Understanding Amity School of Engineering & Technology Vectors in Java
Amity School of Engineering & Technology Vectors provide a flexible way to manage dynamic arrays in Java programming. With capabilities to store objects of any type and accommodate dynamic resizing, these vectors offer optimized storage management through capacity and capacity increment settings. Constructors in the Vector class allow for customization of initial capacity and capacity increment values, enabling efficient vector usage in Java applications.
Download Presentation
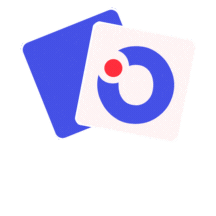
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Amity School of Engineering & Technology Vectors Vector is a predefined class in the util package of Java. This class can be used to create a generic dynamic array known as vector that can hold objects of any type and any number. The objects don t have to be homogenous. The Vector class implements a growable array of objects. Like an array, it contains components that can be accessed using an integer index. However, the size of a Vector can grow or shrink as needed to accommodate adding and removing items after the Vector has been created.
Amity School of Engineering & Technology Vector Each vector tries to optimize storage management by maintaining a capacity and a capacityIncrement. The capacity is always at least as large as the vector size; it is usually larger because as components are added to the vector, the vector's storage increases in chunks the size of capacityIncrement. An application can increase the capacity of a vector before inserting a large number of components; this reduces the amount of incremental reallocation.
Amity School of Engineering & Technology Fields capacityIncrement The amount by which the capacity of the vector is automatically incremented when its size becomes greater than its capacity. If the capacity increment is less than or equal to zero, the capacity of the vector is doubled each time it needs to grow. elementCount The number of valid components in this Vector object. Components elementData[0] through elementData[elementCount-1] are the actual items. elementData The array buffer into which the components of the vector are stored. The capacity of the vector is the length of this array buffer, and is at least large enough to contain all the vector's elements. Any array elements following the last element in the Vector are null.
Amity School of Engineering & Technology Constructors in Vector class Vector() Constructs an empty vector so that its internal data array has size 10 and its standard capacity increment is zero. Vector(int initialCapacity) Constructs an empty vector with the specified initial capacity and with its capacity increment equal to zero. Parameters: initialCapacity - the initial capacity of the vector. Throws: IllegalArgumentException - if the specified initial capacity is negative Vector(int initialCapacity, int capacityIncrement) Constructs an empty vector with the specified initial capacity and capacity increment. Parameters: initialCapacity - the initial capacity of the vector. capacityIncrement - the amount by which the capacity is increased when the vector overflows. Throws: IllegalArgumentException - if the specified initial capacity is negative
Amity School of Engineering & Technology Advantages of Vector over arrays It is convenient to use vectors to store objects. A vector can be used to store a list of objects that may vary in size. One can add and delete objects from the list as and when required.
Amity School of Engineering & Technology Cont. One drawback of using vectors is that one cannot directly store simple data types in a vector; only objects can be stored.
Amity School of Engineering & Technology Methods of Vector class vectorobject.addElement(item); Adds the items specified to the list at the end. vectorobject.elementAt(n); vectorobject.size(); Gives the name of the nth object Gives the number of the objects present. Removes the specified item from the list. Removes the item stored in the nth position of the list. Removes all the elements in the list. Copies all the items from the list to array. vectorobject.removeElement(item); vectorobject.removeElementAt(n); vectorobject.removeAllElements(); vectorobject.copyInto(arrayname); vectorobject.insertElementAt(item,n); Insert the item at nth position.
Amity School of Engineering & Technology Obj.capacity() Returns the current capacity of this vector. Obj.isEmpty() Returns true if and only if this vector has no components, that is, its size is zero; false otherwise. Obj.firstElement() Returns the first component (the item at index 0) of this vector. Obj.lastElement() the last component of the vector, i.e., the component at index size() - 1. Obj.setElementAt( obj, index) Sets the component at the specified index of this vector to be the specified object. The previous component at that position is discarded. The index must be a value greater than or equal to 0 and less than the current size of the vector.
Amity School of Engineering & Technology Program: import java.util.Vector; import java.io.*; class VectorTest { public static void main(String args[]) throws IOException { Vector v = new Vector(); System.out.print("\n The size of Vector is: " + v.size()); System.out.print("\n The capacity of vector is: " + v.capacity()); InputStreamReader isr = new InputStreamReader(System.in); BufferedReader br = new BufferedReader(isr); for(int i =0 ;i < 10; i++) { System.out.print("\n Enter the element: "); String s = br.readLine(); v.addElement(s); }
Amity School of Engineering & Technology Cont. System.out.print("\n The size of Vector is: " + v.size()); System.out.print("\n The capacity of vector is: " + v.capacity()); for(int i =0 ;i < 5; i++) { System.out.print("\n Enter the element: "); String s = br.readLine(); v.addElement(s); } } } System.out.print("\n The size of Vector is: " + v.size()); System.out.print("\n The capacity of vector is: " + v.capacity());
Amity School of Engineering & Technology import java.util.Vector; import java.io.*; class VectorTest2 { public static void main(String args[ ]) throws IOException { Vector v = new Vector(5); System.out.print("\n The size of Vector is: " + v.size()); System.out.print("\n The capacity of vector is: " + v.capacity()); InputStreamReader isr = new InputStreamReader(System.in); BufferedReader br = new BufferedReader(isr); for(int i =0 ;i < 5; i++) { System.out.print("\n Enter the element: "); String s = br.readLine(); v.addElement(s); } System.out.print("\n The size of Vector is: " + v.size()); System.out.print("\n The capacity of vector is: " + v.capacity()); Program 2:
Amity School of Engineering & Technology Cont. for(int i =0 ;i < 3; i++) { System.out.print("\n Enter the element: "); String s = br.readLine(); v.addElement(s); } } } System.out.print("\n The size of Vector is: " + v.size()); System.out.print("\n The capacity of vector is: " + v.capacity());
Amity School of Engineering & Technology Program: 3 import java.util.Vector; import java.io.*; class VectorTest { public static void main(String args[]) throws IOException { Vector v = new Vector(5,2); System.out.print("\n The size of Vector is: " + v.size()); System.out.print("\n The capacity of vector is: " + v.capacity()); InputStreamReader isr = new InputStreamReader(System.in); BufferedReader br = new BufferedReader(isr); for(int i =0 ;i < 5; i++) { System.out.print("\n Enter the element: "); String s = br.readLine(); v.addElement(s); } System.out.print("\n The size of Vector is: " + v.size()); System.out.print("\n The capacity of vector is: " + v.capacity());
Amity School of Engineering & Technology Cont. for(int i =0 ;i < 3; i++) { System.out.print("\n Enter the element: "); String s = br.readLine(); v.addElement(s); } } } System.out.print("\n The size of Vector is: " + v.size()); System.out.print("\n The capacity of vector is: " + v.capacity());