Understand Broadcast Receivers in Android Development
Discover the role of broadcast receivers in Android development as components that allow registration for system or application events. Learn how to register receivers, receive intents, and utilize popular system broadcasts for efficient app functionality. Explore static and dynamic registration methods, intent handling, and event triggering mechanisms.
Download Presentation
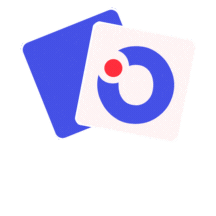
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Cosc 5/4730 Broadcast Receiver
Broadcast receiver A broadcast receiver (short receiver) is an Android component which allows you to register for system or application events. All registered receivers for an event are notified by the Android runtime once this event happens. Basically a listener, but for broadcast information. Such as sms and other broadcast data. Including our own broadcast intents. Note, you don t have access to most screen widgets in a broadcast receiver, except toast.
Uses You app registers which events it wants to receive Your receiver will then receive an intent when the event happens. Boot_completed is popular to start a background service on a reboot. Note the app has to be launched once before this works, but it can be many reboots ago. Text messages custom messages like in the notifications lecture.
System Broadcasts Popular list, but this is not the complete list. Event Description Boot completed. Requires the android.permission.RECEIVE_BOOT_COMPLETED permission. Intent.ACTION_BOOT_COMPLETED Intent.ACTION_POWER_CONNECTED Power got connected to the device. Intent.ACTION_POWER_DISCONNECTED Power got disconnected to the device. Triggered on low battery. Typically used to reduce activities in your app which consume power. Intent.ACTION_BATTERY_LOW Intent.ACTION_BATTERY_OKAY Battery status good again.
The Basics You can register a receiver in the (statically) AndroidManifest.xml or using (dynamically) the context.registerReceiver() method When an intent is received, then the onReceive() method is called. A receiver then does it s work and finishes. In API11+, you can call goAsync() and then the receiver can stay alive until PendingResult.finish() is called.
Declaration. public class MyReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { //now do something with the intent. } } Intent Tells you what it is, via the get getAction() method.
Registering a receiver Statically in the AndroidManifest.xml <application > <activity > </activity> <receiver android:name= myReceiver" > <intent-filter> <action android:name="android.intent.action.BATTERY_LOW" > </action> </intent-filter> </receiver> </application> This is the intent you want the receiver to receive
Registering a receiver (2) Normally in onResume() and onPause() If you forget to unregister, then you will get a leaked broadcast receiver error on exit. You can register for local events or system wide. Local means only your application is going to send intents to it and very likely this custom intents.
Dynamic Local broadcast @Override public void onResume() { super.onResume(); // Register mReceiver to receive messages. LocalBroadcastManager.getInstance(this).registerReceiver(mReceiver, new IntentFilter("CUSTOM_EVENT")); } @Override protected void onPause() { //or onDestory() // Unregister since the activity is not visible LocalBroadcastManager.getInstance(this).unregisterReceiver(mReceiver); super.onPause(); } Example: Where mReceiver is a variable of type BroadcastReceiver(). Must use LocalBroadcastManager.getInstance(getContext()).sendBroadcast(i); in order to Receive if they are registered local.
Send a broadcast Easy to do: Intent i = new Intent("SOME_ACTION"); Global sendBroadcast(i); getActivity().sendBroadcast(i); Local must use LocalBroadcastManager.getInstance(getContext()).sendBroadcast(i); Remember, you can add more data/information to the intent as we have done in many places. NOTE: you can not send system broadcast, like boot_completed.
Dynamic system Wide broadcast Where mReceiver is a variable of type BroadcastReceiver(). @Override public void onResume() { super.onResume(); // Register mReceiver to receive messages. getBaseContext().registerReceiver(mReceiver, new IntentFilter(Intent.ACTION_BATTERY_LOW)); } @Override protected void onPause() { //or onDestory() getBaseContext()unregisterReceiver(mReceiver); super.onPause(); }
Now what? So now your application can respond to an event. Like launch an activity Start a service Or just doing something quickly in the receiver. Remember an intent can contain data in the bundle so an intent you create, send via a pendingIntent through say alarm or notification service. Others system events maybe have information as well.
System Broadcasts. A common one is for an application to start a service when the devices finishes it boot. IE Run on startup and continue to run. These services are common for polling for notifications and sort of thing. Example: SnapChat (and many apps), It running in the background as a service polling every so often their services for new messages . Setups a notification when there are new messages. Should be noted, this a drain on the battery.
Battery and Charging. You app may want to know when the battery state changes. You can be notified when the battery state and charging state changes. Using a receiver and intents listed before. Note, for immediate info, see the following: http://developer.android.com/training/monitoring-device- state/battery-monitoring.html
Screen on/off There is a broadcast to any running applications for the Screen On or Off Intent.ACTION_SCREEN_OFF and Intent.ACTION_SCREEN_ON Your activity or service must be running Or the system will ignore your application. This is an odd one because when the screen turns off , your activity likely just received an onPause() call too.
API 26+ Static register broadcast receivers (androidmanifest.xml) Most implicit broads no longer work (almost many services too) Only the following are except: https://developer.android.com/guide/components/broadcast-exceptions.html Note action_boot_completed is on the list, but not the ac or battery. All dynamic register broadcast receivers work. Implicit and explicit broadcasts. Implicit is a system wide broadcast, which is what most of the previous slides show. Most of these no longer work. Explicit broadcasts are directed at an application only. These all work.
Explicit broadcasts We need the action and the package for explicit So the code now looks like this (and works all API 9+) Intent i = new Intent(MainActivity.ACTION1); i.setPackage("edu.cs4730.broadcastdemo1"); //explicit getActivity().sendBroadcast(i);
Demo code BroadCastDemo1 Simple implementation of a receiver with a static and dynamic registered intent-filter. The dynamic one is also a localbroadcast example. BroadCastDemo2 Setup to receive intents about battery status and power status. Uses dynamic only registrations BroadcastNoti A reimplementation of the notification demo but using only receivers for the broadcast. BroadcastBoot Receives a broadcast on boot, that starts a service. Note, you need to start the main activity once, and to see it really work, reboot the emulator.
References http://www.vogella.com/tutorials/AndroidBroadcastReceiver/a rticle.html http://developer.android.com/reference/android/content/Broa dcastReceiver.html http://www.tutorialspoint.com/android/android_broadcast_re ceivers.htm
QA &