Introduction to Python Variables and Expressions
Today we start Python! Learn two ways to use Python: writing programs in files and testing simple commands in the interpreter. Understand how variables work in Python, allowing you to store and change values easily. Dive into different kinds of variables like numbers, booleans, and strings. Explore the rules for naming variables and when to use them. Discover how Python can output the contents of variables for easier understanding and debugging.
Download Presentation
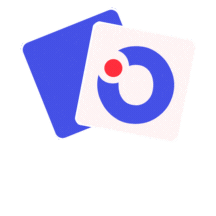
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Variables and Expressions CMSC 201
Today we start Python! Two ways to use python: You can write a program, as a series of instructions in a file, and then execute it. You can also test simple python commands in the python interpreter.
Variables Last class we talked about variables being places with names that store information. In python, you can make a variable like this: someVariable = 10 This creates a variable, called someVariable, that has the value of 10. This value can change later on. The equals sign is called the assignement operator. It allows you to change the value of a variable.
Variables The right hand side of the assignment can be any mathematical expression. For example! someVariable = 10 anotherVariable= 5 + 9 aThirdVariable= 10 * anotherVariable someVariable = someVariable + anotherVariable Whenever a variable is on the right hand side of an expression, imagine it being replaced by whatever value is currently stored in that variable.
Variables There are many different kinds of variables! Numbers (Integers or decimals) True / False values (called booleans) Strings (collections of characters) aString = Hello everyone decimal = 1.12 bool = True
Rules for Naming Variables We have certain rules about what a variable can be named: Variable names are case sensitive. A variable named Hello and a variable named hello are regarded as different. Only may contain alphabetic letters, underscores or numbers. Should not start with a number. Cannot be any other python keyword (if, while, def, etc). You should always attempt to give your variables meaningful names!
When do I make variables? Variables are designed for storing information. Going back to our averaging example from earlier, when we were summing up the list we needed a place to put that sum as it was being generated. Any piece of information you wish your program to use or record must be stored in a variable. Think of it as giving that piece of information a name.
Output We d like to see what s stored in our variables! Python can print things for us: print( Hello world ) You can also output the contents of variables: someVariable = 10 print(someVariable) You can even do combinations! print( Your variable is , someVariable)
Exercise What will the following code snippet print? a = 10 b = a * 5 c = Your result is: print(c, b)
Exercise What will the following code snippet print? a = 10 b = a a = 3 print(b) There are two possible options for what this could do! Any guesses?
Exercise a = 10 b = a a = 3 print(b) It will print out 10. When you set one variable equal to another, they don t become linked; b is set to 10 and no longer has anything else to do with a.
Input Sometimes, we d like the user to participate! We can also get input from the user. userInput = input( Please enter a number ) print(userInput) The output will look like this: Please enter a number 10 10
Input This line: userInput = input( Please enter a number ) Takes whatever the user entered and stores it in the variable named userInput You can do this as many times as you like! userInput1 = input( Please enter your first number. ) userInput2 = input( Please enter your second number. )
Input Note: All input will come as a string, a series of characters. There is a difference between 10 and the number 10. 10 is composed of two characters stuck together, while python understands 10 as a number. To turn an input into a number, you can do the following: someNumber = input( Please enter a number: ) someNumber = int(someNumber) int stands for integer
Exercise Write, on paper or on your computer, a program that asks the user for two numbers a prints out the average.
Exercise Pretend you re writing a program to compute someone s weight grade. You have so far: hwWeight = .4 examWeight = .5 discussionWeight = .1 Write a program starting with these three lines that asks the user for their homework grade, exam grades, and discussion grades and prints out their total grade in the class.