Array Indices: Starting at 0 or 1?
The debate over whether array indices should start at 0 or 1 continues. A compromise of 0.5 was rejected, leading to discussions on JavaScript syntax, function definitions, and iterator implementations in programming. Explore the intricacies of array indexing and programming practices.
Uploaded on Sep 10, 2024 | 0 Views
Download Presentation
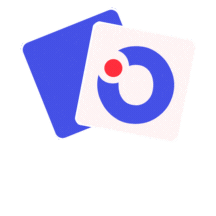
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
CSE305 Programming Languages Daniel R. Schlegel April 25, 2011 Should array indices start at 0 or 1? My compromise of 0.5 was rejected without, I thought, proper consideration. (Stan Kelly-Bootle)
JavaScript Syntax Is this valid? int fact(int n) { if (n === 0) { return 1; } else return n * fact(n - 1); }
JavaScript Syntax Is this valid? int fact(int n) { if (n === 0) { return 1; } else return n * fact(n - 1); } NO! function fact(n) { if (n === 0) { return 1; } else return n * fact(n - 1); }
JavaScript Syntax What does this return? function array(f, g) { //f and g are functions return [f(), g()]; }
JavaScript Syntax What does this return? function array(f, g) { //f and g are functions return [f(), g()]; } What about this? function array(f, g) { //f and g are functions return [f, g]; }
Assignment 5, Part 1 iterator ireverse(array a) { int n = a.length -1; for (int i=0; i<=n; i++) { yield a[n-i]; } } Q: When you call ireverse(a) does it give you all the answers at once? void main { } foreach x in ireverse(a) { foreach y in ireverse (b) { sum = sum + x*y; } }
Assignment 5, Part 1 iterator ireverse(array a) { int n = a.length -1; for (int i=0; i<=n; i++) { yield a[n-i]; } } Q: When you call ireverse(a) does it give you all the answers at once? NO! void main { } foreach x in ireverse(a) { foreach y in ireverse (b) { sum = sum + x*y; } } What does this do?
Assignment 5, Part 1 iterator ireverse(array a) { int n = a.length -1; for (int i=0; i<=n; i++) { yield a[n-i]; } } Q: When you call ireverse(a) does it give you all the answers at once? NO! void main { } foreach x in ireverse(a) { foreach y in ireverse (b) { sum = sum + x*y; } } What does this do? 1. Get the next value of ireverse(a). 2. Set x to it.
We need to simulate this behavior! void main(){ List l1, l2; l2 := nil; foreach x in elements(l1) do{ l2 := cons(x, l2); } print l2; } void main(){ List l1, l2; void thk(int x) { l2 := cons(x, l2); } l2 := nil; elements(l1, thk); print l2; } iterator int elements(List l){ while(l != nil) { yield l.val; l := l.next; } } void elements(List l, void thk(int)){ while (l != nil) do { thk(l.val); l := l.next; } }
Lazy Evaluation What s the advantage?
Lazy Evaluation What s the advantage? Doesn t find a result until that result is needed! We can use functions that generate infinite lists. Does JavaScript have it built in?
Lazy Evaluation What s the advantage? Doesn t find a result until that result is needed! We can use functions that generate infinite lists. Does JavaScript have it built in? No! Can we simulate it? If yes, how?
Lazy Evaluation What s the advantage? Doesn t find a result until that result is needed! We can use functions that generate infinite lists. Does JavaScript have it built in? No! Can we simulate it? If yes, how? Yes, using higher order functions.
How we usually do things function f(x) { return [x+1, x*2, x*x]; } Short running tasks f(100); //[101, 200, 10000] Okay, so these are short running tasks but what if we didn t need all of this?
What about this function f(x) { return [longtask(x), longertask(x), reallylongtask(x)]; } function g(n) { print(f(100)[n]); //Some value } Is there a problem with this? We never use f(100)[0] or f(100)[2], but they are still evaluated!
A lazy version function f(x) { thk1() = longtask(x); thk2() = longertask(x); thk3() = reallylongtask(x); return [thk1, thk2, thk3]; } function g(n) { print(f(100)[n]()); //Some value }
So now extend this to infinite lists numsfrom = function(n){ return cons(n, numsfrom(n+1)); }; What happens when we run this?
So now extend this to infinite lists numsfrom = function(n){ var thk = function(){return numsfrom(n+1);}; return [n,thk]; };
Final Notes JIVE Survey Today is the last recitation Good luck on the assignment and final! Also, I m teaching CSE111 this summer If you know anyone who needs to take it, let them know!