Understanding Function Overloading and this Pointer in C++
Explore the concept of function overloading in C++, allowing multiple functions with the same name but different parameters. Learn about the this pointer in object-oriented programming, which holds the memory address of the current object using a function. See examples of how to implement these concepts in a class with the Rectangle program. Discover the role of friend functions in C++ and how they can access private members of a class, distinguishing them from regular functions.
Uploaded on Sep 21, 2024 | 0 Views
Download Presentation
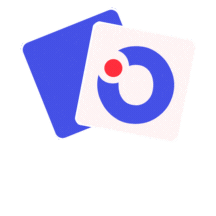
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Function Overloading Two or more functions can have the same name but different parameters Example: int max(int a, int b) { if (a>= b) return a; else return b; } float max(float a, float b) { if (a>= b) return a; else return b; }
this pointer The this pointer holds the memory address of the current object that is using the function The this pointer is automatically supplied when you call a non-static member function of a class
this pointer #include <iostream.h> class Rectangle { public: Rectangle(); ~Rectangle(); void SetLength(int length) { this->itsLength = length; } int GetLength() const { return this->itsLength; } void SetWidth(int width) { itsWidth = width; }
this pointer int GetWidth() const { return itsWidth; } private: int itsLength; int itsWidth; }; Rectangle::Rectangle() { itsWidth = 5; itsLength = 10; } Rectangle::~Rectangle() { }
this pointer int main() { Rectangle theRect; cout << "theRect is " << theRect.GetLength() << " feet long.\n"; cout << "theRect is " << theRect.GetWidth() << " feet wide.\n"; theRect.SetLength(20); theRect.SetWidth(10); cout << "theRect is " << theRect.GetLength()<< " feet long.\n"; cout << "theRect is " << theRect.GetWidth()<< " feet wide.\n"; return 0; } Output: theRect is 10 feet long. theRect is 5 feet wide. theRect is 20 feet long. theRect is 10 feet wide.
Who is a friend ? A friend is a one who has access to all your PRIVATE Stuff
Friend function C++ friend functions are special functions which can access the private members of a class.
How is it different from a normal function? A member is access through the object Ex : sample object; object.getdata( ); Whereas a friend function requires object to be passed by value or by reference as a parameter Ex : sample object; getdata(object) ;
Friend Functions Example: class myclass { int a, b; public: friend int sum(myclass x); void set_val(int i, int j); }; Syntax: class class_name { //class definition public: friend rdt fun_name(formal parameters); };
Code Snippet class demo { int x ; public : demo(int xxx) { x = xxx; } friend void display(demo) ; }; void display(demo d1) { cout<<dd1.x; } int main( ) { demo d(5); display(d); return 0; }
Friend function Friend functions have the following properties: 1) Friend of the class can be member of some other class. 2) Friend of one class can be friend of another class or all the classes in one program, such a friend is known as GLOBAL FRIEND. 3) Friend can access the private or protected members of the class in which they are declared to be friend, but they can use the members for a specific object. 4) Friends are non-members hence do not get this pointer. 5) Friends, can be friend of more than one class, hence they can be used for message passing between the classes. 6) Friend can be declared anywhere (in public, protected or private section) in the class.
Program for Implementation Pgm to create a class ACCOUNTS with function read() to input sales and purchase details. Create a Friend function to print total tax to pay. Assume 4% of profit is tax.