Essential Guide to F# Programming
Dive into Functional Programming with F# - Learn the key concepts, syntax, and objectives to kickstart your journey. Explore the differences between imperative and functional programming, grasp the basic functional concepts, and understand the overview of F#. Discover the power of F# as a general-purpose language for various applications, from games to web services. Get started with F# today!
Download Presentation
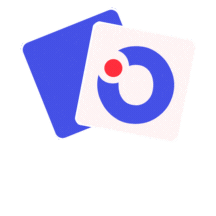
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Busy Developer's Guide to F#
Objectives Get started with F# Learn how FP is different from O-O Learn how F# provides O-O Get some syntax under your belt Realize that this is just the start of a journey
What's it mean, exactly? FUNCTIONAL PROGRAMMING
Concepts What's wrong with imperative statements? dependences on mutable state compiler out-of-order rewrites difficulties reasoning about the code concurrency planning/programming
Concepts Functional languages functional as in mathematics' notion of function for every x, there is a corresponding value y this implies no side effects not imperative statements, but expressions x = x+1 is not increment... it's impossible this implies expressions can be substituted ... or executed independently (parallellism) spectrum of "functional-ness", known as
Concepts Some basic functional concepts immutable values over mutable variables functions as first-class values currying, partial-application of functions expressions-not-statements laziness/deferred execution Some optional functional concepts strongly-typed, type-inferenced recursion
From a high level, what is this thing called 'F#'? F# OVERVIEW
Overview What is F#? General-purpose Object-oriented Functional Hybrid Running on the .NET platform With full Visual Studio integration
Overview General-purpose Meaning, use it for anything! Games WSDL or REST services Web applications Math/science calculations
Overview Object-oriented Object = Union of state + data Class-based Interfaces Implementation inheritance Method overriding
Overview Functional Functions are first-class values Partial application Function composition Currying Immutable state Lack of side effects
Overview Hybrid Objects + Functions = BETTER Use OO when it makes sense Use FP when it makes sense When neither makes sense, just wing it
Overview .NET Platform Full CLI Producer and Consumer Runs on the CLR Garbage-collection Compiles to IL, JIT-optimized Full access to the .NET platform and libraries Full access to the .NET ecosystem (NuGet, etc) Produce assemblies for consumption by C#/VB/etc
Overview Visual Studio Integration Comes out of the box in VS2010+ VS debugger, profiler, etc Color syntax highlighting, IntelliSense, ... F# Project types FSI (F# Interactive)
Overview Some example F# code let message = "Hello, world! (From F#)" System.Console.WriteLine(message) for i = 1 to 10 do System.Console.WriteLine(message + " " + i.ToString())
Overview Elements of note F# is a type-inferenced language type-qualifiers can always be appended if desired/necessary F# has full access to the .NET platform F# basic types are CLR basic types (mostly) int, string, float, ... are all CLR types Comments are (* *) pairs or // lines /// are XML comments Semicolon line terminators unnecessary
Overview Three modes of execution compile (Visual Studio or fsc.exe) files are compiled in order; this is important "one-pass"/"no-lookahead" rules script (fsi.exe) file needs an .fsx extension in this case REPL (Visual Studio FSI or fsi.exe) in Visual Studio, Ctrl-Enter is your friend here
Basics Syntax indentation-sensitive (* *) and // comment syntax /// XML comments Unicode character set Identifiers are [a-z][A-Z][_]0-9 acceptable
Basics let let establishes a name/value binding Once bound, value cannot be changed type is often inferred provide a type annotation to identifier when desired or necessary
Basics let let x = 5 let y = 4.7 let message = "Now is the time for all good men" let multilineMessage = "Now is the time for all good men to take up the cause of their country."
Basics Types byte, sbyte, int16, uint16, int, int32, uint, uint32, int64, uint64 float, float32, decimal bigint (System.BigInteger) string char Bounded Types bool: true, false unit: ()
Basics () : unit null is evil
Basics Arithmetic operators +, -, , /, %,* (exponent Boolean operators && (and), || (or), not (not)
Basics Comparison operators <, <= less than , >= greater than = equality <> inequality
Basics Functions Also just "let" bindings functions aren't the same as methods more on this later final expression provides return value
Basics More let let add l r = l + r let leetSpeak (phrase : string) = phrase. Replace('e', '3'). Replace('o', '0') let leet = leetSpeak "leet" // l33t let tedTest test = test "Ted" let isMe me = if me = "Ted" then "It is Ted!" else "Dunno who you are" tedTest isMe
Basics Control flow if/then/elif/else for (comprehensions)
Basics More let let isOdd n = if (n / 2) = (n % 2) then true else false for i = 1 to 10 do System.Console.WriteLine(i) let evens n = [ for i in 0..n do if i % 2 = 0 then yield i ] let evenSingles = evens 9 // 0, 2, 4, 6, 8
Basics Pattern matching Control flow construct Also a destructuring construct Also a type matching construct And a dessert and floor topping
Basics Pattern matching <</home/runner/work/Slides/Slides/Content/FSharp/basics.fs NOT FOUND>>
Basics Lists bracketed syntax arrays use a different syntax, and are not preferred cons operator (::) range (..) generates range of values Lists used a lot for recursive operations
Basics Lists let list1 = [ ] // empty list let list2 = [1; 2; 3] // list of 1, 2, 3 let list3 = 0 :: list2 // list of 0, 1, 2, 3 let range = [1 .. 100] // list of 1, 2, ..., 99, 100 // Common to use for comprehensions to build a list let evens n = [ for i in 0 .. n do if i % 2 = 0 then yield i ] let evenSingles = evens 9 // 0, 2, 4, 6, 8 // Lists have a number of functions; more on this later
Basics Lists let rec factorial n = if n = 0 then 1 else n * factorial (n-1) let rec sumList xs = match xs with | [] -> 0 | y::ys -> y + sumList ys
Basics Sequences Lazy-evaluated range of values Similar in some ways to a list with the exception that the sequence values aren't in memory (yet) think IEnumerable
Basics Lists let allPositiveIntegers = seq { for i in 1 .. System.Int32.MaxValue do yield i } // fits in memory let alphabet = seq { for c in 'A' .. 'Z' -> c } let abc = Seq.take 3 alphabet // seq ['A';'B';'C'] // Prove it! let noisyAlphabet = seq { for c in 'A' .. 'Z' do printfn "Yielding %c" c yield c } let fifthLetter = Seq.nth 4 noisyAlphabet // 'E'
Basics Tuples unnamed grouping of data values concept: DTOs are tuples commonly used across lots of functional languages "strucutrally-equivalent" type systems can be "destructured" through let binding NOTE: difference between multiple args and one tuple arg is subtle, but very real
Basics Tuples let speaker = ("Ted", "Neward", "iTrellis") // speaker is string * string * string let city = ("Seattle", "Washington", 5000000) // city is string * string * int let person = ("Charlotte", "Neward", 39) // person is string * string * int let firstName, lastName, age = person // firstName = "Charlotte" // lastName = "Neward" // age = 39 let add l r = l + r // add is int -> int -> int let Add (l : int, r : int) = l + r // Add is (int * int) -> int
Basics Tuples let people = [ ("Charlotte", "Neward", 39); ("Ted", "Neward", 42); ("Fred", "Flintstone", 51); ("Katy", "Perry", 21) ] let personsAge p ln = match p with | (_, ln, age) -> age let ages = [ for p in people do yield personsAge p "Neward" ]
Basics Option replacement for null only two possible values: Some(T) or None think of this as a "collection of 1 or 0" but used to help isolate against no responses TIP: where you used to use null, use an option instead
Basics Discriminated Unions A type that can only be one of several possible values Each value referred to as a union case Fully type-checked Vaguely similar to enumerated types but with more structure Can also include other value types as part of the value
Basics Discriminated Unions type Suit = | Heart | Diamond | Spade | Club type PlayingCard = | Ace of Suit | King of Suit | Queen of Suit | Jack of Suit | PointCard of int * Suit let deckOfCards = [ for suit in [Spade; Club; Heart; Diamond] do yield Ace(suit) yield King(suit) yield Queen(suit) yield Jack(suit) for value in 2 .. 10 do yield PointCard(value, suit) ]
Basics Discriminated Unions and Pattern matching let describeCards cards = match cards with | cards when List.length cards > 2 -> failwith "Too few cards" | [Ace(_); Ace(_)] -> "Pocket Rockets" | [King(_); King(_)] -> "Cowboys" | [PointCard(2, _); PointCard(2, _)] -> "Ducks" | [PointCard(x, _); PointCard(y, _)] when x = y -> "Pair | [first; second] -> "You got nuttin"
Basics Modules similar to namespaces, but permits top-level functions more functional style tends towards "module.function" style of invocation Namespaces .NET namespace, with all the same restrictions can only contain classes, interfaces, etc Both are "open"ed to use
Objects Records Sort of a "proto-object" Named fields (unlike tuples) Built-in behaviors (unlike objects) Add properties, methods as desired "Clone" records to new records
Objects Records open System type PersonRecord = { FirstName : string; LastName: string; Age : int} member this.FullName = FirstName + " " + LastName member this.Greet(other : string) = String.Format("{0} greets {1}", this.FirstName, other) let ted = { FirstName = "Ted"; LastName = "Neward"; Age = 42 } Console.WriteLine("{0} {1} is {2}", ted.FirstName, ted.LastName, ted.Age) // Cloning records: use 'with' let michael = { ted with FirstName="Michael"; Age=20 }
Objects Classes Constructors "primary" constructor appears on type declaration "secondary" constructors defer to primary Fields fields are implied from constructor parameters can also be explicitly declared if desired, but... implicitly private, implicitly in scope, implicitly immutable
Objects Classes: constructors, fields type Person(firstName : string, lastName : string, age : int) = member p.FirstName = firstName member p.LastName = lastName member p.Age = age
Objects Classes Properties Properties are members (use the keyword) Properties are declared using "self" syntax Properties are implicitly read-only Methods Methods are members (use the keyword) Methods accept a body Method return is the final expression in the body