Understanding Fortran Templates and Compiler-Driven Design Goals
Delve into the world of Fortran templates through a primer on generics, showcasing design goals set by the compiler. Explore syntax examples and motivating examples such as the AXPY subroutine, offering insight into the self-consistent nature of templates and their flexibility in parameter combinations.
Download Presentation
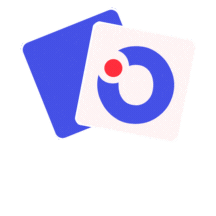
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Fortran Templates A Primer J3 Generics Subgroup
Template Design Goals Compiler ensures the template is: Self consistent Specifies what makes a valid combination of parameters Work with derived and intrinsic types Template doesn t dictate spelling
Some Example Syntax REQUIREMENT fizz(T, buzz) TYPE, DEFERRED :: T FUNCTION buzz( ) END FUNCTION END REQUIREMENT TEMPLATE foo(bar, baz, ) REQUIRES fizz(bar, baz) END TEMPLATE INSTANTIATE foo(integer, operator(+), ), ONLY: a, b => c,
A Motivating Example: AXPY subroutine axpy(a, x, y) real, intent(in) :: a real, intent(in) :: x(:) real, intent(inout) :: y(:) y = a * x + y end subroutine
A Motivating Example: AXPY (cont) template axpy_tmpl(k) integer, parameter :: sp = kind(1.0), dp = kind(1.d0) private instantiate axpy_tmpl(sp) public :: axpy instantiate axpy_tmpl(dp) integer, constant :: k real(sp) :: a, x(10), y(10) interface axpy real(dp) :: da, dx(10), dy(10) procedure axpy_ end interface call axpy(a, x, y) contains call axpy(da, dx, dy) subroutine axpy_(a, x, y) real(k), intent(in) :: a real(k), intent(in) :: x(:) real(k), intent(inout) :: y(:) y = a * x + y end subroutine end template
A Motivating Example: AXPY (cont) template axpy_tmpl(T, plus, times) requirement bin_op(T, op) private type, deferred :: T integer, parameter :: sp = kind(1.0), dp = kind(1.d0) public :: axpy elemental function op(a, b) instantiate axpy_tmpl(real(sp), operator(+), operator(*)) requires bin_op(T, plus) type(T), intent(in) :: a, b instantiate axpy_tmpl(real(dp), operator(+), operator(*)) requires bin_op(T, times) type(T) :: op instantiate axpy_tmpl(integer, operator(+), operator(*)) interface axpy end function real(sp) :: a, x(10), y(10) procedure axpy_ end requirement real(dp) :: da, dx(10), dy(10) end interface integer :: ia, ix(10), iy(10) contains subroutine axpy_(a, x, y) call axpy(a, x, y) type(T), intent(in) :: a call axpy(da, dx, dy) type(T), intent(in) :: x(:) call axpy(ia, ix, iy) type(T), intent(inout) :: y(:) y = plus(times(a, x), y) end subroutine end template
A Motivating Example: AXPY subroutine axpy(a, x, y) real, intent(in) :: a real, intent(in) :: x(:) real, intent(inout) :: y(:) y = a * x + y end subroutine
A Motivating Example: AXPY (cont) template axpy_tmpl(T, U, V, plus, times) requirement bin_op(T, U, V, op) integer, parameter :: sp = kind(1.0), dp = kind(1.d0) private type, deferred :: T, U, V instantiate axpy_tmpl( & public :: axpy elemental function op(a, b) real(sp), integer, real(dp), operator(+), operator(*)) requires bin_op(T, U, U, times) type(T), intent(in) :: a real(sp) :: a requires bin_op(U, V, V, plus) type(U), intent(in) :: b integer :: x(10) interface axpy type(V) :: op real(dp) :: y(10) procedure axpy_ end function end interface end requirement call axpy(a, x, y) contains subroutine axpy_(a, x, y) type(T), intent(in) :: a type(U), intent(in) :: x(:) type(V), intent(inout) :: y(:) y = plus(times(a, x), y) end subroutine end template