Coding Challenges and Object-Oriented Design Concepts
This content covers coding challenges related to determining divisibility of numbers, checking equality of rows in a 2D array, and a multiple-choice question on object-oriented program design. It also includes an AP-style question testing ArrayList operations. Additionally, it provides insights on how to structure classes for storing information about cars in a dealership.
Download Presentation
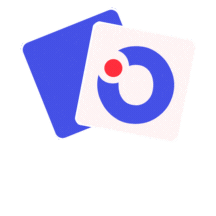
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
A number is considered divisible if every digit of that number divides into the number evenly. For example, 128 is divisible. 128 will divide evenly by 8, 2, and 1. If the number has a 0, then this number is automatically not divisible since dividing by 0 is an error. Create a static method called isdivisible that will take an integer as a parameter and return true if the number is divisible, or return false if the number is not divisible.
Create a static method called isEqual that takes a 2D array(X x Y) as its parameter. This method will determine if any of the rows of the 2D array are equal. Take a look 0 5 8 3 1 4 7 6 5 9 8 9 8 7 9 5 6 1 7 3 = = = = 20 26 39 26 Row 1 and Row 3 are equal The method should return true if any rows are equal and should return false if any rows are not equal
public static boolean isEqual(array2[x][y]) { int[] sumarray = new int[x]; for(int k = 0; k < x; k++) sumarray[k] = 0; for(int i = 0; i < x; i++) for(int j = 0; j < y; j++) sumarray[x] = sumarray[x] + array[i][j]; for(int k = 0; k < x; k++) for(int j = 0; j < x; j++) if(sumarray[k] == sumarray[j]) if(j != k) return true return false; }
Sample Multiple-Choice Question A car dealership needs a program to store information about the cars for sale. For each car, they want to keep track of the following information: number of doors (2 or 4), whether the car has air-conditioning, and its average number of miles per gallon. Which of the following is the best object-oriented program design? (a) Use one class, Car, with three instance variables: int numDoors, boolean hasAir, and milesPerGallon. (b) Use four unrelated classes: Car, Doors, AirConditioning, and MilesPerGallon. (c) Use a class Car with three subclasses: Doors, AirConditioning, and MilesPerGallon. (d) Use a class Car, with a subclass Doors, with a subclass AirConditioning, with a subclass MilesPerGallon. (e) Use three classes: Doors, AirConditioning, and MilesPerGallon, each with a subclass Car.
AP Questions (1) What is the output of the following code? ArrayList<String> animals = new ArrayList<String>(); animals.add( dog ); animals.add( cat ); animals.add( bird ); animals.set(2, lizard ); animals.add(1, fish ); animals.remove(4); System.out.println(animals); (A) [dog, fish, cat] (B) [dog, fish, lizard] (C) [dog, lizard, fish] (D) [fish, dog, cat] (E) The code throws an ArrayOutofBoundsException exception.
Create a class called Students. This class is to contain three private data: an integer to hold student age, an integer to hold student ID, and a String to hold their name. There should be a method that asks you to input the values for a student. There should also be a method that outputs student data in the following manner. Name: ID: Age: John Doe 15421 17 In your main execution, start declaring an array list of this class. Give me four options: 1. Add on student 2. Delete a student 3. Display the entire student list 4. Exit program If I choose to delete a student, your program should give me an option as to which student I want to delete. The program should keep running until I choose Exit program option