Introduction to Swift: Evolution, Overview, and Basic Concepts
Swift, a general-purpose and multi-paradigm programming language, was developed by Chris Lattner in 2010. Its evolution, from the initial release in 2014 to becoming open source in 2015, has led to the upcoming Swift 4.0 release in 2017. This language blends object-oriented principles with functional concepts and offers a static, inferred type system. Key concepts include constants, variables, operators, strings, optionals, control flows, functions, closures, and classes.
Uploaded on Sep 12, 2024 | 0 Views
Download Presentation
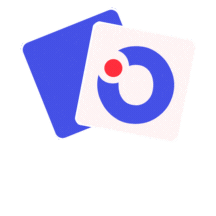
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Lecture 1 - Intro to Swift Ioannis Pavlidis Dinesh Majeti Ashik Khatri
Swift - Evolution Development on Swift began in July 2010 by Chris Lattner, Version 1.0 released on September 9, 2014 Swift became open source on December 3, 2015 Swift 4.0 is expected to be released in Fall 2017
Swift - Overview General-purpose, multi-paradigm, compiled programming language Object-oriented language with functional concepts Typing discipline: Static, Inferred File names end with .swift
Swift Basic Concepts Constants & Variables Operators Strings Optionals Control Flows Functions Closures Classes
Constants and Variables Constants and Variables let maximumNumberOfLoginAttempts = 10 var currentLoginAttempt = 0 let myDoubleArray = [2.1, 3.5, 1.1] Type Annotations var welcomeMessage: String
Operators Supports Arithmetic, Comparison, Logical, Bitwise, & Assignment Operators Supports Ternary Conditional ( Condition ? X : Y ). Unary Plus and Minus
Strings - I String Interpolation let multiplier = 3 let message = "\(multiplier) times 2.5 is \(Double(multiplier) * 2.5)" // message is 3 times 2.5 is 7.5 Can be applied to print statements String Concatenation let str = "Hello" + " swift lovers" // "Hello swift lovers" Methods on string print(str.characters.count) // 18
String - II String Indices str.startIndex // position of the first character of a String str.endIndex // position after the last character in a String let index = str.index(str.startIndex, offsetBy: 7) greeting[index] // will print the 8th character of a String str.substring(to: index) // will print everything up to the 8th character
Optionals? You use optionals in situations where a value may be absent An optional says: There is a value, and it equals x OR There isn t a value at all Example: var serverResponseCode: Int? = 404 Optional variable can be set to nil (absence of a value of a certain type; not a pointer) Example: serverResponseCode = nil nil cannot be used with non-optional constants and variables let possibleNumber = 123 let convertedNumber = Int(possibleNumber) // returns an optional Int or Int?
Control Flows If-else statements if x >= y { print( x is greater or equal to y ) } else { print( y is greater ) } Supports switch statements
Control Flows - unwrapping optionals let possibleNumber = "123" // possibleNumber is type String let convertedNumber = Int(possibleNumber) // convertedNumber is type Int? if convertedNumber != nil { // now that we know that convertedNumber has a value, force unwrap using ! print("convertedNumber has integer value of \(convertedNumber!).") }
Control Flows - Optional Binding - I Use to find out whether an optional contains a value, and if so, to make that value available as a temporary constant or variable Can be used with if and while statements if let actualNumber = Int(possibleNumber) { print("\"\(possibleNumber)\" has an integer value of \(actualNumber)") } else { print("\"\(possibleNumber)\" could not be converted to an integer") } // Prints "123" has an integer value of 123
Control Flows - Optional Binding - II Can also include multiple optional bindings in a single if statement if let firstNumber = Int("4"), secondNumber = Int("42") where firstNumber < secondNumber { print("\(firstNumber) < \(secondNumber)") } // Prints "4 < 42"
Control Flows - Early Exit A guard statement, like an if statement, executes statements depending on the Boolean value of an expression. Unlike an if statement, a guard statement always has an else clause func foo(possibleNumber : String?) { guard let actualNumber = Int(possibleNumber) else { return } print("Number is: \(actualNumber)") }
Control Flows - Loops Swift supports for, for-in, while, and do...while loops for var index = 0; index < 3; index += 1 { } for item in someArray { } while index < 20 { } do { } while index < 20 for i in 0..<3 { /* this will loop 3 times */ }
Functions - I func sayHello(personName: String) -> String { return "Hello, " + personName + "!" } print(sayHello("Bob")) // Prints "Hello, Bob! Supports nested functions Create function inside a function!
Functions - II Functions with Multiple Return Values func minMax(array: [Int]) -> (min: Int, max: Int) { var currentMin = array[0] var currentMax = array[0] // some logic ... return (currentMin, currentMax) } let bounds = minMax([8, -6, 2, 109, 3, 71]) print("min is \(bounds.min) and max is \(bounds.max)")
Closures - I Closures are self-contained blocks of functionality that can be passed around and used in your code { (<parameters>) -> <return type> in <statements> } Functions are special cases of closures global functions have a name and cannot capture any values nested functions have a name and can capture values from their enclosing functions closure expressions don t have a name and can capture values from their context
Closures - II Example let names = ["Chris", "Alex", "Ewa", "Barry", "Daniella"] let sorted = names.sorted() // ["Alex", "Barry", "Chris", "Daniella", "Ewa"] let reversed1 = names.sorted(by: { (s1: String, s2: String) -> Bool in return s1 > s2 }) // ["Ewa", "Daniella", "Chris", "Barry", "Alex"] let reversed2 = names.sorted({s1, s2 in s1 > s2}) // ["Ewa", "Daniella", "Chris", "Barry", "Alex"] let reversed3 = names.sorted(>) // ["Ewa", "Daniella", "Chris", "Barry", "Alex"]
Classes class Person { var name: String var gender = Male var age: Int? init(name: String) { self.name = name } // other functions and variables } let bob = Person(name: Bob ) bob.age = 18
References Swift 3 Programming Language Guide Swift Blog Online Swift REPL