Advanced Programming Platforms Overview
Delve into advanced programming platforms like Eclipse for C, C++, Java, and Android development. Learn to write Hello World programs in C++ and Java, explore data types, data structures, and file models. Enhance your programming skills with these insightful tutorials.
Download Presentation
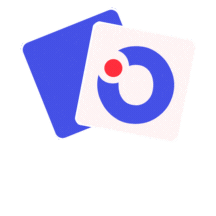
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Fundamentals of Programming Languages-II Unit II Introduction to Advanced Programming Platforms 1
Eclipse Programming Platform: C, C++, Java Eclipse platform is an open-source (free) integrated development environment (IDE). Eclipse comes in different flavors like Juno, Indigo, Helios. Eclipse IDE supports for different programming languages like C, C++, Java. Even Android applications development can be done in eclipse. 2
Hello World in C++: Select File -> New -> Project. Select C++ Project and click Next. The C++ Project wizard opens. Select Hello World C++ Project from Project Type section of Window Provide name to project. Click on Finish Build auto generated C++ code by selecting Project -> Build Project and run sample code to view Hello World ! on console. 3
To write Hello world program in JAVA: Select File->New->Project. Select "Java Project from project list. Click "Next". Enter a project name into the Project name field, for example, "HelloWorld Project". Click "Finish"--It will ask you if you want the Java perspective to open. (select Yes .) Now create a class file using following steps. Explore the project folder in package explorer and right click on src folder. Select New->class, provide class name and check the box in front of public static void main(); Click on finish . Automatic generated code skeleton will appear on screen. Add System.out.println( Hello World! ); in main function Save and run the program to view Hello World! on screen. 5
Add this line in generated program skeleton 6
Data Piece of information Can be number or string or any type of record Type of data Scalar Eg. integer 1234 Composite or structure eg. PRN: college code, branch code, student-id 7
File Models Files: Files are used to store large amount of data in permanent form, on floppy disk, hard disk, tape or other form of secondary storage. Storage Types of File: Text File : ASCII characters format are stored. (C program) Binary File : Same format as in which it is stored in memory. (.o, .exe) Models Structured or Unstructured. UnStructured Files: Unstructured sequence of data. Interpretation of meaning and structure of data are entirely up to application program. Sharing of file is easy. UNIX and MS-DOS use this model. 8
File Models Structured Files: When the file contents are organized in specific format, it is known as structured file. Ex. Log file. A Record is smallest unit of file data. Two Types: Files with non-index records. Files with indexed records. 9
File Handling Operations Some of the important file operations are, fopen() Open an existing file or create a new file for use and load contents in main memory. fclose() Closes a file getc() Reads a character from a file putc() Write a character to a file fprintf() Write a set of data values to a file fscanf() Read a set of data values from a file fseek() Sets the pointer to a desired location in the file ftell() Gives the current position in the file(offset from beginning in number of bytes) 10
Data Structure Scheme for organising, storing and manipulating data. It is Linear or Non Linear. Linear Non Linear Data attached to its previous & next Data is attached to many other data in specific way to reflect relationship Arranged in linear sequence Not in sequence Implemented using Arrays Use of pointers Traversed in linear fashion Traversed in non linear fashion Easy to implement Difficult to implement Insertion & Deletion is possible in linear fashion Sequential Updating or Additition not possible 11
Linked Lists Static Memory Allocation Memory is allocated at compile time. Ex. Array Limitations: 1. Wastage of memory, as maximum required memory should be allocated at declaration. 2. Insertion and deletion of middle data requires movement of data (which is expensive). Solution to these limitations is . Dynamic Memory Allocation i.e. memory is allocated at compile time. 12
Linked List: Dynamically allocated memory blocks (placed anywhere in memory) are connected by maintaining address of next and /or previous memory block. Memory block is logically represented by node. Fig: Node Sequential organization of nodes form a Linked List Address of next node is maintained in previous node. Last Node has address NULL. Unidirectional (Singly) Linked List (SLL):- Data Address of Next Node 34 54 78 Fig: Unidirectional Linked List 13
Unidirectional (Singly) Circular Linked List (SCLL):- Last node stores address of First / Head node. 34 54 78 Bidirectional Linked List (Doubly Linked List): Backward and forward movement in list is possible. RLINK of last node and LLINK of first node is NULL. NODE: Address of Left Node DATA Address of Right Node Example 101 102 105 Bidirectional Circular Linked List: LLINK of first node stores address of last node and RLINK of last node stores address of first node. DATA DATA DATA 14
Linked List vs Array:- . Array Linked List Elements stored in linear order, accessible with index. Elements stored in linear order, accessible with Links. Fixed Size. Not Fixed Variable Size. Inserting data into array is difficult as we need to move data Inserting data is easy as we have to change only address field. Searching is time consuming as we have to traverse whole list. Extra memory required to store link field. Searching is easy because of index No extra memory required to store link field. Easy to code. More complex too code. Memory may be wasted as it is required to declare at start. Memory is not wasted, allocation is as per requirement. Binary search can be performed. Cannot perform Binary search. 15
Dynamic Memory Allocation in C Gives Flexibility to obtain memory space at execution time or release memory space when not needed. 4 library functions in C, available in stdlib.h . Malloc Calloc Realloc Free Malloc Memory Allocation Allocates required space & returns pointer to first byte of allocated space. Function malloc() reserves block of memory. ptr = (cast-type*) malloc(byte-size) ptr = (int*) malloc( 10 * sizeof (int) ) If space is insufficient then allocation fails and returns NULL pointer. 16
Dynamic Memory Allocation in C Calloc contiguousallocation Allocates space for an array elements, initialise to zero and returns pointer to memory. calloc() allocates multiple blocks of memory each of same size. ptr = (cast-type*) calloc( n , element-size) ptr = (float*) calloc( 10 , sizeof (float) ) Allocates contiguous space in memory for an array of n elements. 17
Dynamic Memory Allocation in C Realloc Re - Allocation Allocates more new space if previously allocated memory is insufficient. realloc() allocates more memory. ptr = realloc( ptr , newsize) ptr = realloc( ptr , 20 * sizeof (int) ) Reallocates memory for pointer ptr with specified size. 18
Dynamic Memory Allocation in C Free De-allocates previously allocated space. free()releases memory space used. free( ptr ) Deallocates the space in memory pointed by pointer ptr. Programmers must use free() explicitly to release space. 19
Dynamic Memory Allocation in C++ new operator is used for allocating memory in constructor. delete operator is used for de-allocating same memory with destructor. ptr = new int(10); Memory is allocated for integer with initial value 10, pointed by pointer ptr. delete ptr; Memory is freed allocated to pointer ptr. 20
Application of Linked List For sorted list. Implementing other data structure. Hold records of students. Representing polynomials. Dynamic memory management. Representing sparse matrix. Balancing paranthesis. 21
Trees A Root Node D Height = 2 B C Intermediate nodes H E G F Leaf Node Siblings Basic Terminologies with example: Root Node: Node with no parent (In fig. 1 Node A is root) Leaf Node: Node with no child ((In fig. 1 Node E,F,G,H are leaf nodes) Degree: Total number of incoming & outgoing edges (In fig. 1 degree of node B is 3) Indegree: Total number of incoming edges.(In fig.1 indegree of node B is 1) Outdegree: Total Number of out going edges.(In fig. 1 outdegree of node B is 2) Height: distance from root to leaf I(n fig. 1 degree of node B is 2) fig. 1: Tree 22
Trees A Root Node D Height = 2 B C Intermediate nodes H E G F Leaf Node Siblings Types of tree: Binary Tree: Binary Search Tree: Strictly Binary Tree: Full Binary Tree: fig. 2: Tree 23
8 A Trees 4 B 12 D 1 E 6 F 14 10 H G 5 F I fig.3: Binary Search Tree fig. 2: BinaryTree A A B D B D E F H G E H F G I J fig. 4: Strictly BinaryTree 24 fig.5: Fully Binary Tree
Application of Tree Used to manipulate hierarchical data. Easy to search information. Used to manipulate sorted list data. Used in internet protocol to identify how to reach computers in their networks. 25
TREES Graph is collection of nodes called as vertices and connections between them called as edges. Tree can be a graph without cycles. Spanning subgraph/tree spans all vertices of original graph Mining spanning tree (MST) has minimum total weight To find MST: Kruskal Algorithm Prim s Algorithm 26
Conti First step of Kruskal is to select an edge First step of Prim s is to select a vertex 27
Java Programming Language Java is Object Oriented language that supports portability feature. Platform Independent (Portable) Write-Once Run-Anywhere Platform independent means program created on one operating system can be run on any other operating system unlike C, C++. Java Virtual Machine (JVM) makes Java program platform independent. A Java Virtual Machine (JVM) is a software that implements the Java run time system and executes Java applications. Convert byte code into machine level representation. 28 28
Introduction To Java Virtual Machine(JVM) Java is both compiled and interpreted language. Java compiler produces an intermediate code known as bytecode for a machine, known as JVM. JVM loads, verifies and executes bytecode on respective platform. Byte code (*.class) Java Program (*.java) Java Compiler LINUX JVM JVM Windows JVM Can run on multiple platform JavaOS JVM 29
Classes A class is a collection of fields (data) and methods (procedure or function) that operate on that data. A class is defined as category or template of objects Implementation of Class: Defines new data type. Object is instance of class. Two types of members: Data members and Member functions Data Members: data within class, called as instance variables. Member Functions: Functionalities offered by class. Also called as Methods. 30
Classes Example: Class Circle { private: int radius; float area,circumference; Class Name Circle Public: void CirCircumference() { circumference=2*3.14*radius; cout<< Circumferenceof circle is: << circumference; } Integer radius Float area Data Void CirCircumference() Void CirArea() Methods void CirArea() { area=3.14*radius*radius; cout<< Area of circle is <<area; } 31
Objects Objects are instance of class. They have specific value for the data members. Implementation of Object: Object creation is twofold process. First we create object. Then we allocate memory. Syntax : <class-name> <object-name> = new <class-name>(); Person p1 = new Person(); To access members of class : Syntax: <object-name> <data-member> p1 name= Sachin ; p1 getName(); 32
Data Members Data Type Size (bits) (bytes) Value Range byte 8 bits - 1 byte -128 to 127 short 16 bits 2 bytes -32768 to 32767 int 32 bits 4 bytes -2,147,483,648 to 2,147,483,647 -9,223,372,036,854,755,808 to 9223,372,036,854,755,807 long 64 bits 8 bytes float 32 bits 4 bytes 1.4 e 045 to 3.4 e+038 double 64 bits 8 bytes 4.9 e -324 to 1.8 e+308 char 16 bits 2 bytes 0 to 65536 boolean 1 bit 0 or 1 (True or False) 33
Constructor Special member function of class. Name of constructor is same as class name. Can be parameterised or non-parameterised. Constructor with no parameter is Default constructor. Constructor is used to initialise data members and allocate memory to object. Constructor does not have any return type. Syntax : class person { String name; int id; Person() { id = 0; name= Java . } 34
Constructor class person { String name; int id; person() { id = 0; name= Java . } person(String sname, int sid) { name=sname; id = sid; } } 35
Constructor class Demoperson { public static void main(String s[]) { person p1 = new person(); person p2 = new person( ABC , 1); System.out.print( Name is = + p1.name); System.out.print( ID is = + p1.id); System.out.print( Name is = + p2.name); System.out.print( ID is = + p2.id); } } Name is = ABC ID is = 1 Output : Name is = Java ID is = 0 36
Access Modifier Public : members can be accessed by any other class. Private: members can be accessed with in class. Protected: members can be accessed by sub classes. Default: when no access modifier is specified. members can be accessed by any other class in the package. 37
Signals: Signals occurs when unusual condition occurs. When signal is raised which is of the interest of JVM then a signal handler is called. Signal are used to communicate between processes running simultaneously through wait(), notify() and notifyAll(). Various Types of signal are : 1. Exception : when unusual condition occurs. 2. Errors: JVM raises SIGABRT if condition not recovers. 3. Interrupts: raised asynchronously from outside JVM 4. Controls: handle control signal (control handler routine) 38
Exception Types : ArithmeticException : math operation NullPointerException : access object with null reference IOException : illegal I/O Operation performed RunTimeException : general run time exception Exception : most general type exception ArrayIndexOutOfBoundException : ArrayStoreException: wrong object store in array EmptyStackException : pop element from empty stack 39 39
Exception Handling Try .catch block (for exception thrown) catch (handle exception thrown by try ) try { //exception generate here } catch(Type of Exception e) { //exception handle here } 4040
Event Methods An event(trigger in system) is an object that describe a state change in a source. Some of the activities that causes event to be generated like: Pressing button Entering a character through keyboard Selecting an item from list Event methods in java Events are objects in Java. The events belongs to java.awt.event package There are different type of events: 1. Key Event 2. Mouse Events 3. Windows Events 41 41
Event Methods: 1. Key Event: keyTyped() keyPressed() keyReleased() Mouse Events: mouseCliked() mousePressed() mouseReleased() mouseEntered() mouseExited(). Windows Event: windowsOpened() windowsclosed() windowsclosing() 2. 3. 42
File Handling in JAVA To handle operations on file there are 3 classes: 1. File: to read and modify information 2. FileInputStream: for reading file 3. FileOutputStream: for writing file Note: To use these classes in program user has to import java.io package 43
Basics of Web Technology using HTML HTML is Hyper Text Markup Language HTML describes the content and format of web pages using tags. Document content is what we see on the webpage. Tags are the HTML codes that control the appearance of the document content. web browser interprets tags and display the content accordingly. 44
Basic Hello World HTML Program Open your text editor (Notepad). Type the following lines of code into the document: <html> <head> <title> First web page</title> </head> <body> Hello World ! <IMG SRC= pic1.jpg WIDTH=30HEIGHT=30 ALIGN= left > <a href= http://www.METIOE.edu > Link to MET BKC</a> </body> </html> Save the file with <filename>.html extension. 45
Output of Hello World HTML Program in Internet Browser Output of Img Hello World ! tag Link to MET BKC Output of Anchor tag 46