Introduction to File Processing in Python
Python offers various functions for handling files, allowing users to create, read, update, and delete files. By using the open() function, files can be created or existing ones opened with different modes like reading, writing, or appending. Understanding file handling is essential for storing data persistently across program runs.
Download Presentation
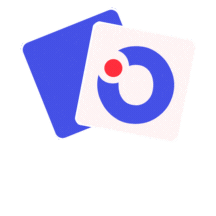
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
15-110: Principles of Computing File Processing Lecture 17, November 06, 2018 Mohammad Hammoud Carnegie Mellon University in Qatar
Today Last Session: Sequences- Part IV (Dictionaries) Today s Session: Files: Why Files File Processing File Functions Examples Announcement: HA05 will be out on November 08. It is due on November 17 by midnight
Files All data that we store in lists, tuples, and dictionaries within any program are lost when the program ends These data structures are kept in volatile memory (i.e., RAM) To save data for future accesses, we can use files, which are stored on non-volatile memory (usually on disk drives) Files can contain any data type, but the easiest files to work with are those that contain text You can think of a text file as a (possibly long) string that happens to be stored on disk!
File Processing Python has several functions for creating, reading, updating, and deleting files To create and/or open an existing file in Python, you can use the open() function as follows: <variable> = open(<file_name>, <mode>) <variable> is a file object that you can use in your program to process (i.e., read, write, update, or delete) the file
File Processing <file_name> is a string that defines the name of the file on disk <mode> can be only 1 of the following four modes: "r : This is the default mode; It allows opening a file for reading; An error will be generated if the file does not exist "a : It allows opening a file for appending; It creates the file if it does not exist "w : It allows opening a file for writing; It creates the file if it does not exist "x : It allows creating a specified file; It returns an error if the file exists
File Processing In addition, you can specify if the file should be handled as a binary (e.g., image) or text file "t : This is the default value; It allows handling the file as a text file "b : It allows handling the file as a binary fine Example: f = open("file1.txt") is equivalent to f = open("file1.txt , rt ) When opening a file for reading, make sure it exists, or otherwise you will get an error!
Reading a File The open() function returns a file object, which has a read() method for reading the content of the file f = open("file1.txt", "r") data = f.read() print(data) print(type(data)) The type of data is str, which you can parse as usual!
Reading a File You can also specify how many characters to return from a file using read(x), where x is the first x characters in the file f = open("file1.txt", "r") data = f.read(10) print(data) print(type(data)) This will return the first 10 characters in the file
Reading a File You can also use the function readline() to read one line at a time f = open("file1.txt", "r") str1 = f.readline() print(str1) str2 = f.readline() print(str2) print(f.readline()) This file contains some information about sales Total sales today = QAR100,000 Sales from PCs = QAR70,000 This is the content of the file
Reading a File You can also use the function readline() to read one line at a time f = open("file1.txt", "r") str1 = f.readline() print(str1) str2 = f.readline() print(str2) print(f.readline()) This file contains some information about sales Total sales today = QAR100,000 Sales from PCs = QAR70,000 Note that the string returned by readline() will always end with a newline, hence, two newlines were produced after each sentence, one by readline() and another by print()
Reading a File You can also use the function readline() to read one line at a time f = open("file1.txt", "r") str1 = f.readline() print(str1, end = ) str2 = f.readline() print(str2, end = ) print(f.readline(), end = ) This file contains some information about sales Total sales today = QAR100,000 Sales from PCs = QAR70,000 Only a solo newline will be generated by readline(), hence, the output on the right side
Looping Through a File Like strings, lists, tuples, and dictionaries, you can also loop through a file line by line f = open("file1.txt", "r") This file contains some information about sales Total sales today = QAR100,000 Sales from PCs = QAR70,000 for i in f: print(i, end = "") Again, each line returned will produce a newline, hence, the usage of the end keyword in the print function
Writing to a File To write to a file, you can use the w or the a mode in the open() function alongside the write() function as follows: f = open("file1.txt", "w") f.write("This file contains some information about sales\n") f.write("Total sales today = QAR100,000\n") f.write("Sales from PCs = QAR70,000\n") f.close() Every time we run this code, the same above content will be written (NOT appended) to file1.txt since we are using the w mode If, alternatively, we use the a mode, each time we run the code, the same above content will be appended to the end of file1.txt
Writing to a File To write to a file, you can use the w or the a mode in the open() function alongside the write() function as follows: f = open("file1.txt", "w") f.write("This file contains some information about sales\n") f.write("Total sales today = QAR100,000\n") f.write("Sales from PCs = QAR70,000\n") f.close() Also, once we are finished writing to the file, we should always close it using the close() function. This will ensure that the written content are pushed from volatile memory (i.e., RAM) to non-volatile memory (i.e., disk), thus preserved
Writing to a File You can also write information into a text file via the already familiar print() function as follows: f = open("file1.txt", "w") print("This file contains some information about sales", file = f) print("Total sales today = QAR100,000", file = f) print("Sales from PCs = QAR70,000", file = f) f.close() This behaves exactly like a normal print, except that the result will be sent to a file rather than to the screen
Deleting a File To delete a file, you must first import the OS module, and then run its os.remove() function import os os.remove("file1.txt") You can also check if the file exists before you try to delete it import os if os.path.exists("file1.txt"): os.remove("file1.txt") else: print("The file does not exist")
Example: Copy a File import os def copyFile(name): if type(name) is str and os.path.exists(name): l = name.split(".") new_name = l[0] + "_copy." + l[1] f_source = open(name, "r") f_destination = open(new_name, "w") f_destination.write(f_source.read()) f_source.close() f_destination.close() else: print("The file does not exist!") copyFile("file1.txt")
Example: Word Count A common utility on Unix/Linux systems is a small program called wc which, if called for any file, returns the numbers of lines, words, and characters contained therein We can create our version of wc in Python as follows: import os def wordCount(name): if type(name) is str and os.path.exists(name): f = open(name) l_c = 0 w_c = 0
Example: Word Count c_c = 0 for i in f: l_c = l_c + 1 list_of_words = i.split() w_c = w_c + len(list_of_words) for j in list_of_words: c_c = c_c + len(j) dic = {"Line Count": l_c, "Word Count": w_c, "Character Count": c_c} return dic else: print("The file does not exist!") print(wordCount("file1.txt"))
Next Class Practicing & Discussing HW05 Problems