Understanding Structs in C++ Programming
Learn about structs in C++ programming, how to define and work with them, store and manipulate data using structs, and the differences with functions. Explore examples of working with struct variables, different structs with the same members, and utilizing functions with structs in C++.
Download Presentation
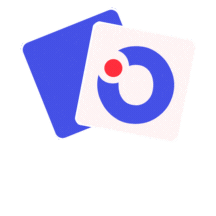
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Structs COMP 11
Example: Celebrity Heights #name feet inches Task: Write a program that reads in a list of celebrity heights from a file and outputs ***insert generic statistic here***. Danny_Devito 5 0 Oprah_Winfrey 5 7 Q: How should we store the data? A: 3 arrays of same length Tom_Cruise 5 7 Nicole_Kidman 5 10.5 A: 1 array of packaged data Bernie_Mac 6 2.5
Structs A struct is a package used to hold different types of data. - can be passed around like any other variable - we can access members of a struct individually - need to define the struct s type first Components of a struct definition: 1. struct keyword struct Celeb { string name; int feet; float inches; }; } 2. Name (typically capitalized) 3. Member declarations (in { }) 4. Semicolon after { }
Working with struct variables struct Celeb { string name; int feet; float inches; }; Nicole_Kidman one_celeb 5 int main() { Celeb one_celeb; 10.5 *bookkeeping* one_celeb.name = "Nicole_Kidman"; one_celeb.feet = 5; one_celeb.inches = 10.5; return value return address argv cout << "Name: " << one_celeb.name << endl; cout << "Height: " << one_celeb.feet << " feet, "; cout << one_celeb.inches << " inches" << endl; argc }
Different structs, same members? struct Celeb { string name; int feet; float inches; }; struct Professor { string name; string courses[10]; int age; }; Totally fine! Member names are local to a given struct. int main() { Celeb one_celeb; Professor richard; one_celeb.name = "Nicole_Kidman"; richard.name = "Richard"; richard.courses[0] = "COMP 11"; }
Structs, Functions struct Celeb { string name; int feet; float inches; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; one_celeb.name = "Nicole_Kidman"; one_celeb.feet = 5; one_celeb.inches = 10.5; Celeb grow(Celeb c) { c.feet += 100; return c; } one_celeb = grow(one_celeb); cout << one_celeb.name << " is now "; cout << one_celeb.feet << " feet tall!"; cout << endl; return 0; }
Structs, Functions struct Celeb { string name; int feet; float inches; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; one_celeb.name = "Nicole_Kidman"; one_celeb.feet = 5; one_celeb.inches = 10.5; Celeb grow(Celeb c) { c.feet += 100; return c; } This modifies a copy of the struct passed as an argument, not the original. one_celeb = grow(one_celeb); cout << one_celeb.name << " is now "; cout << one_celeb.feet << " feet tall!"; cout << endl; return 0; }
Structs, Functions struct Celeb { string name; int feet; float inches; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; one_celeb.name = "Nicole_Kidman"; one_celeb.feet = 5; one_celeb.inches = 10.5; Celeb grow(Celeb c) { c.feet += 100; return c; } Nicole_Kidman c 5 10.5 one_celeb = grow(one_celeb); cout << one_celeb.name << " is now "; cout << one_celeb.feet << " feet tall!"; cout << endl; return 0; Nicole_Kidman one_celeb 5 10.5 }
Structs, Functions struct Celeb { string name; int feet; float inches; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; one_celeb.name = "Nicole_Kidman"; one_celeb.feet = 5; one_celeb.inches = 10.5; Celeb grow(Celeb c) { c.feet += 100; return c; } Nicole_Kidman 5 10.5 one_celeb = grow(one_celeb); cout << one_celeb.name << " is now "; cout << one_celeb.feet << " feet tall!"; cout << endl; return 0; Nicole_Kidman 5 10.5 }
Structs, Functions struct Celeb { string name; int feet; float inches; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; one_celeb.name = "Nicole_Kidman"; one_celeb.feet = 5; one_celeb.inches = 10.5; Celeb grow(Celeb c) { c.feet += 100; return c; } Nicole_Kidman 105 10.5 one_celeb = grow(one_celeb); cout << one_celeb.name << " is now "; cout << one_celeb.feet << " feet tall!"; cout << endl; return 0; Nicole_Kidman 5 10.5 }
Structs, Functions struct Celeb { string name; int feet; float inches; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; one_celeb.name = "Nicole_Kidman"; one_celeb.feet = 5; one_celeb.inches = 10.5; Celeb grow(Celeb c) { c.feet += 100; return c; } Nicole_Kidman 105 10.5 one_celeb = grow(one_celeb); cout << one_celeb.name << " is now "; cout << one_celeb.feet << " feet tall!"; cout << endl; return 0; Nicole_Kidman 5 10.5 }
Structs, Functions struct Celeb { string name; int feet; float inches; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; one_celeb.name = "Nicole_Kidman"; one_celeb.feet = 5; one_celeb.inches = 10.5; Celeb grow(Celeb c) { c.feet += 100; return c; } one_celeb = grow(one_celeb); cout << one_celeb.name << " is now "; cout << one_celeb.feet << " feet tall!"; cout << endl; return 0; Nicole_Kidman 105 10.5 }
Exercise: Structs, Functions struct Bulb { int watts; int lumens; }; 1. Which part of this code defines a struct type? Bulb dim(Bulb b) { Bulb c = b; c.watts += 10; return b; } 1. How many members does the Bulb type have? 1. What does this code print? int main() { Bulb lightA; lightA.watts = 20; Bulb lightB = dim(lightA) cout << lightB.watts << " " << lightB.lumens; return 0; }
Exercise: Structs, Functions struct Bulb { int watts; int lumens; }; Two members 1. Which part of this code defines a struct type? Bulb dim(Bulb b) { Bulb c = b; c.watts += 10; return b; } 1. How many members does the Bulb type have? 1. What does this code print? int main() { Bulb lightA; lightA.watts = 20; Bulb lightB = dim(lightA) cout << lightB.watts << " " << lightB.lumens; return 0; } 20 <???>
So many more copies of array elements... Structs, Functions struct Celeb { string name; int feet; float inches; string every_movie[100]; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; //Initialize one_celeb Nicole_Kidman Structs are passed-by-value: every member is copied when passed to/returned from a function. 5 10.5 movies[0] movies[1] Lots of array elements... one_celeb = grow(one_celeb); Q: What happens on the stack when we pass this struct as an argument? //Do stuff with one_celeb return 0; } A: Every array element is copied! movies[99]
Structs, Functions struct Celeb { string name; int feet; float inches; string every_movie[100]; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; //Initialize one_celeb Nicole_Kidman Structs are passed-by-value: every member is copied when passed to/returned from a function. 5 10.5 movies[0] movies[1] Lots of array elements... one_celeb = grow(one_celeb); //Do stuff with one_celeb return 0; } movies[99]
Structs, Functions, Pointers struct Celeb { string name; int feet; float inches; string every_movie[100]; }; Celeb grow(Celeb c); int main() { Celeb one_celeb; //Initialize one_celeb Nicole_Kidman Structs are passed-by-value: every member is copied when passed to/returned from a function. 5 10.5 movies[0] Pass a struct pointer to avoid excessive copying! movies[1] Lots of array elements... one_celeb = grow(one_celeb); //Do stuff with one_celeb return 0; } movies[99]
Structs, Functions, Pointers c celeb_p struct Celeb { string name; int feet; float inches; string every_movie[100]; }; void grow(Celeb *c); int main() { Celeb one_celeb; //Initialize one_celeb Celeb *celeb_p = &one_celeb; grow(celeb_p); Nicole_Kidman Structs are passed-by-value: every member is copied when passed to/returned from a function. 5 10.5 movies[0] Pass a struct pointer to avoid excessive copying! movies[1] Lots of array elements... void grow(Celeb *c) { (*c).feet += 100; } //Do stuff with one_celeb return 0; } movies[99]
Structs, Functions, Pointers c celeb_p struct Celeb { string name; int feet; float inches; string every_movie[100]; }; void grow(Celeb *c); int main() { Celeb one_celeb; //Initialize one_celeb Celeb *celeb_p = &one_celeb; grow(celeb_p); Nicole_Kidman Structs are passed-by-value: every member is copied when passed to/returned from a function. 105 10.5 movies[0] Pass a struct pointer to avoid excessive copying! movies[1] Lots of array elements... void grow(Celeb *c) { (*c).feet += 100; } //Do stuff with one_celeb return 0; } movies[99]
Structs, Functions, Pointers celeb_p struct Celeb { string name; int feet; float inches; string every_movie[100]; }; void grow(Celeb *c); int main() { Celeb one_celeb; //Initialize one_celeb Celeb *celeb_p = &one_celeb; grow(celeb_p); Nicole_Kidman Structs are passed-by-value: every member is copied when passed to/returned from a function. 105 10.5 movies[0] Pass a struct pointer to avoid excessive copying! movies[1] Lots of array elements... void grow(Celeb *c) { c->feet += 100; } //Do stuff with one_celeb return 0; } Access member mem of struct pointer p with either (*p).mem OR p->mem. movies[99]
Demo: structs, functions, and pointers Task: Write a program that reads in a list of celebrity heights from a file and outputs ***insert generic statistic here***. The file will start with some integer n, then the following n lines will each have the height info for one celebrity.