/f1; if e<0.0001 break end s=s+e; end disp(' the result is: ') s Assistant lecture: Jinan N. Shehab ! ? + ?? ?! ?! Diyala university- College of Engineering Communication Department 8