Understanding Variables and Control Structures in JavaScript
Introduction to JavaScript variables including integers, floats, strings, booleans, and arrays. Learn about control structures like if-else statements, comparison operators, and loops. Practice examples provided for better understanding.
Download Presentation
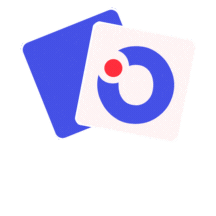
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Lecture 02 Javascript
Variables var
Variables var variableName
Integer var myInteger = 1;
Integer var anyOtherName = 1;
Float var myFloat = 1.00;
Float var myFloat = 1.50;
String var myString ="hello";
Boolean var myBoolean = true;
Boolean var myBoolean = false;
if(a < b){ console.log("a is less"); }else if(a > b){ console.log("a is more"); }else{ console.log("they must be equal"); } a == b a != b && a === b ||
Array var myArray = [1,2,3];
Array var myArray = [1,2,3,4.0];
Array var myArray = [1,2,3,4.0,"hello"];
Array var myArray = [1,2,3,4.0,"hello"]; myArray[0] \> 1
Declare var myArray = []; Populate myArray[0] = 102; Or myArray.push(102); Fits the new value in the first available position
for(var i=0; i<10; i++){ console.log(i); } Execute with var i=0
1 Is minor then ten? 0 for(var i=0; i<10; i++){ console.log(i); } Execute with var i= And so on
var myArray = [1,2,3,4.0,"hello"]; for(var i=0; i<10; i++){ console.log(myArray[i]); } 1 2 3 4.0 "hello"
function myFunction(myArgument){ console.log(myArgument) }
var x = function f(a){ return a + 5; } x(2); 7 var myArray = [1,2,3,4.0,"hello",x]; myArray[5](2); 7
Recap on if Statement Booleans == === != <=
Good to know * / %
1 Write a function that: Takes two arguments a and b subtracts the smaller from the bigger returns the result
2 Declare an array of strings Write a function that: Iterates through the array Checks if hello is in the array In case it is, prints Hi back
3 Declare an array of booleans Write a function that: Iterates through the array For each element: -Prints TRUE if the boolean is true -Prints FALSE if the boolean is false
4 Declare an array of integers Write a function that: Iterates through the array Prints only the even numbers
Declare an array of integers Calculate the average of the array entries 6 *Tip use myArray.length to get the number of elements in the array Write a function that iterates through the array and prints BIGGER If the current value is bigger then the average, SMALLER if the value is smaller and EQUAL if it is equal
Declare an array of integers 5 Write a function that: Iterates through the array For each value of the array, populates a new array with that value only in case it is: -bigger than 5 -smaller than 9 In case numbers are smaller than 5 prints too small In case numbers are bigger than 9 prints too big Returns the array
Objects var myObject = { key_1:"value", key_2:"value", key_3:"value", };
Objects var myObject = { key_1:"string", key_2:1, key_3:[array ], };
Objects var myObject = { key_1:"string", key_2:1, key_3:[array ], key_4:function(){ . . . }, };
Data Driven Documents
Import D3 <script src="https://d3js.org/d3.v5.min.js"></script>
D3 in action d3.select("body").append("svg") .append("circle") .attr("cx",100) .attr("cy",100) .attr("r",20);
var mySvg = d3.select("body") .append("svg") mySvg.append("circle") var myCirc = .attr("cx",100) .attr("cy",100) .attr( r",20); myCirc