Understanding State Management in ASP.NET: A Comprehensive Guide
Explore the vital concepts of session and state management in ASP.NET web applications. From preserving control state to managing data at different levels, discover the importance of client-side and server-side methods along with practical examples. Uncover the various types of state management techniques and how they play a crucial role in maintaining user data and application states dynamically.
Download Presentation
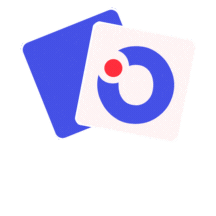
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
State management State management means to preserve state of a control, web page, object/data, and user in the application explicitly because all ASP.NET web applications are stateless, i.e., by default, for each page posted to the server, the state of controls is lost. Nowadays all web apps demand a high level of state management from control to application level.
Types of state management State Mgmt Client Side Server Side Hidden Field Control State Query Strings View State Cookies Session Application
Client side methods 1. Hidden field Hidden field is a control provided by ASP.NET which is used to store small amounts of data on the client. It store one value for the variable and it is a preferable way when a variable's value is changed frequently. Hidden field control is not rendered to the client (browser) and it is invisible on the browser. A hidden field travels with every request like a standard control s value.
<asp:HiddenField ID="HiddenField1" runat="server" /> protected void Page_Load(object sender, EventArgs e) { if (HiddenField1.Value != null) { int val= Convert.ToInt32(HiddenField1.Value) + 1; HiddenField1.Value = val.ToString(); Label1.Text = val.ToString(); } }
Contains a small amount of memory Direct functionality access
View state sometimes the user needs to preserve data temporarily after a post back, then the view state is the preferred way for doing it. It stores data in the generated HTML using hidden field not on the server.
protected void Page_Load(object sender, EventArgs e) { if (IsPostBack) { if (ViewState["count"] != null) { int ViewstateVal = Convert.ToInt32(ViewState["count"]) + 1; View.Text = ViewstateVal.ToString(); ViewState["count"]=ViewstateVal.ToString(); } else { ViewState["count"] = "1"; } } } // Click Event protected void Submit(object sender, EventArgs e) { View.Text=ViewState["count"].ToString(); }
View State It is page-level State Management Used for holding data temporarily Can store any type of data Property dependent
Cookies A set of Cookies is a small text file that is stored in the user's hard drive using the client's browser. Cookies are just used for the sake of the user's identity matching as it only stores information such as sessions id's, some frequent navigation or post-back request objects Whenever we get connected to the internet for accessing a specific service, the cookie file is accessed from our hard drive via our browser for identifying the user.
Persistent Cookie Cookies having an expiration date is called a persistent cookie. This type of cookie reaches their end as their expiration dates comes to an end. In this cookie we set an expiration date. Persistent cookies are stored in a text file on the clients computer. public void SetPersistentCookies(string name, string value) { HttpCookie cookie = new HttpCookie(name); cookie.Value = value; cookie.Expires = Convert.ToDateTime( 12/12/2018 ); Response.Cookies.Add(cookie); }
Non-Persistent Cookie Non-persistent types of cookies aren't stored in the client's hard drive permanently. Non-Persistent cookies are stored in RAM on the client and are destroyed when the browser is closed. It maintains user information as long as the user access or uses the services. Its simply the opposite procedure of a persistent cookie. public void SetNonPersistentCookies(string name, string value) { HttpCookie cookie = new HttpCookie(name); cookie.Value = value; Response.Cookies.Add(cookie); }
Store information temporarily It's just a simple small sized text file Can be changed depending on requirements User Preferred Requires only a few bytes or KBs of space for creating cookies
Control State Control state is based on the custom control option. For expected results from CONTROL STATE we need to enable the property of view state. Used for enabling the View State Property Defines a custom view View State property declaration Can't be modified Accessed directly or disabled
Query Strings Query strings are used for some specific purpose. These in a general case are used for holding some value from a different page and move these values to the different page. The information stored in it can be easily navigated to one page to another or to the same page as well.
// Getting data if (Request.QueryString["number"] != null) { View.Text = Request.QueryString["number"]; } // Setting query string int postbacks = 0; if (Request.QueryString["number"] != null) { postbacks = Convert.ToInt32(Request.QueryString["number"]) + 1; } else { postbacks = 1; } Response.Redirect("default.aspx?number=" + postbacks);
Server side 1. Session Session management is a very strong technique to maintain state. Generally session is used to store user's information and/or uniquely identify a user (or say browser). The server maintains the state of user information by using a session ID. When users makes a request without a session ID, ASP.NET creates a session ID and sends it with every request and response to the same user. Session["Count"] = Convert.ToInt32(Session["Count"]) + 1;//Set Value to The Session Label2.Text = Session["Count"].ToString(); //Get Value from the Sesion
session storage mechanisms In Proc mode State Server mode SQL Server mode Custom mode
In Process mode In proc mode is the default mode provided by ASP.NET. In this mode, session values are stored in the web server's memory (in IIS). If there are more than one IIS servers then session values are stored in each server separately on which request has been made. <configuration> <sessionstate mode="InProc" cookieless="false" timeout="10" stateConnectionString="tcpip=127.0.0.1:80808" sqlConnectionString="Data Source=.\SqlDataSource;User ID=userid;Password=password"/> </configuration>
In State Server mode This mode could store session in the web server but out of the application pool. But usually if this mode is used there will be a separate server for storing sessions, i.e., stateServer. The benefit is that when IIS restarts the session is available. <configuration><sessionstate mode="stateserver" cookieless="false" timeout="10" stateConnectionString="tcpip=127.0.0.1:42424" sqlConnectionString="Data Source=.\SqlDataSource;User ID=userid;Password=password"/> </configuration>
In SQL Server mode Session is stored in a SQL Server database. This kind of session mode is also separate from IIS, i.e., session is available even after restarting the IIS server. This mode is highly secure and reliable but also has a disadvantage that there is overhead from serialization and deserialization of session data. <configuration> <sessionstate mode="sqlserver" cookieless="false" timeout="10" stateConnectionString="tcpip=127.0.0.1:4 2424" sqlConnectionString="Data Source=.\SqlDataSource;User ID=userid;Password=password"/> </configuration>
Custom Session mode Generally we should prefer in proc state server mode or SQL Server mode but if you need to store session data using other than these techniques then ASP.NET provides a custom session mode. This way we have to maintain everything customized even generating session ID, data store, and also security.
Description Attributes Cookieless true/false Indicates that the session is used with or without cookie. cookieless set to true indicates sessions without cookies is used and cookieless set to false indicates sessions with cookies is used. cookieless set to false is the default set. timeout Indicates the session will abound if it is idle before session is abounded explicitly (the default time is 20 min). StateConnectionString Indicates the session state is stored on the remote computer (server). This attribute is required when session mode is StateServer SqlConnectionString Indicates the session state is stored in the database. This attribute is required when session mode is SqlServer.
Application Application state is a server side state management technique. The date stored in application state is common for all users of that particular ASP.NET application and can be accessed anywhere in the application. It is also called application level state management. Application["Count"] = Convert.ToInt32(Application["Count"]) + 1; //Set Value to The Application Object Label1.Text = Application["Count"].ToString(); //Get Value from the Application Object
Exception Exceptions are nothing but unseen error occurs when executing code logic. public partial class _Default : Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { string connectionString = ConfigurationManager.ConnectionStrings["MyConn"].Connecti onString; string selectSQL = "SELECT * FROM tblEmployees"; SqlConnection con = new SqlConnection(connectionString); SqlCommand cmd = new SqlCommand(selectSQL, con); SqlDataAdapter adapter = new SqlDataAdapter(cmd); DataSet ds = new DataSet(); adapter.Fill(ds, "Employees"); GridView1.DataSource = ds; GridView1.DataBind(); } } }
Problem It does not make any sense to the end user although it can be helpful for developers for debugging and investigating the issue. This exposure can help hackers to get information about your application that is not good according to security.
public partial class _Default : Page { protectedvoid Page_Load(object sender, EventArgs e) { if (!IsPostBack) { try { string connectionString = ConfigurationManager.ConnectionStrings["MyConn"].ConnectionString; string selectSQL = "SELECT * FROM tblEmployees1"; SqlConnection con = new SqlConnection(connectionString); SqlCommand cmd = new SqlCommand(selectSQL, con); SqlDataAdapter adapter = new SqlDataAdapter(cmd); DataSet ds = new DataSet(); adapter.Fill(ds, "Employees"); GridView1.DataSource = ds; GridView1.DataBind(); } catch (Exception ex) { // Log the exception Label1.Text = "Something Bad happened, Please contact Administrator!!!!"; } finally { } } } }