Understanding Programming Language Features
Exploring syntax and semantics in programming languages, differences between interpretation and compilation, advantages and disadvantages of interpreters and compilers, and the concepts of static and dynamic behaviors in programming. Discover how different languages handle syntax and semantics, and the impact of interpretation and compilation on code execution.
Download Presentation
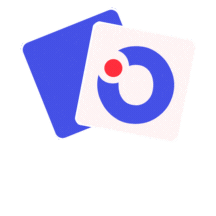
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Some Basic Programming Language Features CMPT 383
Syntax Syntax Python for i in range(seq): val[i] += 1 What the language looks like when you read the source code These all do essentially the same thing, but their syntax differs In practice, syntax is typically precisely specified using grammars C++ for(int i = 0; i < seq.size(); i++) { val[i]++; } Racket (map (lambda (n) (+ n 1)) seq)
Modifies seq in- place (i.e. no copy is made). Semantics Semantics C++ for(int i = 0; i < seq.size(); i++) { val[i]++; } What the language means Logic and mathematics can be used to give expressions clear meaning However, this is quite hard to do as compared to using grammars for syntax, and so in practice we usually use carefully written English to specify semantics Makes a copy of seq. Racket (map (lambda (n) (+ n 1)) seq)
Interpretation vs. Compiling A compiled language processes converts the entire program into machine language Then, after the compilation is successful, you run the resulting machine language An interpreted language process each source code statement one at a time, executing it immediately after its translated Interpreters intermix running the program, and converting it into machine language Many languages are a hybrid of compiled and interpreted. For example, Python compiles to bytecode and then interprets that bytecode.
Interpretation vs. Compiling Interpreters Compilers Pro: easy to test small bits of code Pro: can have access to the source code at run time Pro: compared to a compiler, often easier to implement Con: usually slower than a compiler since conversion to machine language happens at run time Pro: usually faster than an interpreter since conversion to machine language done before running Pro: can catch some errors before running the program Con: more work to run small pieces of code Con: the compiling phase can be get complex (and slow)
Static vs. Dynamic Static generally refers to things done to a program before it runs E.g. anything a compiler does to a program is considered static A defined function and the types of its parameters can be determined at compile-time In most modern languages, the scope of a variable can be determined statically (i.e. at compile-time) Dynamic generally refers to things done to a program while it is running The value of most variables is not known until the program runs, e.g. x = input('Age? ) print(x)
Static Typing vs Dynamic Typing Static typing refers to type information in a program that can be determined by the compiler C++, Haskell, and Go are (mostly) statically typed Their compilers can catch many kinds of type errors before running Dynamic typing refers to type information in a program that can be determined at run-time Python and Racket are dynamically typed Type errors found at run-time