Overview of Pascal Programming Language
Pascal is an offshoot of Algol-W, designed as a teaching language for Computer Science majors to write structured code without GO TO statements. It offers nested subroutines, limited variable types, and a non-case-sensitive approach. Program structure in Pascal involves main programs and procedures/functions, with declarations, assignments, and blocks defined in a specific manner. The language is not suited for large projects but is readable and writable for small-scale applications.
Download Presentation
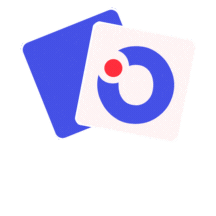
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Pascal Offshoot of Algol-W (which itself was a descendant of Algol 68) named after mathematician Blaise Pascal, inventor of first mechanical calculator Intended to be used as a teaching language for CS majors to write structured code (no GO TO statements) not intended for commercial/industrial use because of limitations that made the language safe but not flexible very readable, writable for small projects, not for large projects Most interesting features are nested subroutines C-languages do not have these scope rules due to nested subroutines non-local variables are not necessarily global variables Other differences from C-languages declarations are written backward parameter passing simplified
Pascal Program Shape All programs start with Program name Programs broken into main program and procedures/functions main program code is a block of code corresponding to the program, that is, not in a procedure or function Procedure and function headers are of the form procedure name(params) function name(params): returntype no prototypes used since program code cannot be compiled separately All blocks placed inside of begin end structures no specific end statements (e.g., endif, endfor) Skeleton Pascal program Program name // main var // declarations Procedure procname( ) // proc vars begin // proc //code here end; begin // main prog // code here end.
Variables Pascal s types are limited to integer, real (float), char, boolean, file (like FILE * in C) data structures are records (struct), arrays, set (built-in type), strings (arrays of chars) enumerated types are available including subranges of ordinal values type days = (Sun, Mon, Tue, Wed, Thu, Fri, Sat); weekdays = Mon .. Fri; var anyday: days; workday: weekdays; pointer build data structures using arrays and/or pointers and the other more basic types you might define a record for a node that consists of some datum and pointer field and then build a linked list out of nodes All variables are typed and compiling time type checking is extenstive but Pascal is not strongly typed because of variant records Pascal is a non-case-sensitive language, X and x are the same
Declarations and Assignments Declarations are placed before executable code start with the word var, syntax is var name : type; var x , y : integer; you can initialize a variable by placing = value after the type as in age : integer = 53; notice you cannot declare and initialize multiple vars on one line! constants are defined the same way but follow the word const instead of var all vars are declared before the begin statement for the procedure/function/program Assignment statements only permit single assignments Use := X := X+1; Y := 0; Z := call_some_function(X,Y)*2;
Functions and Procedures Declared with the word function or procedure preceding the name as in function f1( ) : return returntype; the function must end with an assignment statement assigning f1 as in f1 := x + y procedure p1( ); Subroutines must be embedded within a program (recall slide 2) a subroutine may be invoked by code beneath it only (see next slide) Parameters are listed in two ways: name : type pass by copy (just like in C) var name : type pass by reference (parameter passed is the address of the original datum) unlike in C, you do not explicitly dereference the pointer in the subroutine, the Pascal compiler will insert the appropriate dereferencing for you C does not use pass by reference, you can only simulate it by passing a pointer
Program main; var x : integer; procedure p1( ); var x : integer; procedure p2( ); var x : integer; procedure p3( ); var y : integer; begin // reference to x end; begin end; begin end; procedure p4( ); begin end; begin end. Scope Rules Subroutines are nested inside of the program and each other A variable declared in an outer subroutine (or the program itself) is accessible to anything nested inside of it to determine scope find the inner-most declaration to the right, x in P3 is that of P2 while x in P4 is that of the main program there is no way to reference p1 s x from p2 there is no way to reference p3 s y from p2 The main program can call any procedure p4 can call p1, p2 and/or p3 p1 can call p2 and/or p3 p2 can call p3 // reference to x
Selection Statements and Loops If-then, if-then-else, nested if-then-else requires the reserved word then no ( ) for the condition any clause (then or else) of more than 1 statement needs to be placed inside of a begin end block Case: like C s switch except no need for break statements Three types of loops for loop: for var := first to|downto last do only counts up by 1 or down by 1 while-do: while condition do statement; multiple statements require begin end repeat-until: repeat statement(s) until condition; repeats while condition is false, no begin end needed Pascal has break and continue similar to Java/C, plus a goto statement
I/O uses crt; - command to permit output to monitor I/O commands are write/writeln, read/readln readln(a, b, c); writeln( the output is , a, b, c); follow variable name with :size or :size:decimal to format reals, as in x:5:2 I/O from/to disk file requires a variable of a type of file type intfile = file of integer; var file1: intfile; Assign the file to the variable assign(file1, filename ); rewrite(file1); // used when outputting to the file Access the file through your readln/writeln, file variable being the first param writeln(file1, a, b, c); Close the file using close(file1);
Strings and Arrays Strings are built-in for some versions of Pascal in others, you have to declare the String to be an array of packed chars Several built-in string operations assigned directly using := compared directly using <, =, >, <>, <=, >= UpperCase, LowerCase, Length Pos(substring, string) returns the index in string of where it matched substring Copy(string, position, length) returns the substring of string starting at position of length characters Str(a, s) converts numeric value in a to string s (a can only contain digits, a minus sign, a decimal point) Val(s, a, er) converts string s into numeric variable a, er is the index of a character that could not be converted if an error arises Arrays are declared as type name = array [startindex .. endindex] of type; once the type is defined, use the type to declare your array variable if you want to start at 1, just include the endindex indices can be any ordinal type, e.g., character or days from earlier in the slides
Pointer and Boolean Types Pointers declared as types as in type intPtr = ^int; var ip : integer; use ^ to dereference the pointer as in ip^ := 0; use @ to obtain an address as in ip := @x; a pointer that is not pointing at anything will have the value nil (not null/NULL) modest pointer arithmetic is available in Pascal you can change a pointer by adding or subtracting to it or using increment and decrement (built-in operations for variables of ordinal types) Booleans (store true, false, not an int value) cannot be directly input or output AND, OR and NOT are indicated using the words rather than &&, ||, ! as in if A AND NOT B then AND and OR are not short-circuited, to perform short-circuiting use these variants: AND THEN, OR ELSE
Building Data Structures Create a record for the type of data to be stored e.g., student records To build the data structure create a type that will be an array of the record type or create a record that will store a student record and a next pointer and build a linked list or tree out of the nodes Pascal has a weak form of encapsulation: the unit the unit allows for separate compilation as long as the unit is compiled prior to the driver code the unit has an interface portion for the public components, and an implementation portion for the private components, in a single file unlike Modula-2 in which ADTs are pointed to, the unit is a data structure whose size is determined at compile time change the unit and you have to recompile both the unit and any programs that use the unit
Modula-2 and Modula-3 An offshoot of Pascal but built for software development Syntactically, very similar (see next slide) but, the compilation unit is a module and can be separately compiled the module allows encapsulation you can import an entire module or specific parts of a module (e.g., specific procedures/functions) the module provides abilities to more precisely control information hiding by adding export statements (export a; would make a visible external to the module) Modula-2 was not particularly successful as an industrial-used language, partially because of the successes of C/C++ and Ada Modula-3 was released as a superior follow-up to Modula-2 but was not intended for use in industry it added to M-2 by having templates (generics), multithreading capabilities, exception handling capabilities using try except blocks, and classes
Modula-2 vs Pascal Modula-2 is very similar syntactically to Pascal with the following differences reserved words must be upper case and identifiers are case sensitive strings can be denoted using either or END statements for records and procedures/functions have to include the name as in END function1; or END My_Record_Type; assigning a pointer uses the notation POINTER TO var multidimensional arrays are denoted using x[1..10],[1..5] nested if-then-else use the term ELSIF instead of ELSE IF for loop differs by allowing an explicit step size as in FOR i := 1 TO 9 BY 2 DO there is an infinite loop statement LOOP END (must exit using an EXIT statement as in LOOP IF THEN EXIT END; END;, there is no GOTO statement in Modula-2 I/O operations are defined entirely through library procedures, not built-in operations
Ada As stated earlier, Ada s development was one of the largest planning projects in programming language development history Ada was developed by the US dept of defense to be the only language used for all employees and contractors of the US govt syntactically, it would look somewhat like Pascal including nested subroutines but Ada was a much larger language and far more complex than Pascal it would contain many features Pascal did not contain its implementation is deeply flawed in that some of the features are not comparable to other languages of that time or later revisions of Ada have tried to match the development of such languages as C++, but poorly the DoD stopped requiring the use of Ada in 1997
Ada vs Pascal Syntactically, Ada looks more like Pascal than other languages Similarities include same control statements except Ada has an infinite loop and no repeat-until both languages are close to strongly typed (Ada more so than Pascal) but Ada requires explicit conversion of values if multiple types are in one expression (e.g., adding an int and a float require converting the int to a float) both languages are case insensitive the ; is used differently, in Pascal it separates instructions, in Ada it ends instruction (like C) But aside from the syntactic similarities, there are a lot of differences as Pascal was developed as a safe , teaching language Ada was developed to be used for largescale software development
Continued Differences include Ada permits function names being passed as parameters Ada has keyword and optional parameters and permits parameter initialization Ada uses Packages for ADT definitions/encapsulation/information hiding and supports separate compilation of code Ada array sizes do not have to be specified at compile time if using a flex array Ada has exception handling capabilities including built-in exceptions Pascal s only form of exception handling is EOF and EOLN Ada has a type of generic available for subroutines as well as method overloading in Ada 83, these are not objects (as seen in Java) but instead ADT types Ada s parameter passing implementation is compiler-dependent Ada has instructions available for doing software validation
Problems with Ada Ada contains features that Pascal does not include because Pascal was designed to be a safe language, Ada was not Pascal was designed for teaching and Ada for software development Ada does not have built-in I/O operations instead, you must use library packages (similar to C) GET, PUT input one item, output one item (overloaded so they can be used on different types) Ada compilation is slower than many other language compilers due to extensive type checking In comparison to C++, Ada is less flexible but as complicated