Understanding Python Functions Through Examples
This content provides insight into Python functions through code examples. It covers topics like checking for square grids and concatenating elements in a list. The explanation with images simplifies complex concepts and helps in understanding these functions effectively.
Download Presentation
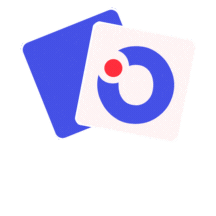
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
CSc CSc 120 120 Introduction to Computer Programming II Introduction to Computer Programming II CODE EXAMPLES 01
def grid_is_square(arglist): length= len(arglist) i = 0 for i in range(length): x = 0 if arglist[i][x] == arglist[x][i]: return True elif arglist[i][x] != arglist[x][i]: return False 3
if BoolExpr: x = True else: x = False def grid_is_square(arglist): length= len(arglist) i = 0 for i in range(length): x = 0 if arglist[i][x] == arglist[x][i]: return True elif arglist[i][x] != arglist[x][i]: return False is equivalent to: x = BoolExpr 4
def grid_is_square(arglist): length= len(arglist) i = 0 for i in range(length): x = 0 return arglist[i][x] == arglist[x][i] Simplifying out the Boolean expression makes the bug in the code easier to see 5
def grid_is_square(arglist): length= len(arglist) i = 0 for i in range(length): x = 0 return arglist[i][x] == arglist[x][i] this initialization has no effect 6
def grid_is_square(arglist): length= len(arglist) i = 0 for i in range(length): x = 0 return arglist[i][x] == arglist[x][i] we don't need this 7
def grid_is_square(arglist): length= len(arglist) for i in range(length): return arglist[i][0] == arglist[0][i] returns on the first iteration 8
def concat_elements(list, startpos, stoppos): result = "" if (startpos < 0): startpos = 0 if (stoppos > len(list)): stoppos = len(list) if (startpos > stoppos): return '' a = list[startpos:stoppos+1] result = ''.join(a) return result 10
def concat_elements(list, startpos, stoppos): result = "" if (startpos < 0): startpos = 0 if (stoppos > len(list)): stoppos = len(list) if (startpos > stoppos): return '' a = list[startpos:stoppos+1] result = ''.join(a) startpos = max(startpos, 0) stoppos = min(stoppos, len(list)) return result 11
def concat_elements(list, startpos, stoppos): result = "" startpos = max(startpos,0) stoppos = min(stoppos, len(list))) This is not really needed if (startpos > stoppos): return '' a = list[startpos:stoppos+1] result = ''.join(a) return result 12
def concat_elements(list, startpos, stoppos): new = "" for i in range (max(0, startpos), min( len(list), stoppos + 1)): new += list [i] return new 13