JavaScript Functions for User Interaction in Web Pages
JavaScript is typically embedded in a web page, with users interacting indirectly through functions such as prompt(), confirm(), and alert() for input and output. This programming practice involves popping up windows for user input, displaying messages, and computing values based on user-provided data. Explore various scenarios where JavaScript functions are utilized to facilitate user interaction in web development.
Download Presentation
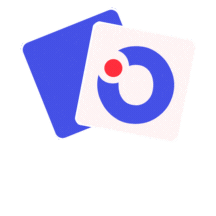
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
JavaScript typically embedded in a web page User doesn t interact directly with the interpreter Functions used to receive Input prompt() confirm() Functions for Output alert() document.write() Programming Part II 2
Pops up a window that allows a user to enter a value Displays a message indicating what info user is supposed to provide Data entered with prompt is returned as a string* Can optionally specify a default answer Usage var userInput = prompt( Enter a number. ); var userInput = prompt( Enter a number. , 0); var userInput = prompt( Enter your name. , W. T. Door ); Programming Part II 3
Pops up a window with message Message to display is passed to the function as a String Usage alert( Hello, world! ); Programming Part II 4
var input1 = prompt("Enter your height in inches"); var input2 = prompt("Enter your weight in pounds"); var height = Number(input1); var weight = Number(input2); var multiplier = 25*12/height; // NOTE: weight is proportional to the cube of the height! var newWeight = multiplier*multiplier*multiplier*weight; alert("If you were 25 feet tall, you would weigh " + newWeight + " pounds!"); How would we modify the program to asks the user for the target height (in feet) rather than simply assume 25 feet? How might we round up or round down? Programming Part I 5
User inputs bill amount Need to establish/designate a variable Script a prompt for this variable input User inputs percentage tip desired Need to establish/designate a variable Script a prompt for this variable input Program computes tip Establish formula in program Program outputs tip amount alert outputs tip amount Programming Part I 6
//this program sums expenses and assigns the variable total //a new value based on total's old value and new additions var num = 0; var total = 0; what is the value of total here? num = prompt("A burger is $1.25. How many do you want?"); total = total + num * 1.25; what is the value of total here? num = prompt("A bag of fries is $0.90. How many do you want?"); total = total + num * 0.90; what is the value of total here? num = prompt("A drink is $1.40. How many do you want?"); total = total + num * 1.40; what is the value of total here? alert("Your total is $" + total + "."); Question: How can I add onto this program so that the alert box repeats the customer's order along with printing out the total cost? Programming Part I 7
var total = 0; var num = 0; var order = ""; // Look Mom, an empty string! // It is to strings what zero is to numbers. num = prompt("A burger is $1.25. How many do you want?"); total = total + num * 1.25; order = order + num + " burgers, " num = prompt("A bag of fries is $0.90. How many do you want?"); total = total + num * 0.90; order = order + num + " fries, and " num = prompt("A drink is $1.40. How many do you want?"); total = total + num * 1.40; order = order + num + " drinks. " alert("Your order is " + order + "Your total is $" + total + "."); Programming Part I 8
Exam expectations: You are expected to be able to understand and modify simple JavaScript programs as a means of demonstrating that understanding, but we are not going to ask you to write a program from scratch to solve a problem. That's beyond the scope of this course. Programming Part I 9