Understanding Client-Server Communication with Flask in Python
Explore the basics of implementing a client-server architecture using Flask in Python. Learn how to set up a server, create APIs, and interact with a client application. The process involves handling API calls, directing requests, and returning data to the client through the server.
Download Presentation
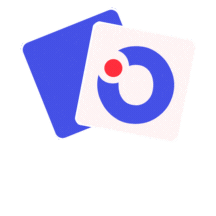
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Client-Server PYTHON AND FLASK
Basic Client - Server Client One Server Many types of clients All connected using a common protocol Browser HTTP(s) Web Server HTTP(s) Mobile App HTTP(s) Desktop Application Client Client
Web Servers Industrial Strength: Development: - IIS - Node - Apache - Flask - Nginx - httpd - Litespeed We will use Flask
Flask Python module Can host basic commands needed for web application work 1. API Call Flask Web Server Client App REST APIs DB 5. API Return 3. API interacts with DB, gets processes data 2. Web server directs API call to handler Your APIs/ Code 4. API returns data to Web Server
Flask basics server side from flask import Flask from flask_restful import Resource, Api from api.hello_world import HelloWorld app = Flask(__name__) #create Flask instance api = Api(app) #api router api.add_resource(HelloWorld, '/hi') #api 'endpoint'
Flask basics server side: API from flask import Flask def list_examples(): from flask_restful import Resource, Api return exec_get_all ('SELECT id, foo FROM example_table') from api.hello_world import HelloWorld class HelloWorld(Resource): def get(self): #Handles get request return dict(example.list_examples())
Flask basics client side import requests #HTTP library for get/ post ... url = http://locahost:8000/hi') #api 'endpoint' response = requests.get(url, params) #HTTP GET, url=endpoint
You now have two applications! First, you will need to start your server (server.py) THEN You will run your unit tests py m unittest v Two commands and TWO terminal windows!!
On the wire GET /hi HTTP/1.1 Host: localhost:5000 User-Agent: python-requests/2.22.0 Accept-Encoding: gzip, deflate Accept: */* Connection: keep-alive HTTP/1.0 200 OK Content-Type: application/json Content-Length: 29 Server: Werkzeug/1.0.1 Python/3.8.0 Date: Sat, 26 Sep 2020 16:21:28 GMT { "1": "hello, world!" } The request The response
On the wire GET /hi?a=1&b=2 HTTP/1.1 HTTP/1.0 200 OK Content-Type: application/json Content-Length: 29 Server: Werkzeug/1.0.1 Python/3.8.0 Date: Sat, 26 Sep 2020 16:21:28 GMT Host: localhost:5000 User-Agent: python-requests/2.22.0 Accept-Encoding: gzip, deflate Accept: */* Connection: keep-alive { "1": "hello, world!" } The request with params The response