Overview of Smalltalk Programming Language
Smalltalk, a pure object-oriented programming language, was initially developed by Alan Kay and further advanced at Xerox PARC. This versatile language features message passing, object-centric design, and a GUI-based system. Explore its classes, methods, class definitions, and more through the Browser window. Dive into defining new classes and methods, viewing existing classes like Integer, and understanding message passing in Smalltalk.
Download Presentation
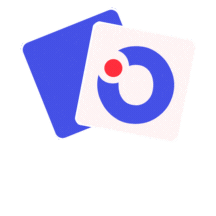
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Smalltalk a Pure (and first) OOPL Smalltalk was developed by Alan Kay as part of his phd dissertation It was further developed by AI researchers at Xerox PARC to construct a GUI-based operating system as well as a graphics language called Turtle Graphics although today Smalltalk has a number of variants, we will limit ourselves to a more recent version called Squeak (an open source product) Pure OOPL everything is an object all data are pointed to by references all communication through message passing messages come in a few forms that make the language look more like non-pure OOPLs that is, message passing doesn t look like message passing in languages like Java
Classes and Methods Examine the definitions for and define classes and methods through the Browser window, there are four top panes that represent (shown on the next slide) categories (name space) classes within the selected category class methods (methods that operate on the class as a whole) instance methods (methods that operate on instances of the class) Selecting any class shows the class definition in the bottom window selecting a category gives you a blank class definition that you can fill in to define a new class: define at least its name and instanceVariableNames (instance data) Selecting any method shows the method s definition in the bottom window selecting a class and clicking in the blank space under the list of methods gives you a blank method to define a new one
Viewing a Class Here, we are viewing the Integer class and looking specifically at how <= is implemented
Defining a Class and Method Here, I have created a new category called MyNameSpace and added a class called MyName (I was going to call it PersonName but had already created a class by that name earlier) To this class, I added four methods as shown on the right, the first method s implementation is shown in the bottom pane
Messages: Binary Called binary because these methods operate on two objects x < 5 pass to x the message < 5 to test to see if the value stored in x is less than 5, returns true or false x == z pass the message equal to z to x, returns true or false a + 1 pass the message add 1 to a, returns the value of a + 1 (a does not change) b * c + d / e several messages passed to b in a left to right manner so this is actually the same as (((b * c) + d) / e), / is used for float division x // y integer division (use \\ for mod)
Messages: Continued Other binary messages include <, >, <=, >=, ==, ~= (not equal), ~~ (not identical), eqv: (equivalent) not, and: (or &), or: (or |), xor: & and | are short-circuited, and: and or: are not when using not, the not comes after the object as in x not (instead of not x because the not message is being passed to x) Some binary messages are of the form object message: object as in x quo: y. (integer divide) x rem: y. (integer remainder) x gcd: y. (greatest common denominator) x roundTo: 2. (round x to 2 places, also truncateTo:) x log: base. (compute log base x) x max: y. (return the maximum between the two values) -5is a unary message (literally passes negate to 5)
Messages: Multiple Parameters For other messages, use the notation object messagename[: parameters]. as in x increment: 5. pass to variable x the message increment: 5 (presumably to add 5 to the value stored in object x) object message: firstparam keyword2: secondparam . here, the parameters are denoted by special keywords Messages can be chained together in which case they operate left to right x abs sqrt factorial. is the same as sqrt(|x|)!
Example Message Passing 3 factorial + 4 factorial between: 10 and: 100. this computes 10 <= 3! + 4! <= 100 Specifically, it works as follows: 3 gets the factorial message, returns 6 4 gets the factorial message and returns 24 6 (from 3 factorial) gets the message + 24 and returns 30 30 gets the message between: 10 and: 100 and returns true Consider: (3 factorial + 4) factorial between: 10 and: 100. 3 factorial + 4 = 10, 10 gets factorial and computes 10!, which is not between 10 and 100 so this returns false All messages return a value of some kind (although the return value doesn t have to be used) All messages end with a period
Local Variables and Assignments To declare variables inside a method, use | | example: | x y z | variables are untyped (they are all references to objects) Assign variables values using one of :=, <- or _ x := 0. y <- x * 5 + 3. z := y raisedTo: 3. strings are available using individual characters are denoted as $char as in $a or $* (which would be * ) LISP-like symbols are available using $ as in $apple $banana
Strings and Characters Strings are placed between single quotes (not double quotes) string messages include isEmpty size at: index (return the character at the given index, indices start at 1, not 0) copyFrom: index to: index indexOf: $char indexOf: $char ifAbsent: [block]. String new: size. (as in String new: 10.) at: index put: character Characters follow $ symbols as in $a for a character messages include isLowercase, isUppercase, isLetter, isVowel, isDigit, isAlphaNumeric, isSeparator, asLowercase, asUppercase, asString, asciiValue
Other Useful Message between: and: as in x between: 5 and: 10. returns true if x stores a numeric value between 5 and 10 isKindOf: className. as in x isKindOf: Number. or name isKindOf: String. isMemberOf: ClassName. respondsTo: messageName. here, we ask if the given message is available for the object example: x respondsTo: sqrt. Others: isNil, positive, strictlyPositive (> 0), even, odd, isLiteral, isInteger, isFloat, isNumber, isUpperCase, isLowerCase
Blocks Blocks of code are placed inside [ ] If there are parameters passed to the block, use [ :params | block code ]. the block returns the last value computed in the block, like LISP Example: [:x | x + 1]. receives a parameter (x) and returns the value + 1 Blocks are typically used in control statements expr ifTrue: [then clause] ifFalse: [else clause] expr ifTrue: [ expr2 ifTrue: [ inner then clause] ifFalse: [inner else clause]] ifFalse: [ expr3 ifTrue: [ inner then clause2 ] ifFalse: [ inner else clause2]]. expr whileTrue: [ while loop body ]. there is also a whileFalse: expr timesRepeat: [ for loop body ].
Input and Output Output is performed through the Transcript object (a window) Pass messages to Transcript Transcript clear. clear the window, also cr (\n) and tab (\t) Transcript show: item output item where item is a variable or a literal string as in hello world string printOn: Transcript alternate way to print to Transcript window To get input, use an assignment statement and the FillInTheBlank message as in x := FillInTheBlank request: Enter your age
Arrays Create an array through var := Array new: size. Assign array elements using array at: put: as in x at: i put: y. (same as x[i] = y) Access array elements using at: without put: as in y := y + x at: i. Initializing an array is done with successive with: parameters var := Array with: value with: value with: value You can iterate through the array elements using var do: [ :index | block of code ] For instance, to output the elements of an array do x do: [ :i | Transcript cr; show i: ].
Other Array Messages includes: value as in x includes: 3. (returns true or false) copyFrom: to: as in y := x copyFrom: 2 to: 8. (copies x[2] through x[8] into y) occurrencesOf: value(counts number of occurrences of value) select: [ a block that contains a test ] y := x select: [:a | a > 0]. copies all elements of x into y which are > 0 reject: [ block ] opposite of select collect: [ block ] returns elements of the array after being processed by the block y := x collect [:a | a + 1]. y will be all elements of x after being incremented by 1
Continued inject: object into: [ block ] this performs a computation on the array based on instructions in the block examples: sum := x inject: 0 into: [:a :b | a + b]. Sums up the values in x max := x inject: 0 into: [:a :b | (a > b) ifTrue: [a]. ifFalse: [c]]. max gets the max value in array x shuffled randomizes elements in the array asSortedCollection sorts array asOrderedCollection same but creates an ordered collection (a flex array) asSet converts array to set
More Types There are bitwise methods available bitAnd:/bitOr:/bitXor: (followed by value to be ANDED/ORED/XORED to) bitShift: (followed by number of bits to shift, negative for right shift) Dictionary using key/value pairs denoted using #key->value example: myDictionary := Dictionary new. myDictionary add: #a->1; add: #b->12; add: #c->5; add: #d- >10; yourself. has many methods such as keyAtValue: ifAbsent:, removeKey: ifAbsent:, includes:, includesKey:, occurrencesOf: value, select, reject, inject, as Array, asOrderedCollection, assortedCollection Date and Time classes Point class (Cartesian point) Drawing classes