Control Statements in C Programming
Control statements in C programming play an essential role in managing the flow of operations through iterative and conditional statements. Learn about for, while, and do-while loops, along with if-else and switch-case statements to effectively control program execution.
Download Presentation
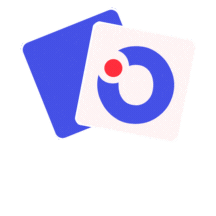
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
1 Developed by Mr. Sandeep G. Shukla
Control Statement In programming we need to sometimes control the flow of operation other than just the sequential statements. In this case we need the control statements. Control statements are classified into two viz. the iterative statements and conditional statement. Iterative statements are use to perform certain operation repetitively for certain number of times. In C we have three iterative statement viz. for loop, while loop and do-while loop. Conditional statements are use to perform the operations based on a particular condition i.e. if a condition is true perform one task else perform another task. In C we have two conditional statement namely if- else statement and switch-case statements. 2
FOR LOOP IN C A for loop is a repetition control structure that allows you to efficiently write a loop that needs to execute a specific number of times. Syntax: The syntax of a for loop in C programming language is: for ( init; condition; increment ) { statement(s); } 3
Ex. Write a program in c to generate first 20 Fibonacci numbers #include<stdio.h> #include<conio.h> void main() { int a=0, b=1,c,i,n=20; printf( Fibonacci Series ); printf( \n %d \n %d ,a,b); for(i=1; i<=n-2; i++) { c=a+b; printf( \n%d ,c); a=b; b=c; } getch(); } Fibonacci Series 0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987 1597 2584 4181 4
WHILE LOOP IN C A while loop statement language in C programming executes a target statement as long as a given condition is true. repeatedly Syntax: T he syntax of a while loop in C programming language is: while(condition) { statement(s); } 5
Ex. Write a C program to generate prime numbers between 1 to n. Enter a number: 10 The following are the prime numbers 2 3 5 7 11 13 17 19 23 29 while(n!=0) { x++; i=2; while(x%i!=0) { i++; } if(x==i) { printf("\n%d",x); n--; } } getch(); } #include<stdio.h> #include<conio.h> void main() { int i,n,x=1; clrscr(); printf("Enter a number:"); scanf("%d",&n); printf("\nThe following are the prime numbers "); 6
DO...WHILE LOOP IN C Unlike for and while loops, which test the loop condition at the top of the loop, the do...while loop in C programming language checks its condition at the bottom of the loop. A do...while loop is similar to a while loop, except that a do...while loop is guaranteed to execute at least one time . Syntax: T he syntax of a do...while loop in C programming language is: do { statement(s); }while( condition ); 7
C - IF STATEMENT An if statement consists of a Boolean expression followed by one or more statements. Syntax: The syntax of an if statement in C programming language is: if(boolean_expression) { /* statement(s) will execute if the Boolean expression is true */ } 8
C - IF...ELSE STATEMENT An if statement can be followed by an optional else statement, which executes when the Boolean expression is false. Syntax: The syntax of an if...else statement in C programming language is: if(boolean_expression) { /* statement(s) will execute if the Boolean expression is true */ } else { /* statement(s) will execute if the Boolean expression is false */ } 9
C - SWITCH STATEMENT A switch statement allows a variable to be tested for equality against a list of values. Each value is called a case, and the variable being switched on is checked for each switch case. Syntax: The syntax for a switch statement in C programming language is as follows: switch(expression) { case constant-expression : statement(s); break; /* optional */ case constant-expression : statement(s); break; /* optional */ /* you can have any number of case statements */ default : /* Optional */ statement(s); } 10
Ex. Write a C program to read an integer and display each of the digit of the integer in English. #include<stdio.h> #include<conio.h> case 2:printf("Two "); break; case 3:printf("Three "); break; case 4:printf("Four "); break; case 5:printf("Five "); break; case 6:printf("Six "); break; case 7:printf("Seven "); break; case 8:printf("Eight"); break; case 9:printf("Nine "); break; } } getch(); } void main() { int n,digit,rev=0; clrscr(); printf("Enter a number:"); scanf("%d",&n); while(n!=0) { digit=n%10; rev=rev*10+digit; n=n/10; } while(rev!=0) { digit=rev%10; rev=rev/10; switch(digit) { case 0:printf("Zero "); break; case 1:printf("One "); break; Enter a number: 345 Three Four Five 11
Loop Control Statements Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed. C supports the following control statements. Control Statement Description Terminates the loop or switch statement and transfers execution immediately following the loop or switch. break statement to the statement Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating. continue statement Transfers control to the labeled statement. Though it is not advised to use goto statement in your program. goto statement 12
C - Pointers A pointer is a variable whose value is the address of another variable, i.e., direct address of the memory location. Like any variable or constant, you must declare a pointer before you can use it to store any variable address. The general form of a pointer variable declaration is: type*var-name; Here, type is the pointer's base type; it must be a valid C data type and var-name is the name of the pointer variable. The asterisk * you used to declare a pointer is the same asterisk that you use for multiplication. However, in this statement the asterisk is being used to designate a variable as a pointer. Following are the valid pointer declaration: int *ip; /* pointer to an integer */ double *dp; /* pointer to a double */ float *fp; /* pointer to a float */ char *ch /*pointer to a character */ a 39 Variable name 46768 Memory Address ip=46768; Example :ip=&a;
How to use Pointers? There are few important operations, which we will do with the help of pointers very frequently. (a) we define a pointer variable (b) assign the address of a variable to a pointer and (c) finally access the value at the address available in the pointer variable. This is done by using unary operator * that returns the value of the variable located at the address specified by its operand. Following example makes use of these operations:
C Pointers in Detail Pointers have many but easy concepts and they are very important to C programming. There are following few important pointer concepts which should be clear to a C programmer: Concept Description C - Pointer arithmetic There are four arithmetic operators that can be used on pointers: ++, --, +, - C - Array of pointers You can define arrays to hold a number of pointers. C - Pointer to pointer C allows you to have pointer on a pointer and so on. Passing pointers to functions in C Passing an argument by reference or by address both enable the passed argument to be changed in the calling function by the called function. Return pointer from functions in C C allows a function to return a pointer to local variable, static variable and dynamically allocated memory as well.
MCQs. What is the final value of x when the code int x; for(x=0;x<=10;x++) a. 10 b. 9 c. 0 d. 11 Looping in the program means a. Branching to a specific branch or label in program b. Repeating a given set of statement c. Both of above Correct Ans. D d. None of above Correct Ans. B Find the output of the following C code #include<stdio.h> #include<string.h> void main() { int i=0; for(;i<=2;) printf( %d ,++i); a. 0 1 2 Correct Ans. B b. 1 2 3 c. 0 1 2 3 d. Infinite loop
MCQs. Find the output of the following code. #include<stdio.h> void main() { int s=0; while(s++<10) { } } a. 1 2 3 4 5 6 7 8 9 b. 1 2 3 10 Correct Ans. B if(s>3 && s<10) continue; printf( \n%d\t ,s); c. 4 5 6 7 8 9 10 d. 4 5 6 7 8 9 The do-while loop is an entry controlled loop a. True b. False Correct Ans. B c. Depends on the condition d. None of the above
MCQs. Find the output of the following program #include<stdio.h> void main() { int i,j; for(i=1;i<=2;i++) { } } a. Hello Hello Hello Hello Hello Hello Hello Hello Correct Ans. B for(j=1;j<=3;j++) { } printf( \n ); printf( Hello ); b. Hello Hello Hello c. Hello Hello d. Hello Hello Hello Hello Hello Hello Hello Hello Hello Hello Hello Hello Hello
MCQs. Find the output of the following program #include<stdio.h> void main() { int n=400; if(n%10==0) { } else { } } a. Yes Correct Ans. A printf( Yes ); printf( No ); b. No c. Compilation error d. None of the above
MCQs. Find the output of the following program void main() { int i,j=6; for(;i=j;j-=2) printf( %d ,j); } a. Error Find the correct output void main ( ) { int a=2,b=0,c=-2; if(b,a,c) printf( True ); else printf( False ); } a. True Correct Ans. C b. Garbage value c. 642 d. 6420 Correct Ans. A b. False c. Compile time error d. Run time error