Implementation Pointers for Secure Data Handling
In this project, we delve into the implementation of secure data handling techniques, including creating a table with encrypted columns in MySQL, generating a symmetric key, loading data into a database, and reading data securely. From encrypting sensitive information like credit card details to securely storing and retrieving data, this project showcases best practices for safeguarding sensitive information.
Download Presentation
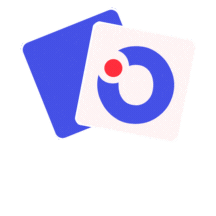
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Project 2 Implementation Pointers Tyler Moore CS 7403, University of Tulsa 1
goto_cart.py link form login.py signup.py confirm.py Tx PAN, Request TPAN Tx (TPAN , CVV, exp, amt, ) pay_tok.py issue_tok.py Tx TPAN No Valid PAN? get_pan.py Tx PAN Yes Tx TPAN pay_pan.py store_tok.py No Auth? Merchant https://127.0.0.2 DB: goldies (user: root, pwd: SEcom7403) /var/www/p2/ Token Vault https://127.0.0.149 DB: tv (user: tokenv, pwd: IndirectionRocks) /var/www/tokenvault/ Yes Issuer https://127.0.0.99 DB: ccissuer (user: issuer, pwd: HamsterEMV) /var/www/issuer/ thanks.py 2
How to create a table with encrypted columns (MySQL) Data: PAN CVV Expmo Expyear ZIP Name 5123456789012340 123 12 2016 74104 Henry Kendall 5123456789012340 383 8 2017 74114 Paschal Twyman 5678901234567890 148 12 2018 2138 Drew Faust 4567890123456780 490 12 2018 2482 Kim Bottomly 3456789012345670 668 4 2019 75214 Gerald Turner create table ccinfo (ivpan BLOB, pan BLOB, ivcvv BLOB, cvv BLOB, expmonth INT, expyear INT, billzip INT, customer VARCHAR(40),primary key (expmonth,expyear,billzip,customer)); 3
How to make a symmetric key #From /home/webdev/issuer/loadCC.py import os,binascii from Crypto.Cipher import AES ekey = os.urandom(32) #write the key in hexadecimal format to file keyf= open('/home/webdev/issuer/issuer.aes.key','w') keyf.write(binascii.b2a_hex(ekey)) keyf.close() 4
Loading into database (loadCC.py) for line in open("/home/webdev/issuer/ccnums.csv"): (pan,cvv,expmonth,expyear,billzip,name) = line.split(',') name = name.strip() #generate random initialization vector paniv = os.urandom(16) # Encryption enc = AES.new(ekey, AES.MODE_CFB, paniv) panc = enc.encrypt(pan) #cvv cvviv = os.urandom(16) cvvenc = AES.new(ekey, AES.MODE_CFB, cvviv) cvvc = cvvenc.encrypt(cvv) stmt = "INSERT INTO ccinfo values (%s,%s,%s,%s,%s,%s,%s,%s);" c.execute(stmt,(paniv,panc,cvviv,cvvc,expmonth,expyear,billzip,name)) conn.commit() 5
Reading out of the database #/var/www/issuer/authLocal.py import os,binascii from Crypto.Cipher import AES ekeyhex = "257b44ef6da5538c199227312ba24703dbf96195dda0851cc3543c9e3315b994" expmonth=4 expyear=2019 billzip=75214 customer="Gerald Turner" pan = "3456789012345678" cvv = "668" cmd = "SELECT ivpan,pan,ivcvv,cvv FROM ccinfo WHERE expmonth=%s and expyear=%s and billzip=%s and customer=%s;" c.execute(cmd, (expmonth,expyear,billzip,customer)) (ivpan,panc,ivcvv,cvvc) = c.fetchone() 6
Reading out of the database (2) # Decryption ekey = binascii.a2b_hex(ekeyhex) #PAN dec = AES.new(ekey, AES.MODE_CFB, ivpan) dbpan = dec.decrypt(panc) #CVV dec = AES.new(ekey, AES.MODE_CFB, ivcvv) dbcvv = dec.decrypt(cvvc) if dbpan == pan and dbcvv == cvv: print "Success" else: print dbpan, pan print dbcvv, cvv 7
goto_cart.py link form login.py signup.py confirm.py Tx PAN, Request TPAN Tx (TPAN , CVV, exp, amt, ) pay_tok.py issue_tok.py Tx TPAN No Valid PAN? get_pan.py Tx PAN Yes Tx TPAN pay_pan.py store_tok.py No Auth? Merchant https://127.0.0.2 DB: goldies (user: root, pwd: SEcom7403) /var/www/p2/ Token Vault https://127.0.0.149 DB: tv (user: tokenv, pwd: IndirectionRocks) /var/www/tokenvault/ Yes Issuer https://127.0.0.99 DB: ccissuer (user: issuer, pwd: HamsterEMV) /var/www/issuer/ thanks.py 8
How to communicate between pages 1. Browser submits form data (should use POST) 2. Server submits data via POST 3. Server redirects 9
Server submits data via POST #/var/www/issuer/testPost.py import urllib import urllib2 url = 'https://127.0.0.149/receiveTest.py' values = {'firstname' : 'foo', 'lastname' : 'bar' } data = urllib.urlencode(values) req = urllib2.Request(url, data) response = urllib2.urlopen(req) the_page = response.read() print "Content-Type: text/html" print "" print the_page 10
Server receives data via POST #/var/tokenvault/receiveTest.py print "Content-Type: text/html" print "" form = cgi.FieldStorage() for item in form.keys(): print item, form[item] 11
Server redirects to another location #/var/www/tokenvault/testRedir.py import cgi, cgitb cgitb.enable() print "Location: https://127.0.0.2/index.py" print "" 12