Lecture 4 Dynamic Programming
In dynamic programming, large problems are broken down into smaller sub-problems to optimize solutions. This approach involves memorizing sub-problem results to avoid redundant computations. The concept is illustrated using examples like computing Fibonacci numbers efficiently. Learn about the principles and steps involved in dynamic programming algorithms with practical strategies for problem-solving.
Download Presentation
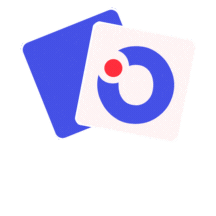
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Basic Algorithm Design Techniques Divide and conquer Dynamic Programming Greedy Common Theme: To solve a large, complicated problem, break it into many smaller sub-problems.
Dynamic Programming Idea: Break the problem into many closely related sub-problems, memorize the result of the sub- problems to avoid repeated computation.
Warmup Example: Fibonacci Numbers f(n) = f(n-1) + f(n-2), f(1) = f(2) = 1
Nave solution Follow the recursion directly f(7) f(6) f(5) f(4) f(3) f(5) f(4) f(3) f(4)
Nave solution Follow the recursion directly f(7) f(6) f(5) f(4) f(5) f(3) f(4) f(3) f(4)
Memoization f(n) 1. IF n < 3 THEN RETURN 1. 2. IF f(n) has been computed before THEN RETURN stored result. 3. res = f(n-1) + f(n-2) 4. Mark f(n) as computed, Store f(n) = res 5. RETURN res n 1 2 3 4 5 6 7 8 f(n) 1 1 2 3 5 8 13 21 Dynamic Programming Table
Filling in the table iteratively Observation: f(n) is always computed in the order of n = 1, 2, 3, No need to recurse f(n) 1. IF n < 3 THEN RETURN 1. 2. a[1] = a[2] = 1 3. FOR i = 3 TO n 4. a[i] = a[i-1] + a[i-2] 5. RETURN a[n]
Basic Steps in Designing Dynamic Programming Algorithms Relate the problem recursively to smaller sub- problems. (Transition function, f(n) = f(n-1)+f(n-2)) Organize all sub-problems as a dynamic programming table. (A table for all values of n) Fill in values in the table in an appropriate order. (n = 1, 2, 3, )
Example 1: Longest Increasing Subsequence Input: Array of numbers a[] = {4, 2, 5, 3, 9, 7, 8, 10, 6} Subsequence: list of numbers appearing in the same order, but may not be consecutive Example: {4, 2, 5}, {4, 3, 8}, {2, 5, 7, 8, 10} Subsequence b[] is increasing if b[i+1] > b[i] for all i. Output: Length of the longest increasing subsequence. Example: 5, the subsequence is {2, 5, 7, 8, 10} or {2, 3, 7, 8, 10} (both have length 5)
Example 2 Knapsack Problem There is a knapsack that can hold items of total weight at most W. There are now n items with weights w1,w2, , wn. Each item also has a value v1,v2, ,vn. Goal: Select some items to put into knapsack 1. Total weight is at most W. 2. Total value is as large as possible.