Understanding Algorithms and Python in Computer Science
Introduction to algorithms and problem-solving in computer science, highlighting the importance of definitions and characteristics of algorithms. Exploring the significance of Python as a high-level interpreted language and its role in modern programming. Discussing high-level languages, their impact on productivity, and the need for translation into machine language for execution.
Download Presentation
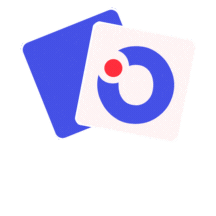
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
COSC 1306 COMPUTER SCIENCE AND PROGRAMMING Jehan-Fran ois P ris jfparis@uh.edu Fall 2017
THE ONLINE BOOK CHAPTER I GENERAL INTRODUCTION
Problem solving Given a problem, find a solution Should be Correct Accurate Complete We want an algorithm
Why? Once we have an algorithmic solution for a problem, we can convert it into a program Let a computer solves many instances of the problem Algorithms existed before computers existed Euclid s algorithm, Computers made them more important
What defines an algorithm Must always produce the right result Under all circumstances Instructions should be well-defined Anybody using the algorithm should obtain the same answer Should have a finite number of steps Cannot run forever
These are not algorithms On a shampoo bottle: Lather Rinse Repeat
These are not algorithms On a shampoo bottle: Lather Rinse Repeat How many times?
These are not algorithms On fuel tank cap: Turn until three o'clock
These are not algorithms On fuel tank cap: Turn until three o'clock Ambiguous!
Python High-level interpreted language Created by Guido Van Rossum Named after Monty Python's Flying Circus BBC comedy series of early 70 s
High-level languages Have replaced assembler Increase programmer s productivity Can run on multiple architectures Intel/AMD and ARM Must be translated into machine language before being executed Two approaches Compiled languages Interpreted languages
Compiled languages Programs written in old Fortran, C, C++ and so go through a program that translates them into directly executable code The compiler Doing g++ myprogram.cpp o myprogram produces an executable file called myprogram that can run anytime No need to recompile
C program Once C compiler Executable Every time Computer Results
Advantages The executable can run on any computer with Same CPU instruction set Same or compatible operating system We can sell copies of the executable without revealing the secrets of our program Reverse engineering is possible but very time-consuming
Interpreted languages Languages like Python and Ruby are not compiled Translated again and again into bytecode each time we execute them Bytecode is interpreted by the language interpreter
Source code Python Interpreter: translates and executes the program Every time Results
Advantages Platform independence: Bytecode can run on any platform for which there is an interpreter Dynamic typing: Same variable can refer to a string then an integer then Smaller executable sizes
Disadvantages Portability limitations : Bytecode will not run on any machine on which the interpreter was not installed. Speed: Bytecode is not optimized for a specific architecture Just-in-time compilation introduces delays Cannot sell copies of a program without revealing its source code
A partial solution In many cases, speed is not an issue outside of loops than get executed thousand and thousands of times Loops inside other loops Can rewrite code for these inner loops in C and include this code into a Python program Use Python C API
Neither fish nor fowl Java is compiled Like C into bytecode Like Python Bytecode is executed by Java Virtual Machine
A comparison Compiled languages Translate once the whole program into an executable Interpreted languages Translate programs line by line each time they are executed Much slower Can run on any machine having the interpreter installed Fast Can run on any machine having same architecture and same OS
Programs Specify a sequence of instructions to be executed by a computer The order of the instructions specify their order of execution
Example Informal sales_tax = total_taxable tax_rate with tax_rate = 0.0825 Python taxRate = 0.0825 salesTax = totalTaxable*taxRate
My first Python program print("I add two numbers, 2 and 3") print(2 + 3) It s print and not Print Cannot use smart quotes Semicolons are optional and nobody uses them
Types of instructions Input & output name = input("Enter your name: ") print("Hello! ") Math and logic celsius = (fahrenheit - 32)*5/9 Conditional execution if celsius > 100 : Repetition for all lines in myfile:
Debugging Finding what s wrong in your program Finding the problem Hardest Fixing the error
Types of errors Syntax errors print("Hello!) # missing closing quotes print("Hello!") # correct A bigannoyance Especially for beginners Everyone ends better at it
Types of errors Run-time errors/Exceptions Division by zero A forgotten special case
Types of errors Semantic errors Your solution is wrong You expressed it poorly taxRate = 8.25 # in percent! salesTax = totalTaxable*taxRate
Experimental debugging (I) Modify your program until it works?
Experimental debugging (II) Look at your program Try to explain it to yourself, to friends Do not let them copy your code! Add print statements after each step Remove them or better comment them out when they are not needed anymore.
Experimental debugging (III) Act as if you are investigating a mystery
Natural and formal languages Natural languages are complex, tolerate ambiguities, often have to be taken figuratively I am literally dying of thirst Could you bring me a glass of water? Formal languages are simpler, have much more strict syntax rules, and want to be unambiguous What they say will be taken literally
Natural language ambiguities KIDS MAKE NUTRITIOUS SNACKS STOLEN PAINTING FOUND BY TREE QUEEN MARY HAVING BOTTOM SCRAPED MILK DRINKERS ARE TURNING TO POWDER SQUAD HELPS DOG BITE VICTIM
My first program print("I add two numbers, 2 and 3") print(2 + 3) It s print and not Print Cannot use smart quotes Semicolons are optional and nobody uses them
Interactive Python Requires Javascript to be authorized for at least interactivepython.org
Commenting Main purpose To help human readers understand your programs Becomes more important as program sizes grow To record who wrote the program, when it happened and so on
In-line comments Anything following a pound sign ( # ) is ignored by the Python interpreter print ("Hello World!") print ("Hello World!") # what to say? r = d/2 # compute radius of circle #Begin main loop
Multi-line comments Anything between three double quotes """ is ignored by the Python interpreter """ Tell what the program does Jehan-Francois Paris Assignment # 1 COSC 1306 MW 2:30-4:00 Fall 2017 """ At the beginning of each program