A Primer on Dart Programming Language and Flutter
Flutter, a UI toolkit by Google, uses Dart language for app development. Dart is an object-oriented language supporting features like interfaces, mixins, and static typing. This article provides an overview of Dart syntax, variables, data types, and more, essential for understanding Flutter.
Download Presentation
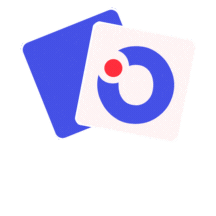
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Flutter The language Dart a primer
Dart Google had its first ever release of Flutter 1.0 , December 2018. Flutter 2.0 March 2021, Flutter 3.0 May 2022. Dart is the programming language used to code Flutter apps. Dart looks a bit like C and is an object-oriented programming language. And a lot of javascript "like" pieces as well. Dart is both a compiled language and a scripting language. It transcompiles to JavaScript for use as web front end. Dart is an object-oriented, class defined, garbage-collected language[9] using a C-style syntax that transcompiles optionally into JavaScript. It supports interfaces, mixins, abstract classes, reified generics, static typing, and a sound type system. https://en.m.wikipedia.org/wiki/Dart_(programming_language)
Dart, a primer This is not expected to be a complete guide to Dart. there are references a the end. This should get the basics and at most of the Dart language used in the examples. Dart is some what of tricky language, because it's changed over the last couple of years, adding some complexities to tutorials and guides the nullablity like used in kotlin is a recent addition for example.
Hello word, the Dart version file: main.dart void main() { print ('Hello, Dart Word!'); } dart main.dart Note, you can also use DartPad to test and run Dart commands and language. but you have to install dart in order to use flutter, so it exists on your command line.
public vs private. there are no keywords public and private if a variable (or class?) starts with a _ then it private, otherwise it's public int _myvar =1; //private int myvar2 =2; //public
Variables types Numbers Integer (64-bit signed) and Double (64-bit double precision). int x = 35; double y = 36.6; Strings no distinction between characters and strings. just strings. uses UTF-16 codes for representation. uses either ' ' or " ", with special case for """ """ that is litterals and allows for multi line. string var = "hello world"; OR string var = 'hello world'; string var2 = """hello world""";
variable types (2) bool bool myBool = true; uses the two keywords true and false. you can't use integers like c++ List and Map list is an ordered group of objects map is a set of values as a key value pairs. dynamic, the scripting language dart can have typeless variables (called dynamic), but these are not allowed flutters version of dart. final and const make a variable immutable. final is after it initialized during runtime, while const is during compile time. final today = DateTime.now(); const daysInYear = 365;
null and nullabity. null is very similar to kotlin's syntax int ? var; //this variable can be null. int var2 = var!; ! is a null assertion that it will not be null here. But there is a better way late int var; //we will initialize this variable, before it used. int var2 = var; //now the compiler knows there will be a value.
null and nullabity. (2) the ? says that it can be null. only strings can be null and some return values. String ? dairy if (dairy != null) { } But null is also uninitialized, so int ? var; is perfect legal as well. list?.isEmpty says that this list? could be uninitialized and we don't want the code the blow because isEmpty is no there. String ? notAstring = null; print (notAstring?.length); //outputs null and ignores the length variable. but print (notAstring!.length); //the asset fails and the program stops with an exception.
null and nullabity. (3) testing for null. ?? is short cut if statement. if null return the value that follows value = name ?? 'guest'; trinary version value = name != null ? name : 'guest'; long version if (name != null) { value = name } else { value = 'guest'; }
list list are pretty much arrays, but are closer to java collections then actual c++ array. var list = <int>[]; //empty list of integers. var mylist = [0,1,10]; print(mylist); //output [0,1,10] you have access to add, addAll, and remove methods on the list mylist.add(100); mylist.remove(10); print(mylist); //output [0,1,100] there is a sort, insert position, shuffle methods. https://api.dart.dev/stable/2.19.4/dart-core/List-class.html if you want a fixed length array, like c++ int arr[5]; final fixedarr = List<int>.filled(5,0); //5 positions, 0 to 4, with a value of 0. final prevents add/remove methods.
maps maps have a key value association. think of python lists or any hashing. var gifts = { // Key: Value 'first': 'partridge', 'second': 'turtledoves', 'fifth': 'golden rings' }; gifts['first'] = 'partridges'; //changes first gifts['third'] = 'French hens'; //adds third
flow control if, if else, if else if, switch case, for, while, and do .. while loops exist as you would expect. switch (variable_experssion) { case constant_expr1: statement(s); break; //not optional case constant_expr2: statement(s); break; case epr3: //empty clause fall throw is allowed. case exp4: statement(s); break; default: statement(s); //default is optional } if ( boolean_expression) { statement(s); } else if (Boolean) trinary operator as well var visibility = isPublic ? 'public' : 'private';
flow control (2) loops while(boolean_expression) { for(var initialize; boolean_expression; increment) { statement(s); } using a comma, you can join multiple statements together in the for ( ) itself prints factorial for(var temp, i = 0, j = 1; j<30; temp = i, i = j, j = i + temp) { print('${j}'); } } do { statement(s); } while( boolean_expression)
break and continue work very much like c++ does break; exit out of the inner most loop. continue; //go back to the top of the loop, start with iteration.
flow control (3) foreach. But it's part of the lists, iterates over the list (can not change the list value) List daysOfWeek = ['Sunday', 'Monday', 'Tuesday']; daysOfWeek.forEach((print)); daysOfWeek.forEach( (day) { print("day is $day");}); daysOfWeek.forEach((day) => print ("day is $day") );
flow control (3) map function, which allow you to return a new list of values List ls1 = ['Sunday', 'Mondy', 'Tuesday']; var ls2 = ls1.map( (day) { if (day == "Mondy") day= "Monday"; return day; } var ls2.forEach( (print)); //Monday is changed. var ls1.forEach( (print) ); //Mondy remains.
functions functions are as simple as c++ functions, but with a scripted language twist. basic function returnvalue Name(type parameter, type parameter) { //return value is option, as are the parameters return value; } example bool isGreater(int value) { return value > 12; } bool isGreater(int value) => value > 12; //if a one line return value, the short version.
functions (2) You can also used named parameters instead of ordered parameters void myFlags({bool? bold, bool? hidden}) { } myFlags(hidden: false, bold: myvar); defaults values, also so you don't have use all the parameters void myFlags(bool? bold, [bool hidden=false]) { } //[] is optional an sets a default value. myFlags(true); //sets only bold. hidden gets the default value. note flutter widget constructors use named parameters, so you don't need to know the ordering.
Cascades notation When object has a series of methods/values you want to set at the same time. very much like java does it objects uses the .. or ?.. if there is null can be an issue var paint = Paint() ..color = Colors.black ..strokeCap = StrokeCap.round ..strokeWidth = 5.0;
classes it's pretty standard class definition. class <name> { //variables <name>() { }; //default constructor <name>(parameters) { }; //other constructors. //methods } constructors can use initializing lists like c++.
classes (2) you inherent from classes using the extends syntax like java extends or using implements. class baseclass { }; class nextclass extends baseclass { } like standard variables, class variables can be ? (null) if the constructor sets the value, then better to declare it late instead. super. to call base class methods. @override to override a method.
classes and abstract and implements abstract key in front the class. abstract class baseclass2 { void functionname(); //abstract method } class nextclass2 implements baseclass2{ void functionname() { }; } multiple class can be implemented using the ,. implements class1, class2, class3 {
class and generics generic same as java. called a template in c++ abstract class Cache<T> { T getByKey(String key); void setByKey(String key, T value); } Cache<String> name;
References https://dart.dev/ https://dart.dev/guides/language/language-tour https://www.tutorialspoint.com/dart_programming/index.htm Flutter & Dart Cookbook, Rose, O'reilly, Dec 2022
QA &