Python Modules and Functions
Python modules and functions play a crucial role in code organization and reusability. Modules are files containing Python code, while functions are blocks of organized, reusable code. By importing modules and calling functions, you can enhance modularity and efficiency in your applications. Explore how to create, import, and use modules along with defining functions. Discover the power of built-in functions like pow() and math functions for advanced calculations.
Download Presentation
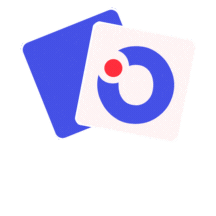
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Module A module is a file consisting of Python code. A module can include runnable code. A module can define functions and classes. Functions need to be called to run. The import Statement: We can use any Python source file as a module by executing an import statement to import the module into Python modules that need that module. The import has the following syntax: import module1[, module2[,... moduleN]
Example of import a runnable Python module #This is grade.py try: score= float(input("Enter exam score: ")) if score < 60: grade="F" elif score < 70: grade="D" elif score < 80: grade="C" elif score < 90: grade="B" else: grade="A" print("Your grade is: " + grade) except: print("Enter digits only!") #This module needs to run grade.py import grade firstName=input("Enter first name:") lastName=input("Enter last name:") fullName=firstName + lastName print("Full name is:" + fullName) #import module4 Note 1: The import statement typically is placed at the top of the module, but can be inserted at any point where needed. Note 2: import grade, not import grade.py
myFiancialService.py: A module with two functions and can be imported to other module def futueValue(presentValue,rate,year): futureValue=presentValue*(1+rate)**year return futureValue def monthlyPayment(loan,rate,term): pmt=(loan*rate/12)/(1-(1+rate/12)**(-12*term)) return pmt
Function A function is a block of organized, reusable code that is used to perform a single, related action. Functions provide better modularity for your application and a high degree of code reusing. built-in functions: no need to import pow(2,3), input(), print() Python math functions module: need to import math module Import math user-defined functions.
Built-in Functions https://docs.python.org/3/library/functions.html#bin Examples: Int(), float(), print(), pow(2,3), str(), round(12.3456,2) ->12.35 round(12.3416,2) ->12.34
Python math module https://docs.python.org/3/library/math.html Examples: math.ceil(x) Return the ceiling of x, the smallest integer greater than or equal to x. import math math.ceil(2.1) 3 math.floor(x) Return the floor of x, the largest integer less than or equal to x. math.floor(2.9) 2 math.sqrt(x) Return the square root of x.
User-Defined Functions Function blocks begin with the keyword def followed by the function name and parentheses ( ). Any input parameters or arguments should be placed within these parentheses. The code block within every function starts with a colon (:) and is indented. The statement return [expression] exits a function: Value-returning function: passing back an expression to the caller. Void-function: A return statement with no arguments is the same as return None (or no return statement). def functionname( parameters ): code block return [expression]
A function may be placed in a module with the calling program. To call a function that returns a value: variableName=function(parameters) def fullName(firstname,lastname): fullName=firstname+ " " + lastname return fullName fname=input("Enter first name: ") lname=input("Enter last name: ") fullname=fullName(fname,lname) print(fullname) Note 1: Place the function before the program. Note 2: Positional parameters: No need to specify the parameter name before specifying the parameter value.
Keyword Arguments: We can also send arguments with the argument = value syntax. def fullName(firstname,lastname): fullName=firstname+ " " + lastname return fullName fname=input("Enter first name: ") lname=input("Enter last name: ") fullname=fullName(fname,lname) print(fullname) fullname=fullName(lastname=lname, firstname=fname) print(fullname)
A function is not required to return a value (void function) To call a function that does not return a value: function(parameters function(parameters) ) def printfullName(firstname,lastname): fullName=firstname+ " " + lastname print(fullName) ### No return statement # return ### or return with no expression fname=input("Enter first name: ") lname=input("Enter last name: ") printfullName(fname,lname)
A function is not required to have parameters def print5stars(): print('*****') print5stars()
Local variable: A variable declared inside the function's body is known as a local variable. It does not exist outside the function. def sum2(): num1=float(input('Enter num1:')) num2=float(input('Enter num2: ')) sum=num1+num2 print('The sum of {} and {} is {}.'.format(num1, num2,sum)) sum2() print('The sum of {} and {} is {}.'.format(num1, num2,sum)) ***error
We can have more than one function in a module def fullName(firstname,lastname): fullName=firstname+ " " + lastname return fullName def sumTwo(num1,num2): sum=num1+num2 return sum fname=input("Enter first name: ") lname=input("Enter last name: ") fullname=fullName(fname,lname) print(fullname) print('First name is' + fname) n1=float(input('Enter num1: ')) n2=float(input('Enter num2: ')) sum=sumTwo(n1,n2) print (sum)
Several Functions may be placed in a module as a package and can be imported to other module. Create a module called: myFinancialService that offer these functions: futureValue: compute the future value taking present value, interest rate and year as arguments. monthlyPayment: compute the monthly payment taking loan, rate and term as arguments.
myFiancialService.py def futueValue(presentValue,rate,year): futureValue=presentValue*(1+rate)**year return futureValue def monthlyPayment(loan,rate,term): #pmt=(loan*rate/12)/(1-(1+rate/12)**(-12*term)) pmt=(loan*rate/12)/(1-pow(1+rate/12,-12*term)) return pmt
The module that uses functions in the myFiancialService module must use the import statement to import module import myFinancialService as mf loan=float(input("Enter loan:")) rate=float(input("Enter rate(4.5% entered as 0.045):")) term=float(input("Enter term in year:")) pmt=mf.monthlyPayment(loan,rate,term) output="With ${:,.2f} loan, {:.2%} rate, {} years term, the monthly payment is:${:,.2f}." print(output.format(loan,rate,term,pmt)) Note 1: import myFinancialService without the .py extension. Note 2: The as keyword defines an alias for the package name.
We may pass function name as argument def futureValue(presentValue,rate,year): futureValue=presentValue*(1+rate)**year return futureValue def monthlyPayment(loan,rate,term): pmt=(loan*rate/12)/(1-pow(1+rate/12,-12*term)) return pmt def useMyFun(funcName,amount,rate,term): outValue=funcName(amount,rate,term) return outValue value=float(input("Enter a value: ")) rate=float(input("Enter annual interest rate (3.5% entered as 0.035):")) term=float(input("Please enter the term (in years):")) fv=useMyFun(futureValue,value,rate,term) print('Future value is: ${:,.2f}'.format(fv)) payment=useMyFun(monthlyPayment,value,rate,term) print('Monthly payment is: ${:,.2f}'.format(payment))
Exercise Add a goalSeek function to the myFiancialService.py module that takes present value, interest rate and target future value as input, and compute and return the number of year to reach the target.
Python Lambda function A lambda function is a small anonymous function. A lambda function can take any number of arguments, but can only have one expression. Syntax: lambda arguments : expression Example: a, b, c are arguments x = lambda a: a + 10 print(x(5)) x = lambda a, b : a * b print(x(5, 6)) x = lambda a, b, c : a + b + c print(x(5, 6, 2))
You can apply the function to an argument by surrounding the function and its argument with parentheses: >>> x = (lambda a, b, c : a + b + c)(2,4,6) >>> print(x) 12
Why Use Lambda Functions? The power of lambda is better shown when you use them as an anonymous function inside another function. Say you have a function definition that takes one argument, and that argument will be multiplied with an unknown number: def myfunc(n): x=lambda a : a * n return x mydoubler = myfunc(2) # mydoubler now is a lamda function a:a*2 print(mydoubler(11)) mytripler = myfunc(3) # mytripler now is a lamda function a:a*3 print(mytripler(11))
Example def decimals(n): myformat='${:,.'+str(n)+'f}' return lambda a: myformat.format(a) my2=decimals(2) ## create a lambda function my3=decimals(3) x=123.456789 y=my2(x) z=my3(x) print(y) print(z)