Understanding Structures and Unions in C Programming
Exploring the concept of structures and unions in the C programming language, this reference material covers their definition, initialization, assignment, members, arrays, and nested structures. Learn how structures aggregate data types and how unions can share memory locations. Gain insights into struct syntax, examples, initialization rules, assignments, and practical applications in programming.
Download Presentation
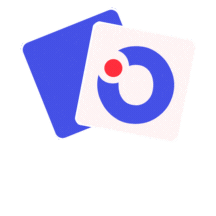
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Structure & Union Reference: 10.1-10.3, 10.5, 11.4 Prepared by: Johra Muhammad Moosa Assistant Professor Department of Computer Science & Engineering Bangladesh University of Engineering & Technology
Structure Aggregate data type Composed of two or more related variables Called member Each member can be of different types
Structure (General Form) struct tag-name { type member1; type member2; type member3; . . . type memberN; } variable-list; The keyword struct means that a structure type is defined tag-name is the name of the type Either tag-name or variable-list is optional
Structure Example struct point { int x; int y; } p1, p2; struct { int x; int y; } p1, p2;
Structure Example struct point { int x; int y; }; struct point p1, p2; Keyword struct before variable declaration is necessary Each instance of a structure contains its own copy of the members Structure declaration without any variable name does not reserve any storage Describes template or shape of a structure
Structure Initialization p1.x=10; p1.y=5; struct point p3={5, 2}; p2={10, 5};//error, not possible
Structure Assignment Possible when type of the both objects are same p2=p3;
Structure Member printf("%d, %d\n", p3.x, p3.y); scanf("%d %d\n", &p3.x, &p3.y);
Structure Array #include <stdio.h> struct point { int x; int y; } ap[10] ; int main(void) { struct point p[10]; int x; for(x=0; x<10; x++) { scanf("%d %d", &p[x].x, &p[x].y); } return 0; } Member x and int x are different
Nested Structure #include <stdio.h> struct point { int x; int y; } p1, p2; struct rect { struct point p1; struct point p2; }; int main(void) { struct rect r1; r1.p1.x=10; r1.p1.y=5; printf("%d, %d\n", r1.p1.x, r1.p1.y); return 0; }
Function Returning Structure struct point makepoint (int x, int y) { struct point temp; temp.x=x; temp.y=y; return temp; }
Pointer to Structure struct point *pp; pp=&p3; pp->x=4; pp->y=8; printf("%d, %d\n", p3.x, p3.y);
typedef (user defined data types) typedef type new-type type: an existing data type Example: typedef int age age is a user defined data type, equivalent to int typedef double height[100]; height male, female;
typedef with Structure typedef struct { } new-type; typedef struct { int month; int day; int year; } date;
Union Single piece of memory shared with multiple variables When values need not be assigned to all of the members at any one time Individual data types may differ from one another Members within a union share the same storage area The user must keep track of what type of information is stored at any given time union tag{ member 1; member 2; member n; };
Union union u_type { int i; char c[2]; double d; }sample; Assuming 8 bytes double, 2 bytes int, and one byte char d c[0] c[1] i
Union Example #include<stdio.h> int encode (int i); int encode(int i) { union crypt_type{ int num; char c[2]; }crypt; unsigned char ch; crypt.num=i; ch=crypt.c[0]; crypt.c[0]=crypt.c[1]; crypt.c[1]=ch; return crypt.num; } int main () { int i; i=encode(10); printf("10 is encoded to %d\n", i); return 0; }
Bit-fields (excluded from syllabus) A variation on a structure member Useful when data size is concern type name: size Number of bits: specified by size type is either int or unsigned If signed higher order bit is treated as sign bit
Bit-fields (excluded from syllabus) struct b_type{ unsigned dept: 3; //upto 7 dept unsigned instock: 1; //0 if not in stock, 1 otherwise }inv[10]; If(!inv[4].instock) printf( Out of stock );