Understanding Cookies and Sessions in Servlets
Exploring the concepts of cookies and sessions in servlets, including how cookies work, managing cookies in servlets, and an example servlet code for handling user input using cookies. Learn about the basics of servlets and how they interact with client-side cookies to enhance web application functionality.
Uploaded on Oct 08, 2024 | 0 Views
Download Presentation
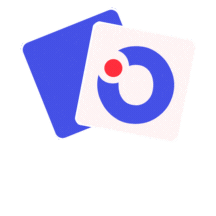
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Servlets (part 2) Dr Solange Karsenty Hadassah Academic College
? (1) Cookies - (2) Session - (3) (4) ? servlets - servlet ? Chaining Servlets RequestDispatcher (include/forward, difference redirect)
- (1) Cookies http , / : Cookies http : Cookies are private to each website: cookies of website x.y.com are not readable by z.com and vice versa. Within a website, cookies can be associated to a folder. It will be available to all pages in that folder and subdirectories (you can hide cookies from parent folders). Cookies are used for non-sensitive information (do not store credit cards) because the cookies file is readable on your computer You can manually delete cookies in your browser (in settings) Cookies length is limited; it cannot be used to store long information (around 4Kb, you can check on your browser the limit) Cookies can have an expiration date: these will be automatically erased at the expiration date (for example you may want to forget stored user information after a month). Cookies can be created, read and updated on the client side (with Javascript) AND on the server side (PHP, Java, javascript etc). So how does code on the server side read/write cookies that are stored on the client? Because: All cookies are sent to the server and back to the browser for each new http request (whenever you access a URL, click on a link, submit a form, or when you perform an Ajax call). All cookies are sent to the server and attached back to the page returned to the browser and the browser updates cookies (this is how the server can write cookies). 3
Managing Cookies Get the cookies from the service request: Cookie[]HttpServletRequest.getCookies() Add a cookie to the service response: HttpServletResponse.addCookie(Cookie cookie) Cookie getter methods: getName(), getValue(), getPath(), getDomain(), getMaxAge, getSecure Cookie setter methods: setValue() , setPath(), setDomain() 4
Example <html> <head> <title>Login Page</title> </head> <body> <h1>Logon to My Site</h1> <form action="/WelcomeBack" method="get"> Your Name: <input type="text" name="username"> <input type="submit"> </form> </body> </html> File login.html 5
public class WelcomeBack extends HttpServlet { public void doGet(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException { String user = req.getParameter("username"); if (user == null) { // see (3) Cookie[] cookies = req.getCookies(); for (int i = 0 ; cookies!=null && i < cookies.length ; i++) if (cookies[i].getName().equals("username")) user = cookies[i].getValue(); } else // user filled the name in the form res.addCookie(new Cookie("username", user)); // see (2) // here we are in in the case user never filled the form so we send him // to the login page res.sendRedirect("/dbi-servlets/login.html"); } } } if (user != null) // see (2) res.setContentType("text/html"); PrintWriter out = res.getWriter(); out.println("<html><body><h1>Welcome Back " + user + " </h1></html></body>"); } else { 6
(1) This page is a static HTML page with a form to call the WelcomeBack Servlet 7
(2) This page is the response of the WelcomeBack Servlet after the user has pressed the submit button. This is a GET request: the parameters from the form are appended in the request URL. The Servlet method doGet() is called. 8
(3) This page is the response of the WelcomeBack Servlet when one enters the URL http:// /WelcomeBack in the brower and hits return: This is a simple GET request sent to the Servlet without any parameters. The Servlet method doGet() is called. In this example, the user previously entered his name with the form so it is read by the servlet and displayed. 9
(2) Sessions () , session ID ) session session ID http session- ( : session expiration time . . 10
Accessing the Session Data Session data is represented by the class HttpSession Use the methods getSesssion() or getSession(true) of the doGet/doPost/doXXX request to get the current HttpSession object, or to create one if it doesn t exist Use getSession(false) if you do not want to create a new session if no session exists 11
HttpSession Methods Session Strings Cookies - ) ( Attributes Session data is accessed in a hash-table fashion: - setAttribute(String name,Object value) - ? - Object getAttribute(String name) More methods: - removeAttribute, getAttributeNames - Invalidate - isNew, getId - getCreationTime, getLastAccessedTime - getMaxInactiveInterval, setMaxInactiveInterval ) ( 12
: In the following example a basic shopping cart for an online store is implemented The application consists of two Servlets: - Store.java: the main store site - ShoppingCart.java: handles cart manipulation - Each servlet handles different pages, depending on the shopping cart content and if the user already visited the store or not - In general, a servlet handles multiple pages corresponding to different states of website 13
(1) This is displayed by the Store servlet as the user enters the http:// /Store URL in the browser and hits return. A GET request is sent to the Servlet. The user never visited before and no shopping cart object exists yet in the session. 14
(2) This is displayed by the ShoppingCart servlet as the user hits the submit button of previous page. 15
(3) This is displayed by the Store servlet as the user enters the http:// /Store URL in the browser and hits return. A GET request is sent to the Servlet. The user has a non empty shopping cart displayed by the servlet. 16
(4) This is displayed by the ShoppingCart servlet as the user enters http://../ShoppingCart in the browser and hits return.S GET request is sent without any parameters. 17
public class Store extends HttpServlet { public void doGet(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException { res.setContentType("text/html"); PrintWriter out = res.getWriter(); out.println("<HTML><HEAD><LINK rel='stylesheet' type='text/css href= 'cartstyle.css'></HEAD><BODY>"); HttpSession session = req.getSession(); List itemList = (List)session.getAttribute("item-list"); if(itemList==null) { out.println("Hello new visitor!<br><br>"); itemList = new LinkedList(); session().setAttribute("item-list", itemList); } for(Iterator it = itemList.iterator(); it.hasNext();) out.println("<LI>"+it.next()+"</LI>"); out.println("</I></OL>"); out.println("<FORM method='POST' action= ShoppingCart'>Add item:<INPUT name='item' type='text'>" + "<INPUT type='submit' value='send'><BR><BR><INPUT type='submit' value='Empty Cart' name='clear'>" + "</FORM></BODY></HTML>"); out.close(); } } out.println("Your Shopping Cart:<OL><I>"); 18
public class ShoppingCart extends HttpServlet { public void doPost(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException { res.setContentType("text/html"); PrintWriter out = res.getWriter(); out.println("<HTML><HEAD><LINK rel='stylesheet type='text/css' href='cartstyle.css'></HEAD><BODY>"); List items = (List)req.getSession().getAttribute("item-list"); if (req.getParameter("clear")!= null) { // user hit the empty cart button (button name= clear ) items.clear(); out.println("Your Shopping Cart is Empty!"); } else { // user hit submit and added an item to cart String item = req.getParameter("item"); items.add(item); out.println("The item <I>" + item + "</I> was added to your cart."); } out.println("<BR><BR><A HREF='Store'> return to store</A>"); out.println("</BODY></HTML>"); // it s important close = to signal the browser we are finished sending the output out.close(); } } 19
(3) ServletContext ServletContext object Can store Web application initialization parameters Servlet container ) ( in ServletContext.getInitParameters Can store and manipulate application-shared attributes - servlets ) ( in ServletContext.get/setAttribute Can be used to access the logger web )! ( stdout debugging Can be used to dispatch requests to other resources 20
ServletContext Methods Access initialization parameters: getInitParameter(String name), getInitParameterNames() // paramscan be defined with the @WebInitParam annotation Web-application attributes: getAttribute(String name), getAttributeNames() setAttribute(String, Object), removeAttribute(String) Transform context-relative paths to absolute paths: getRealPath(String path), URL getResource(String path) 21
ServletContext Methods Write to the application log: log(String msg), log(String message, Throwable exception) Get a resource dispatcher (discussed later): RequestDispatcher getRequestDispatcher(String path) Name and version of the servlet container: String getServerInfo() 22
- response response Servlet 2 other servlets Html pages - response - request
(4) RequestDispatcher servlet ) servlet ) ( ( html The RequestDispatcher object is used to send a client request to any resource on the server To send a request to a resource x, use: getServletContext().getRequestDispatcher( x ) 25
Request Dispatcher Methods Equivalent to the 3rd parameter (next) of a route in nodeJS (http request) : : servlet : void forward(ServletRequest request, ServletResponse response) Forwards a request from a servlet to another resource (servlet) Note: forward from doGet()/doPost() sends to doGet()/doPost() ( http response void include(ServletRequest request, ServletResponse response) Includes the content of a resource in the response ) - servlet 26
Browser still points to: /Shop /Shop include http request http response Usually set HEADERS here and CLOSE response stream here Shop include(req,res) include(req,res) include(req,res) include(req,res) Do NOT close the response stream here! Why? Header user details shopping cart Footer (static) (dynamic) (dynamic) (static)
In java out = response.getWriter(); RequestDispatcher rd = request.getRequestDispatcher("/header.html"); rd.include(request, response); rd = request.getRequestDispatcher("/userdetails"); // a servlet returning some HTML rd.include(request, response); // note that you can out.write() anywhere here too! rd = request.getRequestDispatcher("/shoppingcart"); // a servlet returning some HTML rd.include(request, response); rd = request.getRequestDispatcher("/footer.html"); rd.include(request, response); out.close(); // make sure to close ONLY AT THE END!!!
Browser still points to: /Checkout /Checkout forward http request http response Usually the LAST servlet in the chain is the one closing the response stream Dispatcher Servlet ) Checkout ShoppingCart ( ) - ( response : servlets forward(req,res) forward(req,res) authentication payment forward(req,res)
Common mistakes Closing the response stream in a Servlet that was included Closing the response stream before forwarding the request to another Servlet Setting the headers (setContentType()) AFTER we already sent data in the response: for example after inside an included Servlet while we already wrote back HTML to the client
) - ? ( 3 ? servlets cookies Data that will be used only for this request: request.setAttribute("key", value); Here you can pass for example form input to be processed Data will be used for this client (also for future requests one session = one client or user): session.setAttribute("key", value); Here you can store for example a shopping cart Data that will be used in the future for any client (the context is shared by all users of the website) context.setAttribute("key", value); Here you can put for example a counter of visitors. 31
) ( Redirect URL - 2 " http request/response - request " What are the advantages of having only one URL? SendRedirect triggers a second HTTP request from the browser Therefore you must use session/cookies if you need to pass data from another page 32
Servlets in one picture cookies cookies Servlet Lifecycle 1. The Servlet container calls the no-arg constructor. 2. The Servlet container calls the init() method. This method initializes the servlet and must be called before life of a servlet, the init() method is called only once. 3. After initialization, the servlet can service client requests. Each request is serviced in its own separate thread. The Web container calls the service() method of the servlet for every request. 4. Finally, the Servlet container calls the destroy() method that takes the servlet out of service. The destroy() method, like init(), is called only once in the lifecycle of a servlet. users request response Web Server + Servlet container tomcat The Servlet container maintains a bounded pool of worker threads to handle requests. thread thread thread response.addCookie( ) request.getCookies() Servlet A Servlet B Servlet C Your code request.setAttribute(..) // addd extra params dispatcher.forward(request, response) // forward to other servlet website session.setAttribute( ) tomcat object session session object object object object session.getAttribute( ) 34 object
More material Link to an online excellent tutorial: https://www.tutorialspoint.com/servlets/index.htm