Working with Arrays: Definitions and Examples
In this content, you will find detailed explanations and examples on defining and working with 1D and 2D arrays in programming languages like C++. It covers creating arrays, populating them with values, and working with nested loops. The content also includes pseudocode examples for a better understanding of nested loop structures.
Download Presentation
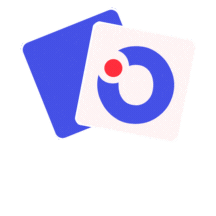
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Arrays Arrays 1D & 2D Arrays
Defining a 1D array Defining a 1D array CREATE array nums [size] Ps
C++ C++ - - Define a 1D Array Define a 1D Array <type><name>[size]; For example: int size = 10 int grid[size]
Defining a 2D array Defining a 2D array CREATE array nums [numRows][numColumns] Ps
C++ C++ - - Define a 2D Array Define a 2D Array <type><name>[<row_size>][<column_size>]; For example: int grid[10][20];
Working with 2D arrays Working with 2D arrays Usually involves nested loops, as shown below. Problem Statement: Create an array of 4 rows and 5 columns. Populate the array with the numbers 1-20. --------------------------------------------------------------------- Create array grid[4][5] count = 1 FOR each element in a row FOR each element in a column grid[row][col] = count count = count + 1 END INNER FOR END FOR
int count = 1; int grid[4][5]; //getting the rows in the array for (int row = 0; row < 4; row++) { //populate the values in the array (row) we're currently on from the outer loop for (int column = 0; column < 5; column++) { grid[row][column] = count; count++; } } //for each array in the grid (represented by rows) for (int row = 0; row < 4; row++) { for (int column = 0; column < 5; column++) { //for each element in the array (row) we're currently on cout << grid[row][column] << ","; if(grid[row][column] % 5 == 0) { cout << endl; } } }
Pseudocode Nested for Loop Example BEGIN MAIN CREATE maxRows = 10, row, star FOR (row = 1, row < maxRows, row = row + 1) FOR (star = 1, star <= row, star = star + 1) PRINT * ENDinnerFOR PRINTLINE () ENDouterFOR END MAIN Ps
Nested for Loop Example int maxRows = 10; for (int row = 1; row <= maxRows; row++) { for (int star = 1; star <= row; star++) { cout << "*"; } cout << endl; }