Working with FrameNet in Python
Examples provided demonstrate how to access FrameNet data in Python using functions like frames(), frame(), lus(), and lu(). You can search for specific frames, get details of individual frames, lexical units, and roles in FrameNet. The content showcases how to retrieve information on medical frames, lexical units like "a little bit", and detailed information on a specific lexical unit.
Download Presentation
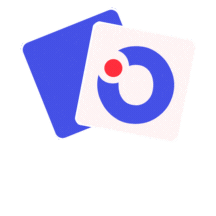
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
FrameNet in Python: Examples To get a list of all of the Frames in FrameNet, use frames() function and provide a regular expression pattern to the frames() function. Output will be a list of all Frames whose names match that pattern: >>> from pprint import pprint >>> from nltk.corpus import framenet as fn >>> len(fn.frames()) 1019 >>> pprint(fn.frames(r'(?i)medical')) [<frame ID=256 name=Medical_specialties>, <frame ID=257 name=Medical_instruments>, ...]
FrameNet in Python: Examples To get the details of a particular Frame, you can use the frame() function passing in the frame number: >>> from pprint import pprint >>> from nltk.corpus import framenet as fn >>> f = fn.frame(256) >>> f.name 'Medical_specialties' >>> f.definition # doctest: +ELLIPSIS "This frame includes words that name ..." >>> pprint(sorted([x for x in f.FE])) ['Affliction', 'Body_system', 'Specialty', 'Type'] >>> pprint(f.frameRelations) [<Parent=Cure -- Using -> Child=Medical_specialties>]
FrameNet in Python: Examples Details for a specific lexical unit can be obtained using this class's lus() function, which takes an optional regular expression pattern that will be matched against the name of the lexical unit: >>> from pprint import pprint >>> from nltk.corpus import framenet as fn >>> len(fn.lus()) 11829 >>> pprint(fn.lus(r'(?i)a little')) [<lu ID=14744 name=a little bit.adv>, <lu ID=14733 name=a little.n>, ...]
FrameNet in Python: Examples To obtain detailed information on a particular LU by calling the lu() function and passing in an LU's 'ID' number: >>> from pprint import pprint >>> from nltk.corpus import framenet as fn >>> fn.lu(256).name 'foresee.v' >>> fn.lu(256).definition 'COD: be aware of beforehand; predict.' >>> fn.lu(256).frame.name 'Expectation' >>> fn.lu(256).lexemes[0].name 'foresee'
Roles in FrameNet There are two types of roles in FrameNet Core Roles and Non Core roles Core Roles Attribute Scalar property of ITEM Difference Distance by which ITEM changes its position on the scale Final State A description that present the item state after the change in attribute value as an independent prediction. Final Value Position on the scale where item ends up Initial State A description that present the item state before the change in attribute value as an independent prediction. Initial Value Initial position of item. Item The entity that has position on the scale. Value range A portion of scale.
Roles in FrameNet There are two types of roles in FrameNet Core Roles and Non Core roles Non Core Roles Duration Time over which changes takes place in value Speed Rate of change of value Group The group in which item changes the value
FrameNet Examples: With Roles Oil rose in price by 2% Item=Oil, in price= Attribute 2%=Difference Microsoft share fell to 750 Item=Microsoft share, Final value=750 A steady increase from 10 to 20 percent in dividend Item=Dividend, initial-value=10, final-value=20
Wordnet-Python >>> from nltk.corpus import wordnet as wn >>> wn.synsets('bass') [Synset('bass.n.01'), Synset('bass.n.02'), Synset('bass.n.03'), Synset('sea_bass .n.01'), Synset('freshwater_bass.n.01'), Synset('bass.n.06'), Synset('bass.n.07' ), Synset('bass.n.08'), Synset('bass.s.01')] >>> wn.synsets('program') [Synset('plan.n.01'), Synset('program.n.02'), Synset('broadcast.n.02'), Synset(' platform.n.02'), Synset('program.n.05'), Synset('course_of_study.n.01'), Synset( 'program.n.07'), Synset('program.n.08'), Synset('program.v.01'), Synset('program .v.02')] >>> syns=wn.synsets('program') >>> print(syns[0].name()) plan.n.01 >>> print(syns[0].definition()) a series of steps to be carried out or goals to be accomplished
Wordnet-Python >>> syns=wn.synsets('bank') >>> print(syns[0].name()) bank.n.01 >>> print(syns[0].definition()) sloping land (especially the slope beside a body of water) >>> wn.synsets('bank') [Synset('bank.n.01'), Synset('depository_financial_institution.n.01'), Synset('b ank.n.03'), Synset('bank.n.04'), Synset('bank.n.05'), Synset('bank.n.06'), Synse t('bank.n.07'), Synset('savings_bank.n.02'), Synset('bank.n.09'), Synset('bank.n .10'), Synset('bank.v.01'), Synset('bank.v.02'), Synset('bank.v.03'), Synset('ba nk.v.04'), Synset('bank.v.05'), Synset('deposit.v.02'), Synset('bank.v.07'), Syn set('trust.v.01')]
Wordnet-Python >>> print(syns[1].definition()) a financial institution that accepts deposits and channels the money into lending activities >>> print(syns[1].examples()) ['he cashed a check at the bank', 'that bank holds the mortgage on my home'] >>> print(syns[0].examples()) ['they pulled the canoe up on the bank', 'he sat on the bank of the river and wa tched the currents'] >>>