Introduction to Sequences, Variables, Strings, and Lists
Learn about creating variables, working with strings, understanding lists, and performing various operations on them. Explore different data types and ways to store and manipulate data in Python. Discover how to handle scenarios where the number of elements is unknown in advance through practical examples and images.
Download Presentation
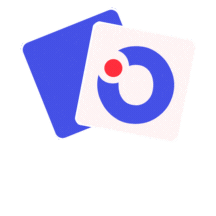
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Module 5 Module 5 Part 1 Part 1 Sequence Types
Motivation Motivation Problem: store five integer numbers. Solution: create five variables! CREATE number1 = 10 CREATE number2 = 20 CREATE number3 = 30 CREATE number4 = 40 CREATE number5 = 50 PRINT("number1: + number1) PRINT("number2: + number2) PRINT("number3: + number3) PRINT("number4: + number4) PRINT("number5: + number5) What if we don t know ahead of time how many we need?
Topics Topics Strings Lists and their Properties Creating lists and using lists Manually iterating through lists Tuples Ranges
Strings Strings Sequence type which we ve been using so far Is a collection of 0 or more characters Characters are ordered (meaning one comes before the other) The characters may not be sorted (e.g.: there could be a z before an a in a string) We use [ ] to access elements inside Start with an index of 0 Technically immutable name = Alice print(name[0]) # prints A
Lists Lists Sequence type Is a collection of 0 or more elements Elements in a list can be of any type These elements are ordered (meaning one comes before the other) The elements may not be sorted (may not be arranged from smallest to largest) We use [ ] to create them and access elements inside Start with an index of 0 Variable size
Lists Lists Operations we can perform with lists: Creating a list Retrieving an element from a list Retrieving multiple elements from a list Adding an element to a list Deleting an element from a list Checking if a list has a particular element Sorting a list
Lists Lists Creating Creating # this creates an empty list names = [] # this list has been initialized with some values states = [ Georgia , Florida , Alabama ] # this list has been initialized using an iterable object numbers = list(range(100)) # this list is initialized using a list comprehension us_states = [state for state in states]
Lists Lists Accessing Accessing # this prints Florida # lists always start at 0 print(states[1]) #Slicing. More on this in a bit print(states[0:1]) # checks if e is in Alice , returning True of False print( e in Alice ) # checks if z is not in Alice , returning True of False Print( z not in Alice )
Lists Lists Adding elements Adding elements # Adds Alice to the end of the list names.append( Alice ) # inserts Charlie at the 0thposition # shifts all elements starting at 0 back one space names.insert(0, Charlie ) names.append( Bob ) # will print [ Charlie , Alice , Bob ] print(names) # replaces the item at position 1 with David names[1] = David
Lists Lists Removing elements Removing elements # will remove Charlie from the list del names[0] # returns and removes the element at position 0 name = names.pop(0) # will empty the list of elements names.clear() # will remove the elements from position 0 up to # but not including the element in position 2 del states[0:2] # will remove the first occurrence of Eve in names # will crash, because the list has no Eve names.remove( Eve )
Lists Lists Slicing Slicing The previous slides used something called slicing This feature is not usually available in other languages It allows the retrieval of a slice of a sequence type [start : end] [start : end : step] end is not inclusive name = Alice # this will print lic print(name[1:4])
Lists Lists Slicing (Continued) Slicing (Continued) Most Sequence types in python implement slicing Notice that the type of the slice usually depends on the type of the original sequence i.e.: slicing a string gives you a string, slicing a list gives you a list states = [ Georgia , Florida , Alabama ] # this will print [ Florida , Alabama ] print(states[1:3])
Lists Lists Useful Methods Useful Methods len(list) # returns the number of elements min(list) # returns the smallest element max(list) # returns the largest element list.count(e) # total number of occurrences of element e in the list list.index(e) # returns the index of the first occurrence of e list.reverse() # reverses the list. Doesn t return anything list.sort() # sorts the list from smallest to largest. Doesn t return anything
Lists Lists Manual Iteration Manual Iteration What if you want to perform different actions to elements on a list depending on what the element is? We can simply use a for loop! Remember that a for loop can iterate through any iterable All sequence types are iterable e.g.: states = [ Georgia , Florida , Alabama , Delaware ] for state in states: if e in state: print(state + contains the letter e! )
Lists Lists Manual Iteration Manual Iteration Notice that reassigning the variable used to iterate through the for loop will not replace the element in the original list states = [ Georgia , Florida , Alabama , Delaware ] for state in states: if e in state: print(state + contains the letter e! ) state = This state had the letter e print(states) # still contains the original states
Lists Lists Manual Iteration Manual Iteration Instead, we need to replace the element at the specific position where it is The enumerate() method can be used here to give us the element as well as where it is currently being stored states = [ Georgia , Florida , Alabama , Delaware ] for position, state in enumerate(states): if e in state: print(state + contains the letter e! ) states[position] = This state had the letter e print(states) # states have been replaced
Lists Lists Manual Sorting Manual Sorting While we can always call the sort() method to sort a list, what if we wanted to sort a list manually? After all, when we call sort(), the computer is running some code. What could that code look like? In practice, you ll hardly ever be asked to write code to sort something manually Not all sorting algorithms are created equal Some use more space Some run slower Some are more complex It s still a good idea to know basics
Lists Lists Manual Sorting Manual Sorting Bubble Sort: the most basic sorting algorithm Algorithm: Compare two elements If they are out of order, swap them Keep doing this until you reach the end of your collection Once you do, the last element is guaranteed to be the last one! Do this again to find the second-to-last element, then the third-to-last, etc
Lists Lists Bubble sort Bubble sort list_of_numbers = [43,58,65,38,38,4,57,36,6,45] list_length = len(list_of_numbers) for iteration in range(list_length): for position in range(list_length iteration - 1): if list_of_numbers[position] > list_of_numbers[position + 1]: temp = list_of_numbers[position] list_of_numbers[position] = list_of_numbers[position + 1] list_of_numbers[position + 1] = temp print(list_of_numbers)
Lists Lists Lists inside Lists Lists inside Lists It is possible to put a list inside another list! This is sometimes done to simulate a 2-dimensional list In this case, the outer list is keeping track of the rolls while the inner lists are keeping track of the columns The list below has 3 elements: 3 empty lists names = [[], [], []] Notice that accessing the element at position 0 gives us the first list. print(names[0]) # prints an empty list []
Lists Lists Lists inside Lists Lists inside Lists If we want to append an element to the first list inside the names list, we need to access the element in the first index and call its append() names below is now [[ Alice ],[],[]] names[0].append( Alice ) What will names look like after the operations below? names[0].append( Amanda ) names[0].append( Ana ) names[1].append( Bob ) names[1].append( Bilford ) names[2].append( Charlie ) names[2].append( Chance ) names[2].append( Cameron ) names[2].append( Corbin )
Lists Lists Lists inside Lists Lists inside Lists If we want to access an element inside of an element, we just need to add an extra pair of square brackets. names = [[ Alice ],[],[]] print(names[0]) # prints Alice print(names[0][0]) # prints A names.append( Bob ) # append Bob to outer list # append Charlie to element in position 0 names[0].append( Charlie ) print(names) # the print above will print the following: [[ Alice , Charlie ],[],[], Bob ]
Lists Lists Lists inside Lists Lists inside Lists Be mindful of what element is accessed in a sequence! Recall that each data type has a different set of operations that can be performed on them. names = [[ Alice ],[],[]] # appends Bob to the outer list names.append( Bob ) # appends Charlie to the first inner list names[0].append( Charlie ) # This is an error # We are trying to call append() on a string names[0][0].append( David )
Tuples Tuples Is very similar to a list, with the biggest difference being that tuples are immutable (i.e.: once you create one, you cannot add any more elements to it) Tuples are much like lists, but with () instead of [] names = () states = ( Georgia , Florida , Alabama ) numbers = tuple(range(100)) # unlike with lists, the line below WILL NOT generate a tuple us_states = (state for state in states)
Tuples Tuples # accessing a particular index in a tuple still uses [] print(states[0]) # prints Georgia print(states[1:3]) # prints ( Florida , Alabama ) Since tuples are immutable, none of the insertion and deletion methods are available. However, we can concatenate two tuples much like we do with strings: states = ("Georgia", "Florida", "Alabama") more_states = ("South Carolina", "North Carolina", "Delaware") states += more_states print(states) # all states are now in a single tuple
Tuples Tuples - - Sorting Sorting How can we sort the elements in a tuple if a tuple is immutable? Tuples do not have the tuple.sort() method, as it would require tuples to be mutable. We can instead use the built-in sorted() method, which takes in any iterable and returns a sorted list of it. If you still need a tuple, you can simply convert it back. # states from previous slide, with 6 states states = tuple(sorted(states))
Ranges Ranges Similar to a tuple (no insertion or deletion allowed) Does not allow for concatenation either Generally used as the iterator in a for loop Main advantage over lists and tuples is the small amount of memory used Ranges don t actually store any values, but instead generate them on the fly With a single input, range() iterates from 0 up to (input 1) With 2 inputs, range() iterates from the left number to (right number 1) With 3 inputs, range() iterates from the left number to (middle number 1) skipping (right number). The right number is usually called the step
Summary Summary Sequence types can any number of elements of different types Elements in a Sequence are always ordered, but may not be sorted Lists can vary in size once created Strings, Tuples and Ranges cannot vary in size once created You can access one or more elements in a Sequence You can put other sequences inside your sequences