Understanding Single-File Source Code Programs in Java 11
Explore the concept of single-file source code programs in Java 11, where the entire program is contained within a single .java file without external dependencies. Learn how to compile and run these programs both before and after Java 11, and discover the new feature of executing Java programs directly from the source code file without separate compilation.
Download Presentation
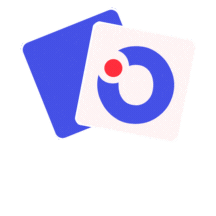
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Single Single- -File Source File Source- -Code Programs and and Higher Higher versions Code Programs in Java 11 versions in Java 11 By Dr. M. Mohamed Surputheen
single file source code programs single file source code programs A single-file source code program is one where the program fits in a single source file. A single-file source code program in Java 11 is a program that contains all of its code in a single .java file, and does not require any external dependencies to run. This means that all the code needed to run the program is contained within a single file, including the main method. There are no restrictions on the number of classes in the file.
Compiling and Running a java single Compiling and Running a java single- -file program before and after java 11 file program before and after java 11 Before Java 11, compiling and running a Java program, including a single source file, in the command line involved two steps, as follows: 1.Compiling: The first step was to use the Java compiler (javac) to compile the Java source code into bytecode. The command to compile a Java source file named "MyFile.java" would look like this: javac MyFile.java 2. Running: The second step was to use the JVM with the "java" command to execute the compiled bytecode. The command to execute the "MyFile.class" file would look like this: java MyFile
Java 11 introduced a new feature called "single-file source-code execution". This allows us to run a Java program directly from the source code file, without having to compile it separately. To run a single-file Java program, we simply use the "java" command followed by the name of the source file (with the .java extension). For example, to run a file named "Hello.java", we would run the command "java Hello.java Note: In Java 11 version, we can still follow the old convention of using the javac command to compile Java source files and the java command to execute the compiled class files.
Execute Java single file program Without Compilation Execute Java single file program Without Compilation Example: Example: class Welcome { public static void main(String[] args) { System.out.println(" Welcome to Java" ); System.out.println(" This code runs without needing to be compiled beforehand"); System.out.println(" Java version is " + System.getProperty("java.version")); } } I:\Java11>java Welcome.java Welcome to Java This code runs without needing to be compiled beforehand Java version is 16.0.1
Example 2:single file source code program with classes and objects // Define a class named HelloWorld class HelloWorld { // Define a method named greet public void greet() { System.out.println("Hello, World!"); } // Define the main method public static void main(String[] args) { // Create an object of the HelloWorld class HelloWorld obj = new HelloWorld(); // Call the greet method using the object obj.greet(); } } Output I:\Java11>java HelloWorld.java Hello, World!
Multiple classes can be defined within the same source file if needed To execute a single-file source-code program, the class containing the main method should be the first class of the file Here is an example of a Java single-file source code where the first class contains a main method. In this code, two classes are defined in a single file. class TestHellow { // Define the main method public static void main(String[] args) { // Create an object of the HelloWorld class HelloWorld obj = new HelloWorld(); // Call the greet method using the object obj.greet(); } } // Define a class named HelloWorld in the another class class HelloWorld { // Define a method named greet public void greet() { System.out.println("welcome to Java 11!"); } } I:\Java11>java TestHellow.java welcome to Java 11!
Here is an example of a Java single-file source code where the first class does not contain a main method. In this code, two classes are defined in a single file. Example3 // Define a class named HelloWorld class HelloWorld { I:\Java11>java TestHello.java error: can't find main(String[]) method in class: HelloWorld But we compile and run the program , It will work // Define a method named greet public void greet() { System.out.println("Hello, World!"); } } I:\Java11>javac TestHello.java class TestHello { I:\Java11>java TestHello Hello, World! // Define the main method in the second class public static void main(String[] args) { // Create an object of the HelloWorld class HelloWorld obj = new HelloWorld(); // Call the greet method using the object obj.greet(); } }
If you have two .java files in your program, you cannot use this feature and must use javac instead. Here's an example program in Java with two .java files: 1.Main.java class Main { public static void main(String[] args) { Helper helper = new Helper(); System.out.println(helper.getMessage()); } } 2.Helper.java class Helper { public String getMessage() { return "Hello from Helper!"; } } I:\Java11>java Main.java Main.java:3: error: cannot find symbol Helper helper = new Helper(); ^ symbol: class Helper location: class Main Main.java:3: error: cannot find symbol Helper helper = new Helper(); ^ symbol: class Helper location: class Main 2 errors error: compilation failed Traditional way to run the Java program I:\Java11>javac Main.java I:\Java11>java Main Hello from Helper!
Command line arguments command line arguments can be passed to a Java program just like with any normally compiled class. Example: // Display all command-line arguments. class CommandLine { I:\Java11>java CommandLine.java jamal mohamed college trichy args[0]: jamal args[1]: mohamed args[2]: college args[3]: trichy public static void main(String args[]) { for(int i=0; i<args.length; i++) System.out.println("args[" + i + "]: " + args[i]); } }
Note A single-file source program in Java 11 is limited to simple programs that do not have external dependencies. For example In Java 11, if we use external dependencies such as the MySQL JDBC driver, we cannot run the program without compilation. This is because a single-file source program in Java 11 is limited to simple programs that do not have external dependencies. Therefore, we must first compile the program with the external dependency using the command line. Once the program is compiled, we can run it as a regular Java application.