Object-Oriented Design
The fundamentals of object-oriented design with insights and practical examples. Dive into concepts such as classes, inheritance, and polymorphism to build robust and scalable software solutions. Understand the importance of encapsulation and abstraction in designing flexible and maintainable code. Learn how to apply these principles to create modular and reusable software components for efficient development workflows. Enhance your programming skills and elevate your understanding of object-oriented design practices.
Download Presentation
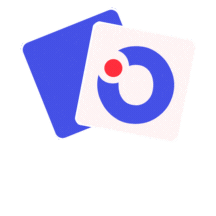
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
A Historical Perspective Programs Procedures to manipulate data Earlier: procedural Think actions/procedures first, data second 1970 s: object-oriented Think data/objects first, procedures/actions second Different perspectives on how to organize problem solving and hence how to write programs
Object-oriented (OO) Design What are the different kinds of objects/data? Design decision on how data are organized OO terminology: class, object Example: Class Person: object mary, object john Class Building: object empireStateBuilding, object olinEngineering 1.
Object-oriented (OO) Design What are the different kinds of objects/data? Design decision on how data are organized OO terminology: class, object Example: Class Person: object mary, object john Class Building: object empireStateBuilding, object olinEngineering 1. How can the objects/data be manipulated? Design decision on how data can be manipulated OO terminology: method (action) Example: Class Person: method getAddress, method changeAddress Class Building: method getAddress empireStateBuilding.getAddress() john.changeAddress(newAddress) 2.
OO vs Procedural Design Depending on the problems OO is more appropriate Many different types of data Many interactions among different types of data Procedural is more appropriate Few types of data Few interactions among different types of data
OO and Java Defining a class In one .java file Class name is the same as the file name E.g. class Person is defined in Person.java Each class has attributes (instance variables) E.g. class Person has attributes: name, address, phone
OO and Java Defining a class In one .java file Class name is the same as the file name E.g. class Person is defined in Person.java Each class has attributes (instance variables) E.g. class Person has attributes: name, address, phone Defining methods Methods are defined within a class I.e. can t define a method without a class Name, parameters, return type
class Person public class Person { private String _name; // attributes (instance variables) private String _address; private int _phone; }
class Person public class Person { private String _name; // attributes (instance variables) private String _address; private int _phone; public Person(String name, String address, int phone)//constructor { _name = name; _address = address; _phone = phone; } }
class Person public class Person { private String _name; // attributes (instance variables) private String _address; private int _phone; public Person(String name, String address, int phone)//constructor { _name = name; _address = address; _phone = phone; } public String getName() // method { return _name; } }
Class PersonTest import java.util.*; class PersonTest { public static void main(String[] args) { // object jane created from class Person Person jane = new Person( Jane Doe , Melbourne , 5551234 ); // get name from object jane and print it System.out.println(jane.getName()); } }
Exercise Create a Person class Attributes (instance variables) name, height (inches), salary (annual salary) Methods constructor , getName, getSalary, getHeightInInches getHeightInFeet getHeightInMeters getHeightInFeetAndInches // return an int array with feet and inches Create a PersonTest class Jane Doe, 61 inches, $55000 Mike Smith, 71 inches, $44000 Print Jane s and Mike s hourly rate--52 weeks, 40 hours/week Print Jane s height in feet, meters, and feet and inches. Same for Mike // return inches in int // return feet in double // return meters in double
Abstraction (Information Hiding) The Person class Hides the internal attributes private Provides external access via its methods public
Hiding Some Info about Height Look closer at height What information are we hiding?
Hiding some info about Height Look closer at height What information are we hiding? how height is stored different methods to get height
Hiding some info about Height Look closer at height What information are we hiding? how height is stored different methods to get height What can be changed in Person without changing PersonTest? Imagine: Person is written by a team in Florida PersonTest is written by a team in California Changes by the Florida team don t affect the team in California
Hiding some info about Height Look closer at height What information are we hiding? how height is stored different methods to get height What can be changed in Person without changing PersonTest? Imagine: Person is written by a team in Florida PersonTest is written by a team in California Changes by the Florida team don t affect the team in California Attribute _height could be in feet and inches instead of inches Changes in Person [by the Florida team] But No changes in PersonTest! [by the California team]
Hiding Height Better The constructor seems to imply the height must be stored in inches How can we improve this?
Hiding Height Better The constructor seems to imply the height must be stored in inches How can we improve this? Multiple constructors
class Person public class Person { private String _name; // attributes (instance variables) private int _height;// inches private int _salary;// annual salary public Person(String name, int heightInches, int salary)//constructor { } public Person(String name, int heightFeet, int heightInches, int salary) { } }
Abstraction (Information Hiding) Which constructor is more natural for external use? Person(String name, int heightInches, int salary) Person(String name, int heightFeet, int heightInches, int salary) 1. 2.
Abstraction (Information Hiding) Which constructor is more natural for external use? Person(String name, int heightInches, int salary) Person(String name, int heightFeet, int heightInches, int salary) 1. 2. Which internal representation of attribute height uses less memory? int height // in inches int heightFeet, heightInches
Abstraction (Information Hiding) Which constructor is more natural for external use? Person(String name, int heightInches, int salary) Person(String name, int heightFeet, int heightInches, int salary) 1. 2. Which internal representation of attribute height uses less memory? int height // in inches int heightFeet, heightInches Abstraction (information hiding) allows: a more natural interface for external use and a more memory-efficient internal representation
Wait a minute Two constructors with the same name Can we do that?
Wait a minute Two constructors with the same name Can we do that? Yes, if the parameter types are different overloading same name, different behavior Applies to methods as well
Overloadingsame name, different sequence of parameter types Given Person(String name, int heightInches, int salary) Person(String name, int heightFeet, int heightInches, int salary) Can we also have Person(String name, int heightInches, int heightFeet, int salary) Person(String name, int salary, int heightFeet, int heightInches) Person(int heightFeet, int heightInches, String name, int salary) 1. 2. 3.
Exercise class Person Add a constructor that allows feet and inches class PersonTest Create a third Person object Michael Jordan, 6 feet, 6 inches, $1,000,000 Print his hourly rate, height in feet, meters, and feet and inches
this object public int getHeightInInches() { return _height; // return this._height; } ------PersonTest--- jane.getHeightInInches();
Parameter is an object of the same class Consider a method that compares two Person objects Whether one is taller than another In procedural thinking, we would want isTaller(jane, mike) In OO thinking, we think object first jane.isTaller(mike) // yep, it looks ugly, but closer to English
this object public boolean isTaller(Person other) { // _height refers to jane s height // other._height refers to mike s height return _height > other._height; // return this._height > other._height; } -------PersonTest--------- jane.isTaller(mike)
A Second Class Non-simple problems usually involve objects from different classes. Let s say we want to see how many minutes Michael Jordan need to work to buy an iPhone. What would be another class in this problem? What are the attributes? What are the methods?
Exercise class Phone Attributes: model, price constructor, getPrice class PersonTest Create a Phone object: iPhone 5c 16 GB unlocked , $549 Create another Phone object: iPhone 5s 16GB unlocked , $649 Print how many minutes Michael Jordan needs to work to buy each of the iPhones
A Note on Checking Invalid Input You probably have learned about checking for invalid input Same with methods, particularly with constructor Invalid values for attributes cause problems later
Checking Invalid Parameter Values public Person(String name, int height, int salary) { // if name is null, attribute _name should be what? // if height is negative, attribute _height should be what? // if salary is negative, attribute _salary should be what? }
Checking Invalid Parameter Values public Person(String name, int height, int salary) { if (name != null) _name = name; else _name = Unknown ; if (height > 0) _height = height; else _height = 0; if (_salary > 0) _salary = salary; else _salary = 0; }
Remember we have another constructor Person(String name, int heightFeet, int heightInches, int salary) We want to check for invalid parameter values too Repeating instructions? How to not repeat instructions?
Remember we have another constructor Person(String name, int heightFeet, int heightInches, int salary) We want to check for invalid parameter values Repeating instructions! How to not repeat instructions? Abstract the checking into a method
set method with checking public Person(String name, int height, int salary) { set(name, height, salary); } public Person(String name, int heightFeet, int heightInches, int salary) { set(name, heightFeet * 12 + heightInches, salary); } private void set(String name, int height, int salary) // public if you allow changes externally { if (name != null) _name = name; else _name = Unknown ; if (height > 0) _height = height; else _height = 0; if (_salary > 0) _salary = salary; else _salary = 0; }