Understanding Makefiles and Automated Compilation in C++ Projects
Makefiles are essential tools that automate the compilation process in C++ projects, saving time and ensuring only necessary files are recompiled. They help manage dependencies efficiently, making development more streamlined and organized. This article discusses the importance of Makefiles, their structure, and how they improve the development workflow.
Download Presentation
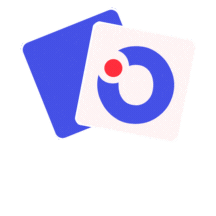
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
~/CSCE221/sampleCode/MakeFiles> Where code is located
GET ONTO CSE.COMPUTE.EDU NOW!!! GET ONTO CSE.COMPUTE.EDU NOW!!! Don t forget to VPN in!! GET ONTO CSE.COMPUTE.EDU NOW!!! Don t forget to VPN in!! GET ONTO CSE.COMPUTE.EDU NOW!!! Don t forget to VPN in!!
CSCE-221 Makefile Introduction Emil Thomas 01/17/19 (Edited by Zhipei Yan, Fall 2019) Based on slides by Prof. Jeremy Gibson(UMB) and Prof. Shawn Lupoli (TAMU)
How do you organize source files? IDE: Visual Studio CodeBlocks Eclipse Xcode Others Makefile CMake Manually?
Make Overview make is a program that automates the compilation of programs whose files are dependent on each other A program typically consists of several files, and a programmer usually only works on a few of them at a time Typically vast majority of files remain unchanged and thus do not need to be recompiled 5
Why using make and Makefile ? automated way of compiling make will only recompile the files that need to be updated (files that depend on modified files), - faster & efficient than recompiling the whole program each time make looks at the timestamps of source files (*.cpp, *.h) that are required to generate an object file (*.o) If a source is newer then the object file, the object file needs to be recompiled Likewise if an object file is newer than the executable it needs to be re-linked can clean up mess from compiling 6
Makefile Example & Demonstration Consider a cpp project with 3 files : driver.cpp Airplane.cpp Airplane.h Our objective is to create a Makefile, with the end goal of a executable named output.out Source files setup (look at includes) Airplane.h Airplane.cpp driver.cpp #ifndef AIRPLANE_H_ #define AIRPLANE_H_ #include <iostream> #include <string> #include <iostream> #include <string> #include <string> #include <iostream> using namespace std; using namespace std; using namespace std; #include "Airplane.h" #include "Airplane.h"
Compilation process Airplane.o: Airplane.cpp Airplane.h g++ -Wall -c Airplane.cpp Airplane.cpp Airplane.o Airplane.h output.out driver.cpp driver.o output.out: Airplane.o driver.o g++ -Wall Airplane.o driver.o o output.out
Makefile Rule Dependency List The files that are required to create the object file. In this case Airplane.cpp and Airplane.h Target The file to create. In this case an object file: Airplane.o Airplane.o: Airplane.cpp Airplane.h g++ -Wall -c Airplane.cpp <TAB> Action(s) Used to signal what follows as an action What needs to be done to create the target. In this case it is the separate compilation of Airplane.cpp 9
Makefile Structure A Makefile is a list of rules of what files are required to generate an object or an executable file Each rule consists of 4 parts: Target: the name of the object or executable to create Usually the final executable name and object files Dependency List: the list of files that the target is dependent upon For an object file target : the corresponding .cpp file and any user defined #included header files in it. (Don t use system header files) TAB: used to set off an action Action(s): a list of actions / linux system commands to take in order to create the target (i.e. g++ ) 10
Makefile (All the project files should be in the same directory as the Makefile) output.out: driver.o Airplane.o g++ Wall -std=c++11 driver.o Airplane.o -o output.out driver.o: driver.cpp Airplane.h g++ Wall -std=c++11 -c driver.cpp Airplane.o: Airplane.cpp Airplane.h g++ Wall -std=c++11 -c Airplane.cpp # -Wall had to come 1st before std=c++11 (for some reason in compute) # -Wall enables all compiler's warning messages. This option should always # be used, in order to generate better code. # -f removes any error messages if the file is not present # *.o mean all files ending with .o clean: rm -rf *.o rm -f *.out 11
Demonstration in compute.cs.tamu.edu Tabs, not spaces compiling, running and cleaning the project using Makefile Selective compiling Up to date target Changing timestamp of .cpp .h files (recompiling efficiently using Makefile): touchfilename Could be used if you did update the file with something non-code related Avoid being recompiled Adding a run target
Makefile another Example Consider a cpp project with files: driver.cpp Point.h Point.cpp Rectangle.h Rectangle.cpp Our objective is to create a makefile, with the end goal of a executable named proj1 A file/target may depend on one or more other files Need to ensure correct compilation order
Dependency Graph of the files output.out Depends on driver.o Point.o Rectangle.o Link driver.cpp Point.cpp Rectangle.cpp Compile Rectangle.h Include Point.h Source: https://www.cs.bu.edu/teaching/cpp/writing-makefiles/
Exercise (Write on paper OR compute) output.out Depends on driver.o Point.o Rectangle.o Link Create a makefile for the dependencies for this chart. Use the reminder code below to help. No code is given. YET driver.cpp Point.cpp Rectangle.cpp Compile Rectangle.h Include output.out: Airplane.o driver.o g++ -Wall -std=c++11 Airplane.o driver.o o output.out Point.h Airplane.o: Airplane.cpp Airplane.h g++ -Wall -std=c++11 -c foobar.cpp
Solution Makefile output.out: driver.o Point.o Rectangle.o g++ -Wall std=c++11 driver.o Point.o Rectangle.o -o output.out driver.o: driver.cpp Rectangle.h Point.h g++ -Wall -std=c++11 -c driver.cpp Point.o: Point.cpp Point.h g++ -Wall -std=c++11 -c Point.cpp Rectangle.o: Rectangle.cpp Rectangle.h Point.h g++ -Wall -std=c++11 -c Rectangle.cpp all: driver.o Point.o Rectangle.o #same as output.out g++ -Wall std=c++11 driver.o Point.o Rectangle.o -o output.out # -c compile # -o output clean: rm -rf *.o rm -f *.out run: ./output.out 16
Exercise to be submitted to ecampus Login to compute.cs.tamu.edu using your netid and password Putty in Windows Terminal (SSH) in mac or Linux Create a folder csce221 ; and a subfolder lab1_makefile1 and go inside it using the cd command mkdir csce221 cd csce221 mkdir lab1_makefile1 cd lab1_makefile1 Retrieve the files using (after the first one below, just hit up arrow and edit the filename at the end) wget https://people.engr.tamu.edu/slupoli/notes/DataStructures/labs/code/makeFileLecturer/Lecturer.cpp wget https://people.engr.tamu.edu/slupoli/notes/DataStructures/labs/code/makeFileLecturer/Lecturer.h wget https://people.engr.tamu.edu/slupoli/notes/DataStructures/labs/code/makeFileLecturer/driver.cpp wget https://people.engr.tamu.edu/slupoli/notes/DataStructures/labs/code/makeFileLecturer/Makefile.txt Code the cpp and .h files and complete the included Makefile using your favorite editor See the comments inside the cpp and .h files Compile and run the code, using the Makefile By executing make make run %You should have a target named run inside your Makefile make clean Compress the .cpp , .h and Makefile to a single zipfile(Don t include .o or the executable or the original tar file) Upload the zip file to ecampus under Makefile1
Alternative ways to access the server Use sftp (port 22) to connect to sftp://compute.cs.tamu.edu to upload or download your files. FileZilla WinSCP Terminal Zip the files GUI tools: 7zip, winzip, winrar Cmd: zip r xxx.zip yourfiles Note: compute.cs.tamu.edu is RHEL 7.7
Questions ? (survey next!)
Complete the survey https://goo.gl/forms/8jQ0296hACw4Lftl2 Click the link from eCampus