Understanding Program Execution in Linux
Explore the process of program execution in Linux, including details on the ELF executable file format, creating executable files, static vs. dynamic linking, and the impact of linking on executable sizes. Learn about compilation, linking, and the differences between static and dynamic linking in the Linux environment.
Download Presentation
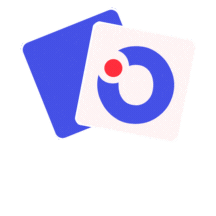
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Program Execution in Linux David Ferry, Chris Gill, Brian Kocoloski, James Orr, Marion Sudvarg CSE 422S - Operating Systems Organization Washington University in St. Louis St. Louis, MO 63143 1
Executable File Format The current binary file format is called ELF - Executable and Linking Format ELF header Word size, byte ordering, file type (.o, exec, .so), machine type, etc. .text section Code .rodata section Read only data: jump tables, .data section Initialized global variables .bss section Uninitialized global variables or ones initialized with 0 Block Started by Symbol Has section header but occupies no space Other sections added by gcc ELF header .text .rodata .data .bss .symtab .rel.txt .rel.data .debug Section header table 2 CSE 422S Operating Systems Organization
Creating an Executable File //Source code #include <stdio.h> Two stages: Compilation Linking int foo = 20; int main( int argc, char* argv[]){ printf( Hello, world!\n ); return 0; } The compiler translates source code to machine code (via the assembler) Compiler Relocatable Object file: 00000000 D foo 00000000 T main U puts The linker connects binary files to libraries to create an executable Linker Executable file 3 CSE 422S Operating Systems Organization
Static vs. Dynamic Linking Static linking required code and data is copied into executable at compile time Dynamic linking required code and data is linked to executable at runtime my_program.o Static: Dynamic: Program code my_program.o libc.so Program code Program Data Library Code/Data Library Code/Data Program Data 4 CSE 422S Operating Systems Organization
Static Linking With multiple programs, what happens to the size of the executables? Program code Program Data Library Code 5 CSE 422S Operating Systems Organization
Static Linking With multiple programs, what happens to the size of the executables? Program code Program code Program code Program code Program Data Program Data Program Data Program Data Library Code Library Code Library Code Library Code Program code Program code Program code Program Data Program Data Program Data Library Code Library Code Library Code 6 CSE 422S Operating Systems Organization
Dynamic Linking With multiple programs, what happens to the size of the executables? Program code Program code Program code Program code Program Data Program Data Program Data Program Data Library Code Program code Program code Program code Program Data Program Data Program Data 7 CSE 422S Operating Systems Organization
Running a Statically Linked Program All functions and data needed by the process space are linked as the last step of the compiler Stack Static library A code/data Static library B code/data Only that code/data from the library needed by the program are loaded into virtual memory Heap .bss .data .text 8 CSE 422S Operating Systems Organization
Running a Statically Linked Program A statically linked program is entirely self-contained 1. An existing process is fork()ed to get a new process address space 2. execve() reads program into memory 3. The new process starts executing at _start() in the C runtime (added automatically by the linker), which sets up environment 4. The C runtime eventually calls the program s main() function 5. After main() returns, C runtime does some cleanup 9 CSE 422S Operating Systems Organization
Running a Dynamically Linked Program Some functions and data do not exist in process space at runtime Stack Memory Map Segment (s) (added at runtime by linker) The dynamic linker (called ld) maps these into the memory map segment on-demand Heap .bss .data .text 10 CSE 422S Operating Systems Organization
Linking at Runtime Shared libraries are with Position Independent Code which allows for branch and jump instructions to addresses relative to other code segments Global Offset Table (GOT) (in .data) Used to resolve locations of library objects (functions and global variables) All references to variables in shared libraries are replaced with references to the GOT Procedure Linkage Table (PLT) (in .text) All function calls to shared libraries are replaced by stub functions that query the PLT at runtime for the address of the function Calls the dynamic linker to resolve locations of library functions if unknown, points to GOT entry if known 11 CSE 422S Operating Systems Organization
Linking at Runtime At compile time: The dynamic linker (ld) is embedded in program Addresses of dynamic functions are replaced in GOT with calls to the linker At runtime the linker does lazy-binding: The program runs as normal until it encounters an unresolved function Execution jumps to the linker The linker maps the shared library into the process s address space and replaces the call to the linker in GOT with the resolved address This is called lazy update of GOT 12 CSE 422S Operating Systems Organization
Runtime Linker Implementation //Source code #include <stdio.h> int foo = 20; Stack int main( int argc, char* argv[]){ printf( Hello, world!\n ); return 0; } Procedure Link Table (PLT) Heap GOT entry for printf() .bss Global Offset Table (GOT) .data dynamic linker (ld) .text 13 CSE 422S Operating Systems Organization
Runtime Linker Implementation //Source code #include <stdio.h> int foo = 20; Stack int main( int argc, char* argv[]){ printf( Hello, world!\n ); return 0; } Library with printf() function Procedure Link Table (PLT) Heap GOT entry for printf() .bss Global Offset Table (GOT) .data library printf() .text 14 CSE 422S Operating Systems Organization
Static vs. Dynamic Linking Tradeoffs Static: Does not need to look up libraries at runtime Does not need extra PLT indirection Consumes more memory with copies of each library in every program Dynamic: Less disk space/memory (7K vs 571K for hello world) Shared libraries already in memory and in hot cache Incurs lookup and indirection overheads 15 CSE 422S Operating Systems Organization
Binary File Utilities Not covered in CSE 361: nm prints symbol table objdump prints all binary data readelf prints ELF data pmap prints memory map of a running process ldd prints dynamic library dependencies of a binary strip strips symbol data from a binary 16 CSE 422S Operating Systems Organization
GNU Debugger (gdb) Allows you to debug a program while it executes Parent process (gdb) forks to launch a program Uses ptrace system call to observe execution of child program Can set breakpoints to pause execution of child at specific instruction addresses Can set watchpoints to monitor changes to particular memory locations 17 CSE 422S Operating Systems Organization