Understanding Type Conversions in ANSI C Programming
This content delves into type conversions in ANSI C programming, covering automatic and explicit conversions, unary and binary conversions, with examples and explanations. It discusses how different data types are handled in arithmetic operations, including the promotion of lower types to higher types. It provides insights into handling expressions containing variables and constants of different types, along with the use of casts for explicit type conversions.
Download Presentation
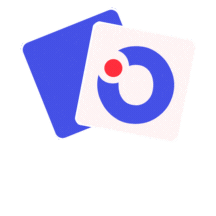
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
COM101B LECTURE 5: TYPE CONVERSIONS ASST. PROF. DR. HACER YALIM KELE REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992
TYPE CONVERSIONS An expression may contain variables and constants of different types. We will discuss how such expressions are evaluated REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992
AUTOMATIC TYPE CONVERSIONS ANSI C performs all arithmetic operations with just six data types: int, unsigned int, long int, float, double, long double Any operand of the type char or short is implicitly converted to int before the operation Conversions of char and short to int are called automatic unary conversions. REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992
AUTOMATIC BINARY TYPE CONVERSIONS ABT is applied to both operands Carried out after automatic unary conversions In general: if a binary operator has operands of different types, the lower type is promoted tp the higher type before the operation proceeds. The result is of the higher type. The sequence of ops: char/short > int > long > float > double > long double REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992
EXAMPLE Expr: ( c / u l ) + s * f c: char; u: unsigned int; l : long int; s: short int; f: float REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992
EXPLICIT TYPE CONVERSION C provides a special construct called a cast for this purpose: (cast-type) expression where, cast-type is one of the C data types. Exaple: (int) 12.8 is 12 (int) 12.8 * 3.1 is 12 * 3.1 -> 37.2 REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992
A typical usage of explicit type casting: Forcing an integer division to a floating-point division Example: (float) 3 / 2 is 3.0/2 -> 1.5 REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992
TYPE CONV. IN ASSIGNMENTS When variables of different types are mixed in an assignment expression, the type of the value of the expression on the right side of the assignment operator is automatically converted to the type of the variable on the left hand side of the operator. The type of the resultant expr. is that of the left operand. REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992
SIMPLE MACROS Macros are constants that are replaced by their defined values in the pre- compilation stage. We can define macros as follows: #define MAX 365 Where the macro const MAX appears in the code is replaced by the value 365 in the code. REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992
MACRO EXAMPLE #include <stdio.h> #define PI 3.14 // MACRO const definition int main() { float r; scanf( %f , &r); printf( Area: %f\n , PI*r*r); return 0; } REFERENCE: PROGRAMMING IN ANSI C , KUMAR & AGRAWAL, WEST PUBLISHING CO., 1992