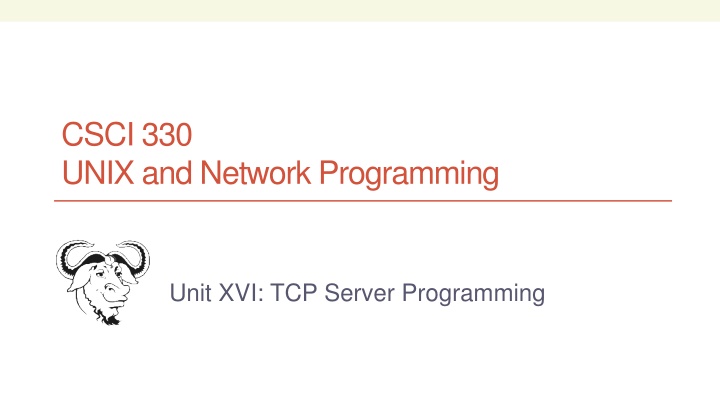
TCP Server Programming Overview in CSCI 330
Explore TCP server programming concepts in CSCI 330 Unix and Network Programming, covering topics like TCP client-server communication, DNS lookup, forking processes, listing files, and socket system calls. Learn about creating communication endpoints, binding addresses, accepting connections, and sending/receiving data over TCP connections. Dive into illustrations of TCP server and client interactions, along with basic client request handling. Review the logic behind a TCP server's continuous operation and forking to service client requests.
Download Presentation
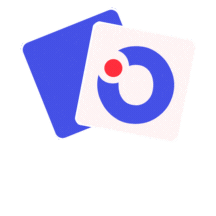
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
CSCI 330 UNIX and Network Programming Unit XVI: TCP Server Programming
CSCI 330 - UNIX and Network Programming 2 Unit Overview TCP client & server programming add DNS lookup: basicClient server fork to process client request example TCP server list files in directory specified
CSCI 330 - UNIX and Network Programming 3 TCP programming provides multiple endpoints on a single node: port common abstraction: socket socket is end-point of communication link identified as IP address + port number can receive data can send data
CSCI 330 - UNIX and Network Programming 4 Socket system calls client server Primitive Meaning socket Create a new communication endpoint bind Attach a local address to a socket listen Announce willingness to accept connections accept Block caller until a connection request arrives connect Actively attempt to establish a connection write Send(write) some data over the connection read Receive(read) some data over the connection close Release the connection
CSCI 330 - UNIX and Network Programming 5 TCP server illustration
CSCI 330 - UNIX and Network Programming 6 TCP client illustration
CSCI 330 - UNIX and Network Programming 7 Illustration: Basic TCP request client
CSCI 330 - UNIX and Network Programming 8 Review: TCP Server basic logic while (true) { connSock = accept(sock, ...); // via connSock // process client s request close(connSock); }
CSCI 330 - UNIX and Network Programming 9 TCP Server fork server starts loop blocks on accept for connection from client after accept: accept returns dedicated connection socket server forks to service client request parent process closes dedicated connection socket continues to block for next accept child process serves client request communicates with client via dedicated connection socket
CSCI 330 - UNIX and Network Programming 10 TCP Server fork: logic while (true) { connSock = accept(sock, ...); if (fork()) { close(connSock); } else { // process client s request via connSock ... } } // parent process // child process
CSCI 330 - UNIX and Network Programming 11 TCP server/fork illustration
CSCI 330 - UNIX and Network Programming 12 TCP server/fork illustration
CSCI 330 - UNIX and Network Programming 13 Server example: list directory after accept, server forks to service client request parent process will continue to block on new accept child process serves client request open directory read directory entries
CSCI 330 - UNIX and Network Programming 14 Server child: processClientRequest
CSCI 330 - UNIX and Network Programming 15 TCP server: opendir detail // open directory DIR *dirp = opendir(path); if (dirp == 0) { // duplicate socket descriptor into error output close(2); dup(connSock); perror(path); exit(EXIT_FAILURE); }
CSCI 330 - UNIX and Network Programming 16 TCP server: readdir detail while ((dirEntry = readdir(dirp)) != NULL) { strcpy(buffer, dirEntry->d_name); strcat(buffer, "\n"); size = strlen(buffer); if (write(connSock, buffer, size) != size) { perror("Mismatch in number of bytes"); exit(EXIT_FAILURE); } cerr << "sent: " << buffer; }
CSCI 330 - UNIX and Network Programming 17 Summary TCP server programming server fork to process client request