If-Else-If Statements in Programming
The if-else-if statement in programming allows for multiple conditions to be evaluated in sequence. This enables more complex decision-making processes based on different scenarios. With examples and animations, explore how to use if-else-if statements effectively in your code.
Download Presentation
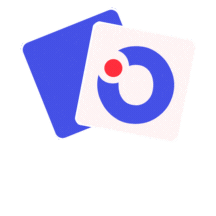
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
The if-else if Statement The general form of the if-elseif statement is as follows: if (Boolean expression) { statement(s) } else if (Boolean expression) { statement(s) } else if (Boolean expression) // Incl. as many else ifs as needed for the alternatives { statement(s) } else { statement(s) } 24
Example Example if (x > 0) { System.out.println(x + " is positive"); } else if (x == 0) { System.out.println(x + " is zero"); } else { System.out.println(x + " is negative"); } 25
animation Trace if-elseifstatement Suppose score is 70.0 The condition is false if (score >= 90.0) { grade = 'A'; } else if (score >= 80.0) { grade = 'B'; } else if (score >= 70.0) { grade = 'C'; } else if (score >= 60.0) { grade = 'D'; } else { grade = 'F ; } 26
animation Trace if-elseifstatement Suppose score is 70.0 The condition is false if (score >= 90.0) { grade = 'A'; } else if (score >= 80.0) { grade = 'B'; } else if (score >= 70.0) { grade = 'C'; } else if (score >= 60.0) { grade = 'D'; } else { grade = 'F ; } 27
animation Trace if-elseifstatement Suppose score is 70.0 The condition is true if (score >= 90.0) { grade = 'A'; } else if (score >= 80.0) { grade = 'B'; } else if (score >= 70.0) { grade = 'C'; } else if (score >= 60.0) { grade = 'D'; } else { grade = 'F ; } 28
animation Trace if-elseifstatement Suppose score is 70.0 grade is C if (score >= 90.0) { grade = 'A'; } else if (score >= 80.0) { grade = 'B'; } else if (score >= 70.0) { grade = 'C'; } else if (score >= 60.0) { grade = 'D'; } else { grade = 'F ; } 29
animation Trace if-elseifstatement Suppose score is 70.0 Exit the if statement if (score >= 90.0) { grade = 'A'; } else if (score >= 80.0) { grade = 'B'; } else if (score >= 70.0) { grade = 'C'; } else if (score >= 60.0) { grade = 'D'; } else { grade = 'F ; } 30
Nested if-else Statements An if-else statement can contain any sort of statement within it. In particular, it can contain another if-else statement. An if-else may be nested within the "if" part. An if-else may be nested within the "else" part. An if-else may be nested within both parts. 31
Example 1 Example 1 32
Example 2 Example 2 Given three values, x, y, and z, determine the largest value and assign this value to the variable largest. 33