Understanding Authorization in Modern Applications
Tips for securing modern applications through authentication, authorization, and claims in ASP.Net Core. Learn the differences between authentication and authorization, the role of identity providers, how authorization works, and how to set up authorization in your application.
Uploaded on Sep 23, 2024 | 0 Views
Download Presentation
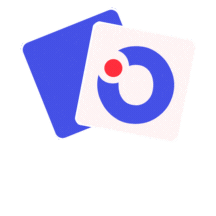
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Authorization in Asp.Net Core TIPS FOR SECURING MODERN APPLICATIONS
Jeff Zuerlein Software Developer, DBA, and some DevOps. Freelancer Blog @ BetterWithCode.com Email Jeff@Zuerlein.com
Authentication Vs. Authorization Authentication = Who is this user? Authorization = What can this user do?
What are Claims? Microsoft moved to a claims based model. A claim is a key-value pair that tells something about the user. Could come from an identity provider, database, or local storage. Does not tell what a user can do.
ClaimsIdentity vs. ClaimsPrincipal A ClaimsIdentity object is like a passport or drivers license. Contains descriptive information analogous to a claim. Issued by an entity that has validated the user. A ClaimsPrincipal represents a user. May contain multiple ClaimsIdentitys issued from different entities such as Twitter, Facebook, Microsoft, or your own identity provider.
Identity Providers Someone else takes on the risk of managing Identity. Issues security token (bearer token). Tokens are self contained Authentication is stateless Enables single sign-on. JSON Web Token Registered claims defined in the spec. Public claims defined by the who ever creates the token Private claims agreed to be used by issuer and consumer
How does authorization work? Static File Middleware A request is made. Authentication Authorization filter runs after routing, but before model binding or validation. If authorization fails, the filter returns an error to the user, and the action is not executed. Authorization Index Action Method If authorization is successful, the action method executes. Index View
Hello World of Authorization Setup Authorization in Startup.cs Create a ClaimsPrincipal Apply Authorization Attributes to Controllers or Actions.
Apply Authorization Attributes [Authorize] [AllowAnonymous] [Authorize(Roles = )] [Authorize(Policy = )]
What is a policy? A policy is a list of requirements that a user must meet in order to perform on operation. Demo Can a user enter the server room. The user must be an in the Security or Engineer role. The user s height must be greater than 12 and less the 10 . The user must have a current networking certification. The server room must be safe to enter.
Building Blocks of a Policy Policy CertificationRequirement CertificationHandler ServerRoomSafeRequirement SmokeDetectorHandler TemperatureHandler
Out of the box requirements RequireClaim(string ClaimType, IEnumerable<string> allowedValues) RequireRole(IEnumerable<string> allowedRoles) RequireUserName(string requiredName) RequireAuthenticatedUser RequireAssertion
Evaluating Handlers AuthorizationHandler AuthorizationHandlerContext OR AuthorizationResult AuthorizationHandler OR AuthorizationHandler OR AuthorizationHandler
Evaluating Handlers - Success AuthorizationHandler AuthorizationHandlerContext OR AuthorizationResult AuthorizationHandler OR AuthorizationHandler OR AuthorizationHandler
Evaluating Handlers Fail AuthorizationHandler AuthorizationHandlerContext OR AuthorizationResult AuthorizationHandler OR AuthorizationHandler OR AuthorizationHandler
AuthorizationHandlerContext Requirements only pass if a handler calls Succeed. Requirements always fail if any handler calls Fail. Handlers don t need to call Succeed or Fail. AuthorizationResult.Failure.FailedRequirements
Combining Authorization Requirements AuthorizationRequirement AND AuthorizationRequirement AND AuthorizationRequirement AND AuthorizationRequirement
DefaultAuthorizationService AuthorizeAsync Evaluates a list of requirements or a policy. Creates an AuthorizationHandlerContext Invokes each handler, and passes them the context Context contains AuthorizationResult Gives the context to the DefaultAuthorizationEvaluator Returns the result.
Unit Test Authorization Requirements Create a new IAuthorizationService with the requirement you need to test Create a new Claims Principal with / without the claim being evaluated Test the evaluation
Authentication Schemes Allows you to add specify what authentication methods can be used. Cookies JWTs OpenID [Authorize] attributes can require which authentication schemes are required. Policies can make an authentication scheme a requirement. Useful for MVC apps that also call WebAPIs
Resources Based Authorization User s ability to perform an operation is dependent on a resource. Can t use just [Authorize] because filters run before model binding occurs. Must implement the authorization check in code. Performance impact
Resource-Based Authorization Inject DefaultAuthorizationService into the controller with DI.
Resource-Based Authorization [Authorize] attribute ensures the user is authenticated. Policies used in [Authorize] can t contain resource-based requirements.
Challenge or Forbid ChallengeResult Not authorized because they are not logged in. ForbidResult Logged in but don t meet the requirements. MVC Web Application ChallengeResult will redirect to a login page. ForbidResult will redirect to an access denied page. Web Api ChallengeResult will return a 401 Unauthorized error response. ForbidResult will return a 403 Forbidden error response. In a SPA or Mobile app there is no redirection.
View Based Authorization _ViewImports.cshtml
Problems with Claims Based Authorization Identity is universal, permissions are application specific. Don t put permissions in a security token. Confusion of the meaning of the claim. Token Bloat Most authorization evaluations need more than the claims you get from an identity provider. End up using roles and group memberships. Authorization requirements and users change over time. Management needs to be handled outside of source code. Audit process for changes. Tokens have a lifetime, how quickly do authorization changes need to take effect?
Dream no small dreams! External Policy Management Alter permissions without recompiling. Clear Visibility Have the ability to see who has a permission, and audit. Centralized One UI to make changes. DRY Performant Keep authorization data close to the web server. Scheduled Changes Make changes and verify before they take effect.
Decorating ClaimsPrincipal Example: PolicyServer on GitHub Adds a stage in middleware to inject permissions into ClaimPrincipal. Allows the use of the [Authorize] attribute. Client library to make imperative checks. IsInRole HasPermission
SPA Example With Authorization Provider
Decorating ClaimsPrincipal Good Unit testing authorization requirements is easier without UserManager or repositories. No token bloat, permissions can be added by the application when request is made. Changes take effect immediately, not when token expires. Tooling can be leveraged for auditing, scheduling, and data manipulation. No changes to source code. Bad Probably costs an out of process call to modify ClaimsPrincipal. Depends on where authorization data is stored.