Networking Protocol Implementation Overview
This overview covers the process of receiving and processing neighbor states, searching for new neighbors, updating neighbor sets, handling timeouts, sending hello messages, and utilizing provided classes like Packet, UdpSocket, HostId, NeighborInfo, and HelloMessage in a networking protocol implementation. It also includes methods for handling data packets, binding sockets, managing hosts, and sending messages between instances of a program.
Download Presentation
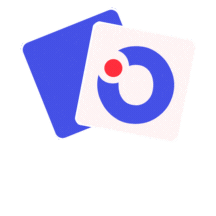
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
To do While (1) Receive hello Process neighbor states (sort of like what we did) Search for more neighbors? Check and if so, search Update neighbor set If some neighbors have timed out, remove them Send hello messages to neighbors Send every 10 sec
Provided Classes Packet ptr: holds the data as a char buffer totalSize: When data is being written to the pkt, totalSize is the size of the buffer, which is Packet::bufferLength, which is 2000 When data is being read from pkt, total size is the total amount to data available for rading offset: where in the buffer data should be read or written from. This is an internal variable. There is no need to adjust this or use its value Before writing to pkt pkt.getReadyForWriting(); Other methods will be demonstrated in the next slides
Provided Classes UdpSocket E.g., bind socket to port 10000 UdpSocket udpSocket; udpSocket.bindToPort(10000); Get packet if ready Packet pkt; If (udpSocket.checkForNewPacket(pkt, 2)>0) // process data else // data is not ready Send data Packet pkt; // fill the packet udpSocket.sendTo(pkt, destinationHostId) Of type HostId, which is discussed next
Provided Classes HostId char _address[ADDRESS_LENGTH]; Boost::uint16_t _port; E.g., set address and port HostId host(address, port); E.g., get HostId from packet Packet pkt; HostId host; If (udpSocket.checkForNewPacket(pkt, 2)>0) host.getFromPacket(pkt); E.g., put HostId onto packet HostId thisHost; // thisHost is the the ip and port of this instance of the program. So messages send from this instance of the program are sent from the source thisHost. Code is included on creating thisHost Packet pkt; thisHost.addToPacket(pkt); udpSocket.sendTo(pkt, hostId); E.g., check if thisHost is the same as hostId If (thisHost == hostId) { // yes, they are the same } E.g., send pkt with thisHost to all host in allHost list Packet pkt; thisHost.addToPacket(pkt); for(HostId &hosted : allHost) udpSocket.sendTo(pkt, hostId);
Provided Classes NeighborInfo HostId hostId; // the id of neighbor time_t timeWhenLastHelloArrived; Functions addToPacket getFromPacket updateTimeToCurrentTime() == Only checks hostId s match, ignores timeWhenLastHelloArrived E.g., Packet pkt; for( NeighborInfo &neighbor : allNeighbors) Neighbor.addToPkt(pkt);
Provided Classes HelloMessage uint8_t type // not important for this project HostId source; uint16_t numberOfNeighbors; list<HostId> neighbors; Functions Show E.g., during development, include the following to see the contents of the message and verify that the data is sensiable: helloMessage.show() addToPacket getFromPacket addToNeighborList E.g., add neighbors to helloMessage HelloMessage helloMessage; helloMessage.type = HELLO_MESSAGE_TYPE; helloMessage.source = thisHost; for(NeighborInfo &neighborInfo : bidirectionalNeighbors) helloMessage.addToNeighborList(neighborInfo.hostId); // helloMessage.show(); // for debugging and testing olny Packet pkt; pkt.getPktReadyForWriting . helloMessage.addToPacket(pkt); udpSocket.sentTo(pkt, someDestinationHostId);
Classes, Class Methods, and Examples unneeded methods are not listed class UdpSocket: used to send a receive a packet UdpSocket udpSocket; // declares a socket object udpSocket.bindToPort(portNum); // binds the socket to port portNum udpSocket.checkForNewPacket(pkt, timeout); // Checks for a new packet. Returns a positive value if a packet has arrive and returns 0 or less than 0 if no packet arrived pkt is a Packet object timeout is the maximum time (in seconds) to wait for a packet. If no packet arrives within this time, then the function returns with value 0 udpSocket.sendTo(pkt, hostId); // sends packet pkt to destination hostId class Packet: holds raw data for sending or receiving via a UdpSocket object Packet pkt; // declare a packet object pkt.getReadyForWriting(); // gets the packet object ready for writing
Classes, Class Methods, and Examples unneeded methods are not listed class HostId: holds data about a host (specifically, the IP address and port the host is using) HostId hostId; // declares a host object hostId.show(); // prints the IP address and port hostIdA == hostIdB ; // compares two hostId and returns true if both hosts have the same IP address and port class NeighborInfo: holds data about a neighbor (specifically, the neighbor s HostId and the last time when a hello was received from the neighbor) NeighborInfo neighbor; // declares the neighborInfo object neighborA == neighbor; // compares two neighbors and returns true if the neighbors have the same IP address and port. Does to compare the last time a hello was received neighbor.updateTimeToCurrentTime(); // sets the timeWhenLastHelloArrived Neighbor.hostId // the hostId of the neighbor object class HelloMesssage: holds a hello message for sending or that has been received HelloMessage helloMessage; // declares a HelloMessage object helloMessage.getFromPacket(pkt); // reads the hello message from the pkt and puts it into a HelloMessage object helloMessage.addToPacket(pkt); // puts data from a HelloMessage objective into a pkt object helloMessage.source // the HostId of the sender of the HelloMessage helloMessage.source == hostIdA // is true if the source of the helloMessage is hostIdA for (NeighborInfo & neighbor : bidirectionalNeighbors) { if neighbor.hostId == helloMessage.source printf( is bidirectional\n ); } // prints message if the source of the hello message is in the bidirectionalNeighbors list helloMessage.source = thisHost; // sets the source of the helloMessage to be this host helloMessage.addToNeigbhorList(hostId); // adds a hostId to the list of recently heard neighbors in the helloMessage for (NeighborInfo & neighbor : bidirectionalNeighbors) { helloMessage.addToNeighbors(neighbor.hostId); } // adds the hosts in the bidirectionalNeighbors list to the helloMessage for (HostId & hostId : helloMessage.neighbors) { hostId.show(); } // prints the hostId of every host listed in the hello message s list of recently heard neighbors helloMessage.show(); // prints the contents of the helloMessage