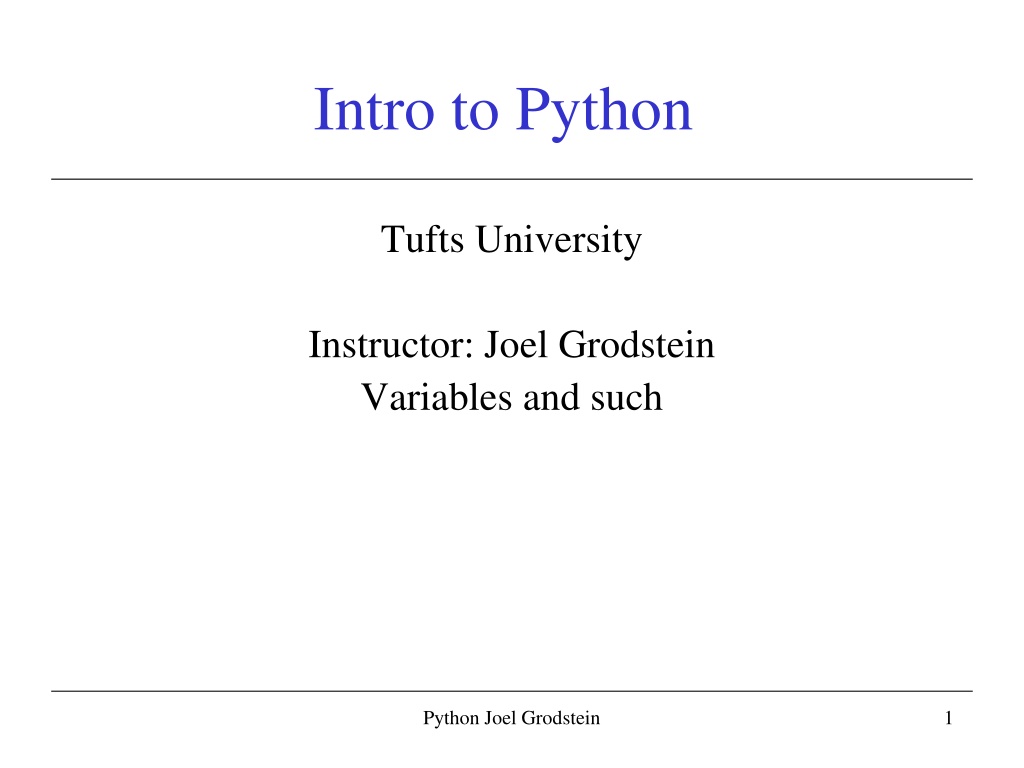
Understanding Variables in Python Programming
Variables play a crucial role in Python programming, serving as little plastic buckets to hold values like numbers or strings. They allow you to assign intuitive names to these values, making your code more readable and flexible. By learning how to work with variables, you gain the ability to store, manipulate, and reuse data effectively in your Python programs. This tutorial delves into the basics of variables, including initialization, value assignment, and the importance of proper variable usage in programming.
Download Presentation
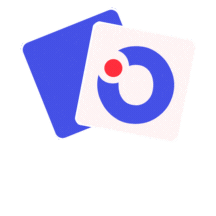
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Intro to Python Tufts University Instructor: Joel Grodstein Variables and such Python Joel Grodstein 1
Variables as little plastic buckets Do a demo with little named buckets as variables Python Joel Grodstein 2
Variables One of the most basic concepts in programming But easy to get very confused for a newcomer Why do we care? What problem(s) do variables solve? They are a place to keep a number (or a string, or most anything) They let you give an intuitive name to a number Python Joel Grodstein 3
The basics sum = 7 sum = 8 sum = 3 + 4 Observations: This is not like algebra. A variable can change its value! Statements come in an order. If we change the order of the statements above, we can leave sum at 8 rather than at 7 sum just holds a number. Assigning it 7 or 3+4 are indistinguishable after the fact Terminology: what we re doing above is called assigning a value to a variable. The first assignment is often called initialization By the way, everything after a # sign is just a comment in Python # Now sum is the number 7. # Now it s not 7 any more! # Now it is 7 again 7 8 U sum Python Joel Grodstein 4
Its really not like algebra Consider this code: i=5 i=i+2 i=i+2 # Pretty obvious # Now i=7 # Now i=9 5 7 U 9 i In algebra i=i+2 would just be impossible Here, it says to First, compute i+2 (i.e., 5+2); it s 7 Next, assign 7 to i The next time, we get 7+2=9 This will turn out to be really useful now computers can count! Python Joel Grodstein 5
Variables save computation sum100_plus_2 = 1+2+3+4+5+ +100 + 2 sum100_plus_3 = 1+2+3+4+5+ +100 + 3 slow sum100 = 1+2+3+4+5+ +100 sum100_plus_2 = sum100 + 2 sum100_plus_3 = sum100 + 3 fast and more clear Reusing a variable means the big long sum is only computed once. And it s more clear (to most people) over the top one? Any advantages of the bottom method Python Joel Grodstein 6
Computers are very picky! What s wrong with this code? sm5 is uninitialized What happens? In some languages (including Python), accessing an uninitialized variable is illegal In some languages (e.g., C++) it s unpredictable Some languages start all variables with some default (e.g., 0) sum5 = 1 + 2 + 3 + 4 + 5 sum5_plus_1 = sm5 + 1 Python Joel Grodstein 7
Variables help clarity x=19 y=x*12 z=x*365 What does this code do? age_in_years = 19 age_in_months = age_in_years*12 age_in_days = age_in_years*365 Which version is more clear? age_in_years = 19 age_in_days = age_in_years*12 age_in_months = age_in_years*365 Nice descriptive names are not real helpful if they re wrong! Python Joel Grodstein 8
Another benefit of variables age_in_years = age_in_months = age_in_years*12 age_in_days = age_in_years*365 18 19 age_in_years = 19 age_in_months = 19*12 age_in_days = 19*365 Any benefits of the top version over the other one? With just a few keystrokes, you can change 19 to 18 or 20, and re-run the program Python Joel Grodstein 9
Strings Variables do not have to just be numbers. Strings work too message = 'Hello world' print(message) prints out Hello world Basically, a string is any collection of characters (letters, numbers, punctuation, etc) Python Joel Grodstein 10
Fun with strings You can do lots of things with strings name = 'jiminy cricket' name = "jiminy cricket" name = "jiminy cricket' name = "O'hare airport" len ('jiminy') len (name) # No different # Not legal! # Works as expected # 6 # 14 ("O'hare airport") len() is a function More on that later Python Joel Grodstein 11
Comments What's with all of the '#' characters? PI = 3.14159 Computer programs can get big. And complicated And confusing Trying to understand somebody else s program can be difficult, and frustrating You could just ask them for help They might not like that They may have graduated 5 years ago When you write code, sometimes a word (or sentence, or paragraph) of explanation for the uninitiated is a really good idea Comments let you do that In some of our HWs, you will have to take code that I've written and add to it My code is liberally commented (If you still don't understand it, it's OK to ask me) # March 14 is pie day Python Joel Grodstein 12
Comments What do these do? PI = 3.14159 # PI = 3.14159 # This one assigns 3.14159 to PI # This one does nothing at all! Python Joel Grodstein 13
Expressions a = 2+3*5 a = (2+3)*5 a =7 % 4 a = 2**3 a=pow(2,3) b = 'ab'+'cd'+'ef' c='12'+b+'gh' b = 3 * 'ab' b = 3 + 'ab' # yields 17 # yields 25 # yields 3 (modulus, or remainder) # yields 8 # also yields 8 # yields 'abcdef' # yields '12abcdefgh' # yields 'ababab' # Illegal Python Joel Grodstein 14
More picky rules Names of variables are case sensitive Size=4 a = size*2 Names cannot begin with numbers or special characters 3squared = 3*3 Squared3 = 3*3 Names can never have a special character three^2 = 3*3 three_sqr = 3*3 threeSqr = 3*3 # error # Illegal # Legal # Illegal # Legal. _ is OK # Another common usage Python Joel Grodstein 15
So many little rules If you don t learn all of these picayune little rules now, you ll discover them on your own (one by one) when you try to write your programs Your programs will break for inexplicable reasons (well, inexplicable to you, anyway) You might suffer from death by 1000 paper cuts Or you might just google these rules and find them out yourselves Or you might even get motivated to read the documentation! (see the syllabus for details) Python Joel Grodstein 16
Printing All of this computation is only useful if you can see what it did . So let s talk about printing Python can print in several ways Python Joel Grodstein 17
print print() is a function that prints things. print(3) # prints 3 print(3+5) # prints 8 print('a=',a) # prints a= 3 (or whatever the print (3, 5) # 3 5 Python can print with fancy formatting (e.g., remove the space, centered in an 8-wide field, ) Not needed in this course Google Python string.format() for details variable a actually is) Python Joel Grodstein 18
Python statement syntax More detail when we talk about loops. For now No spaces at the beginning of a line before a statement One statement cannot span multiple lines Except if you end a line with '\' Or after a comma inside parentheses OK bad a=3 b=4 a=3 + 4 bad OK a=3 + \ 4 print ('a=', a) Python Joel Grodstein 19
Python statement syntax Most languages are free form; they ignore spaces and newlines Except Python High on the list of the best things about Python Also high on the list of the worst things about Python sort of a religious dispute Python Joel Grodstein 20
Functions We ve already used them; let s explain them just a bit n_letters = len ('jiminy') a=pow(2,3) print ('jiminy , 3) A function might compute something and return it to you Or it might have a side effect like printing something It has zero or more parameters Later on you ll write your own functions Python Joel Grodstein 21
Group activity Running Thonny in class In lab, we have the lab machines But today we don t! Course webpage shows you how to install Thonny on your laptop Using the Tufts Virtual Desktop Point a browser at https://vdi.it.tufts.edu/ Choose either run-in-browser or native client, log in with Tufts ID Pick Engineering lab, not TTS Virtual Lab Run Anaconda3/Thonny Python Joel Grodstein 22
Group activity What do these do? a = 4+3*5 a = (2+4)*5 b = 'ab'+'cd'+'ef' b*2 print (b,b) print (b, 'x'+'y') h=2; print (h, 'heads is',h*'head ) # sets a to 19 # sets a to 30 # sets b to 'abcdef' # yields 'abcdefabcdef ' # prints abcdef abcdef # prints abcdef xy # prints 2 heads is headhead Python Joel Grodstein 23
Follow-up activities Try the examples from this lecture yourself Vary them, or even mis-type some to see what happens More exercises. Write a program that takes the radius r of a circle and computes the area takes a number n and computes the value of n+ n*n + n*n*n Start with a variable name and print a banner: ********** *Fred * ********** Read a bit from any of the Python resources in the syllabus Python Joel Grodstein 24