Understanding Debugging in Programming
Debugging is a crucial aspect of programming to identify and fix errors that can cause program failures, hangs, or unexpected results. There are different types of errors such as compile errors, runtime errors, and logic errors, each requiring a different approach to resolve. Learning about the modes of operation in VBA - design time, runtime, and break mode - can help developers efficiently debug their code and ensure smooth execution. Utilizing breakpoints and debug mode are essential tools in the debugging process.
Download Presentation
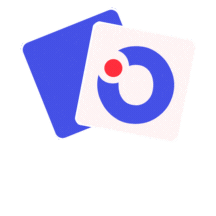
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
ITEC397 Macro Coding 07 DEBUGGING
Debugging 2 While you are writing a program always some bugs may exist that can cause a program failure, the program to hang, or simply unexpected results. Types of Errors Compile Errors Compile errors result from incorrectly constructed code. You may have used a property or method that does not exist on an object, or put in a For without a Next or an If without an End If. When you run this code, the compiler goes through the code first and checks for these types of errors. If any are found, the code will not be run but an error message will be displayed referring to the first error found. Note that there could be several compile errors in a procedure, but only the first one will be flagged. You might correct this error and rerun the procedure, and up comes another one! The real solution is to obey the rules of coding in the first place.
Debugging 3 Runtime Errors These are errors that occur when your program is running. You could, for example, try to open a file that does not exist, or attempt a division by zero. These would create an error message and halt execution of the program. They would not show up at compile time because they are not breaking any programming rules, but they will cause the code not to run.
Debugging 4 Logic Errors Logic errors occur when your application does not perform the way you intended. The code can be valid and run without producing any errors, but what happens is incorrect. These are by far the most difficult errors to locate. They can require a lot of painstaking searching to find, even using all the debugging tools at your disposal.
Design Time, Runtime, and Break Mode 5 When working on an application in VBA, there are three modes that you can be in: Design time: When you are working on the code for the application or designing a form. Runtime: When you run your code or your form. The title bar of the VBA screen will contain the word running, and at this point you can view code but you cannot change it.
Design Time, Runtime, and Break Mode 6 Break: If you press CTRL-BREAK during runtime, it will stop execution of your code. You can also insert a breakpoint by pressing the F9 Key. Pressing it again removes it. You can insert a breakpoint from the VBE menu by selecting Debug Toggle Breakpoint. A dialog will be displayed with the error message, Code execution has been interrupted as well as several buttons. Clicking the Debug button will take you into the code window.
Design Time, Runtime, and Break Mode 7 Break When you click Debug, you go into instant watch mode, also known as debug mode. You ll be able to see your code and the line it has stopped at will be highlighted in yellow. This is the line that is causing the problem. You can place your cursor on any variable that is in scope, and it will give you the value of it instantly. You can also move the point of execution by dragging the yellow arrow to the line of code to be executed.
Design Time, Runtime, and Break Mode 8 Running Selected Parts of Your Code If you know where the statement is that is causing an error, a single breakpoint will locate the problem. However, it s more likely that you will only have a rough idea of the area of code causing the problem. You can insert a breakpoint where you want to start checking the code and then single-step the procedure to see what each statement is doing (With pressing F8).
Design Time, Runtime, and Break Mode 9 Using Stop Statements Entering a Stop statement in your code is the same as entering a breakpoint, except it is in your code. When VBA encounters a Stop statement, it halts execution and switches to break mode. Although Stop statements act like breakpoints, they are not set or cleared in the same way. If you set breakpoints using F9, when you leave your project and then reload it, the breakpoints are all cleared. However, Stop statements form part of the code and are only cleared when you delete them or put a single quote (') character in front to change them into a comment. Make sure that once you have your code working properly, you remove all Stop statements.
Design Time, Runtime, and Break Mode 10 Single Stepping Single stepping allows you to execute one statement at a time. You can place your cursor on variables anywhere in the code to see the state of variables, and you can also use the Debug window to view values of variables. You can single-step by using Debug Step Into from the menu or pressing F8. You can also run the code to the position of the cursor. Click the mouse on a line of code and then press CTRL-F8. The code will only execute as far as where you have clicked the mouse. Note the cursor must be on an executable line of code, not a blank line. You can also step between individual statements if they are on the same line but separated by the : character. temp = 4: If temp = 3 Then Exit Sub
Design Time, Runtime, and Break Mode 11 Procedure Stepping If you have a subroutine or function that is being called, you may not wish to step through the whole procedure line by line. You may have already tested it and be satisfied with its performance. If you use F8 to step through (Single Step), you will be taken all through the subroutine s code one step at a time. This could be extremely time-consuming for something that you know already works. If you use SHIFT-F8 (Step Over), then your subroutine will be treated as a single statement but without stepping through it.
Design Time, Runtime, and Break Mode 12 Call Stack Dialog The Call Stack dialog displays a list of active procedure calls. That is, calls that have been started but not completed. You can display this dialog by using CTRL-L, but it is only available in Break mode. It can help you trace the operation of calls to procedures, especially if they are nested where one procedure then calls another procedure.
Design Time, Runtime, and Break Mode 13 The Debug Window The Debug window allows you to set a watch on specific variables or properties to see what values they hold as your program executes. This will aid you in debugging by allowing you to analyze a variable or property that has an incorrect value and determine where the problem is coming from. You enter the variable or expression that you wish to monitor in the Expression box. For example, if you have a variable x and you wish to keep track of the value of it, you enter x into the box. The context details are entered automatically. You can also specify whether you want the code to break when the value of the variable is True (nonzero) or when the value changes.
Design Time, Runtime, and Break Mode 14 Events That Can Cause Problems When Debugging Mouse Down Mouse down is the event fired off when the user presses any mouse button down. This is before the button comes back up. If you break the code at this point, you will not get a mouse up event. A mouse up event occurs only when you press the mouse button again and release it.
Design Time, Runtime, and Break Mode 15 Events That Can Cause Problems When Debugging Got Focus/Lost Focus This event occurs when a user clicks your form or a particular control on the form to get the focus on that control. If you break the code at this point, you may get inconsistent results. Whether you do or not depends on whether your control had the focus at the point that the BREAK key was pressed.
Design Time, Runtime, and Break Mode 16 Using Message Boxes in Debugging Even you have seen many useful ways and tools for debugging, message boxes are still very useful for letting you know what is going on. They can display the value of a variable or even several variables by concatenating them together. They are extremely useful when you have a large procedure and you do not know the region the bug is in. For example, a large piece of code appears to run but hangs and will not respond to CTRL-BREAK. Perhaps it ran perfectly up to now but has hit certain circumstances that cause it to hang.
Design Time, Runtime, and Break Mode 17 Using Message Boxes in Debugging The question is, how far into the code do you get before the issue occurs? You could try stepping through it, but this is very time-consuming if it is a large procedure. Placing message boxes at strategic points throughout the code will give you an idea of where it stops. Make sure all the message boxes display something meaningful, such as Ok1, Ok2, and Ok3. You can then see what stages the program has covered before it hangs. Clear all the extra message boxes out once you have found the bug!
Avoiding Bugs 18 Careful designing and planning of the application is important and will help reduce bugs. Often it is best to break the application down into smaller component parts that are easier to code and test. It is easier to think of a small portion of code than to try to tackle an entire project in one thought. Make sure you document and comment your code using the single quote (') character.
Avoiding Bugs 19 The comments will turn green within your code. If the application is complicated, it is often difficult to go back even after just a few days to determine what the code is doing. Even professional programmers find if they go back to code they wrote a few months ago, they have difficulty understanding what the code was doing. Comments may provide the help needed. Also, if a programmer leaves an organization, it can be difficult for a new or different programmer to pick up where the former employee left off without any documentation about the intent of the code.
Avoiding Bugs 20 It is important to know what all your variables represent and what each function does. Once your application starts growing and begins to get complicated, its documentation important. Without such documentation, you will need a good memory to keep track of what every variable means! becomes more