Understanding Inter-Process Communication Signals in Operating Systems
Signals in inter-process communication are asynchronous notifications delivered to specific processes, allowing event-based programming. Processes can handle signal delivery by ignoring it, terminating, or invoking a signal handler. Signal handlers can be written in two ways - one handler for many signals or one handler for each signal. A signal example demonstrates how a process sends a signal to another process through the kernel. Handling signals efficiently is crucial in operating systems.
Download Presentation
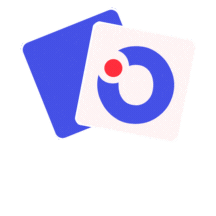
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Inter-Process Communication: Signals David Ferry, Chris Gill, Marion Sudvarg, James Orr CSE 422S - Operating Systems Organization Washington University in St. Louis St. Louis, MO 63130 1
Signals Asynchronous notifications: Generated by hardware or requested by another process Delivered to a specific process Processes receivesignals and respond Allows for event-based programming Conceptually is very similar to hardware interrupts and exceptions, but for processes CSE 422S Operating Systems Organization 2
Signal Receipt For each type of signal, a process can handle signal delivery by: 1. Ignoring it 2. Terminating 3. Invoking a signal handler Additionally, a process may block delivery of certain signals. Undelivered signals are said to be pending. A single bit indicates pending status for each signal type. Pending signals are not queued. (Except real-time signals!) CSE 422S Operating Systems Organization 3
Signal Handler Example #include <signal.h> void sigusr1_handler( int signum ){ //Handle signal } int main( int argc, char* argv[]){ //Normal program flow } CSE 422S Operating Systems Organization 4
Two Ways to Write Signal Handlers 1 handler for many signals 1 handler for each signal void sig_handler( int signum ){ if (signum == SIGUSR1) { //Handle SIGUSR1 } if (signum == SIGUSR2) { //Handle SIGUSR2 } } void sig_handler_sigusr1( int signum ){ //Handle SIGUSR1 } void sig_handler_sigusr2( int signum ){ //Handle SIGUSR2 } int main (int argc, char* argv[]){ struct sigaction ss1, ss2; ss1.sa_handler = sig_handler_sigusr1; ss2.sa_handler = sig_handler_sigusr2; sigaction( SIGUSR1, &ss1, NULL ); sigaction( SIGUSR2, &ss2, NULL ); } int main (int argc, char* argv[]){ struct sigaction ss; ss.sa_handler = sig_handler; sigaction( SIGUSR1, &ss, NULL ); sigaction( SIGUSR2, &ss, NULL ); } Functionally equivalent, matter of programming style, though handler for each signal avoids overhead of if statement CSE 422S Operating Systems Organization 5
Signal Example Process A (pid 1000) Executes code Process B (pid 2000) Event happens kill(2000, SIGUSR1); context switch context switch Kernel sends signal context switch context switch sigusr1_handler() CSE 422S Operating Systems Organization 6
Common Signals SIGINT keyboard interrupt (CTRL+C) SIGTERM terminate program SIGKILL kill program immediately SIGSEGV segmentation fault SIGUSR1 & SIGUSR2 Allows for the delivery of a notifications, but not for delivery of data. (Except real-time signals!) CSE 422S Operating Systems Organization 7
Signal Default Behaviors All signals have a default action. E.g.: SIGTERM terminate program SIGCHLD ignore signal See man 7 signalfor details Special signals that cannot be caught or blocked: SIGKILL (force quit vs. orderly shutdown) SIGSTOP CSE 422S Operating Systems Organization 8
Signals as Hardware Events A hardware event triggers an interrupt or exception handler that raises a signal, e.g.: Divide by zero (SIGFPE) Segmentation fault (SIGSEGV) These are synchronous with program flow! Note: Signals allow userspace programs to respond to and correct hardware-level faults. Compare to how page faults are handled entirely within the kernel. CSE 422S Operating Systems Organization 9
Signal Handler Concurrency A signal handler may be called at any point of execution! Creates a concurrent programming problem even in single threaded programs! Deadlock Races Many of the same risks/strategies of interrupt handlers apply here CSE 422S Operating Systems Organization 10
Concurrency Race Example char* buffer; void sig_handler( int signum ){ buffer = Handler called\n ; write( buffer ); } int main( int argc, char* argv[] ){ buffer = Main called\n ; write( buffer ); } CSE 422S Operating Systems Organization 11
More Subtle Concurrency Race Example int temp; void swap( int *a, int *b){ temp = *a; *a = *b; *b = temp; } What race can happen if this function is called from a signal handler and from elsewhere? CSE 422S Operating Systems Organization 12
Signal Safety Guidelines Use only async-signal-safe functions Many library functions, e.g. printf(), backed by static memory Main thread of execution calls printf(), begins writing to buffer Signal received, handler calls printf(), corrupts buffer Declare global shared variables volatile Prevents compiler from optimizing away reads from memory for value already in register Ensures main thread of execution sees updates from signal handlers Declare global shared variables sig_atomic_t Prevents inconsistent state of variable if update instructions interrupted by signal handler CSE 422S Operating Systems Organization 13
Signal-Driven I/O Described in LPI 63.3. A program: 1. Establishes a handler for SIGIO 2. Uses fcntl() to set process as owner of the file descriptor 3. Opens file with flags O_NONBLOCK and O_ASYNC (or updates flags with fcntl) 4. When file is ready for I/O, kernel invokes signal, caught by handler CSE 422S Operating Systems Organization 14
Real-Time Signals: Overview Provide an increased range of signals (SIGRTMIN to SIGRTMAX) May be sent with accompanying data Queued (up to a limit, SIGQUEUE_MAX) Delivery order is guaranteed (lowest- numbered signal delivered first) CSE 422S Operating Systems Organization 15
Increased Range Linux has many standard signals Only signals without assigned meaning are SIGUSR1 and SIGUSR2 But what if Process 1: Agent: processes batches of jobs Process 2: Monitor: monitors progress of Agent Agent reports status to Monitor after each batch Status 1: Completed Successfully Status 2: Completed with Errors Status 3: Incomplete Need 3 signal types! Use SIGRTMIN, SIGRTMIN+1, SIGRTMIN+2 CSE 422S Operating Systems Organization 16
Sending Real-Time Signals Use: sigqueue(pid_t pid, int sig, const union sigval value) Unlike kill(), can t target a process group sigval may contain An integer value or A (void) pointer to a data structure CSE 422S Operating Systems Organization 17
Receiving Real-Time Signals Just like any signal! Use: sigaction(int sig, struct sigaction * act, struct sigaction * oldact) act->sa_sigaction points to signal handler: void handler(int sig, siginfo_t * si, void * ucontext) si->si_value is union sigval CSE 422S Operating Systems Organization 18
Accompanying Data What if the Monitor needs to know the results of each batch? The Agent can assign an integer result to the sigval passed by sigqueue OR assign a pointer to a more complex data structure (the Monitor must know the pointer type to cast from void *) CSE 422S Operating Systems Organization 19
Queueing Example Agent (pid 1000) Monitor (pid 2000) Completes Batch 1 sigqueue(2000, SIGRTMIN+2, sigval) Completes Batch 2 sigqueue(2000, SIGRTMIN+2, sigval) context switch context switch Kernel sends real-time signals context switch context switch sigrtmin2_handler() sigrtmin2_handler() (Knows two batches completed) CSE 422S Operating Systems Organization 20
Delivery Order Example Agent (pid 1000) Monitor (pid 2000) Completes Batch 1 sigqueue(2000, SIGRTMIN+2, sigval) Terminates Batch 2 sigqueue(2000, SIGRTMIN, sigval) context switch context switch Kernel sends real-time signals context switch context switch sigrtmin_handler() (Guaranteed to handle terminated batch first!) sigrtmin2_handler() CSE 422S Operating Systems Organization 21