Java Programming: Multidimensional Arrays
Delve into the world of multidimensional arrays in Java programming with a focus on two-dimensional arrays. Learn how to create table-like data structures, understand array declarations, reference elements efficiently, and even pass 2D arrays to methods. Explore examples, declarations, and a practical exercise to construct a multiplication table using nested loops.
Download Presentation
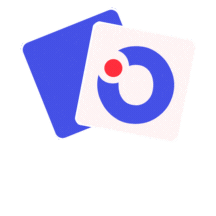
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
BUILDING JAVA PROGRAMS CHAPTER 7.5 MULTIDIMENSIONAL ARRAYS 1
TWO-DIMENSIONAL ARRAY Allow for the creation of table-like data structures with a row and column format. The first subscript is a row index in a table while the second subscript is a column index in a table. Declaration type[][] name = new type [row size][col size] cols 0 1 2 3 [0][3] [1][3] [2][3] Examples 0 1 2 [0][1] [1][1] [2][1] [0][2] [1][2] [2][2] [0][0] [1][0] [2][0] rows int [][] grid = new int[3][4]; 2
DECLARATIONS int[][]table = new int[3][4]; int[][] table = { {0,0,0,0}, {0,0,0,0}, {0,0,0,0} }; Think of a 2D Array as An Array of Arrays 3
REFERENCING ELEMENTS OF 2D ARRAY The array below is a 3 x 5 array of ints Declaration: Int [][] grid = new int[3][5]; 2 4 6 8 10 3 6 9 12 15 grid[0][0]= 4 8 12 16 20 grid[2][2]= grid[1][3]= grid[3][0]= 4
REFERENCING ELEMENTS OF 2D ARRAY The array below is a 3 x 5 array of ints Declaration: char [][] grid = new char[3][5]; A B C D E V W X Y Z grid[0][0]= H I J K L grid[2][2]= grid[1][3]= grid[0][5]= 5
REFERENCING ELEMENTS OF 2D ARRAY int[][] grid = new int[3][5]; for (int row = 0; row < 3; row++){ for (int col = 0; col < 5; col++){ grid[row][col] = row + col; } } 6
PASSING TWO-DIMENSIONAL ARRAYS TO METHODS public void printTable (int[][] table){ for (int row = 0; row < table.length; row++){ for (int col = 0; col < table[row].length; col++){ System.out.printf( %4d , table[row][col]); } System.out.println(); } } 7
LETS TRY SOME CODE Write a piece of code that constructs a two-dimensional array of integers named table with 5 rows and 10 columns. Fill the array with a multiplication table, so that array element [i][j] contains the value i * j. Use nested for loops to build the array. 8
MULTIPLICATION TABLE int[][] table = new int[5][10];//declaration int[][] table = new int[5][10];//declaration for (int i = 0; i < 5; i++) { for (int i = 0; i < table.length; i++) { for (int j = 0; j < 10; j++) { for (int j = 0; j < table[i].length; j++) { table[i][j] = i * j; table[i][j] = i * j; } } } } 9
MORE CODE MATRIX ADD Write a method named matrixAdd that accepts a pair of two-dimensional arrays of integers as parameters, treats the arrays as 2D matrices and adds them, returning the result. The sum of two matrices A and B is a matrix C where for every row i and column j, Cij = Aij + Bij. You may assume that the arrays passed as parameters have the same dimensions. 10
public static int[][] matrixAdd(int[][]a, int[][]b){ int rows = a.length; int cols = 0; if (rows>0){ cols=a[0].length; } int[][]sum= new int[rows][cols]; //declare sum matrix for(int r=0;r<a.length;r++){ for(int c=0;c<a[r].length;c++){ sum[r][c]=a[r][c] +b[r][c]; } } return sum; } 11
WHAT WE COVERED Create, populate and traverse multidimensional arrays! 12